As a beginner React developer, the task of maintaining React applications can be daunting. With so many best practices, tools, and technologies to keep track of, it can be difficult to know where to start.
But let me tell you, maintaining React applications is crucial for keeping the application running smoothly and helps you improve your skills and become a more proficient developer.
With this guide, you’ll learn the best practices, tools, and techniques that will help you to maintain React applications effectively. You’ll learn how to write clean and organized code, how to use version control software and collaborate with other developers, how to document your code, how to test and debug efficiently, how to understand the overall architecture and design of the application.
By following these guidelines, you’ll be able to elevate your skills, improve your coding practices, and become a valuable asset to any software agency. Don’t miss out on this opportunity to take your skills to the next level. A great tool to improve your code is to use a tool like CopyCat that generates any Figma design to code instantly. You’ll be able to analyze code without putting the effort into generating it in the first place. Easily spot improvements and improve your coding practices exponentially.
Best Practices for Writing Clean and Organized Code
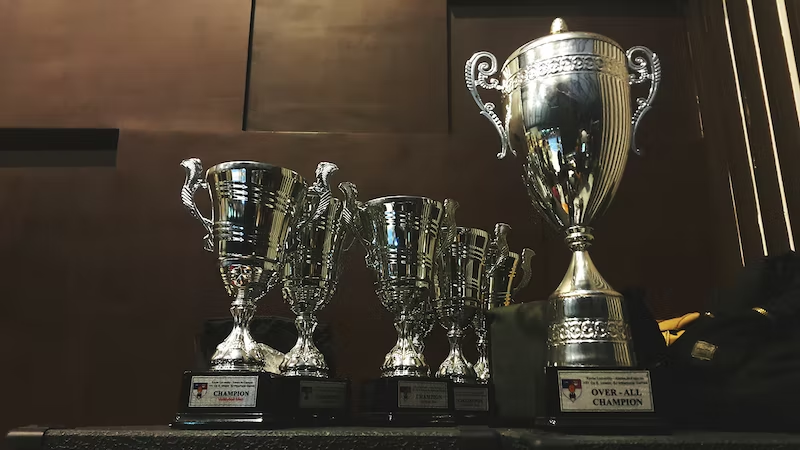
One of the most important things that a beginner React developer can do to maintain an application is to write clean and organized code. Clean code is easy to read, understand, and modify. Organized code is easy to navigate and understand the overall structure of the application.
There are five specific best practices for writing good code:
1. Use Descriptive Variable Names
Use meaningful and descriptive variable names that clearly indicate the purpose and usage of the variable. This will make it easier for other developers to understand the code and navigate the application.
// Bad
let x = fetch("<https://jsonplaceholder.typicode.com/todos/1>")
// Good
let todoData = fetch("<https://jsonplaceholder.typicode.com/todos/1>")
In the first example, the variable name x
needs to clearly indicate its purpose, making it difficult to understand what the variable contains. In the second example, the variable name todoData
clearly indicates its purpose, making it easier to understand what the variable contains.
Another example could be:
//Bad
let a = db.query("SELECT * FROM users")
// Good
let usersData = db.query("SELECT * FROM users")
In the first example, the variable name a
needs to clearly indicate the purpose of the variable, and it makes it difficult to understand what the variable contains. In the second example, the variable name usersData
clearly indicates its purpose, making it easier to understand what the variable contains. You get the idea!
It’s important to use descriptive variable names, not only for the sake of readability but also to make it easy for any other developer to understand the code. Using meaningful names will make the code self-explanatory, making it easier to understand and maintain the codebase.
It’s important to remember that variable names should be clear, concise, and self-explanatory.
2. Break Up Code into Smaller, Reusable Functions
Break up the code into smaller, reusable functions that perform specific tasks. This makes the code more organized, easier to read and understand, and more maintainable in the long run.
Example:
class CheckoutPage extends React.Component {
// Bad
calculateDiscount(price, discount) {
const tax = 0.2;
const finalPrice = price - (price * discount);
const finalPriceWithTax = finalPrice + (finalPrice * tax);
return finalPriceWithTax;
}
handleCheckout(items) {
let total = 0;
items.forEach((item) => {
total += this.calculateDiscount(item.price, item.discount);
});
console.log("Total: " + total);
}
render() {
return (
<div>
<button onClick={() => this.handleCheckout(this.props.items)}>Checkout</button>
</div>
)
}
}
// Good
class CheckoutPage extends React.Component {
calculateDiscount(price, discount) {
return price - (price * discount);
}
calculateTax(price) {
const tax = 0.2;
return price + (price * tax);
}
handleCheckout(items) {
let total = 0;
items.forEach((item) => {
const discountedPrice = this.calculateDiscount(item.price, item.discount);
const finalPriceWithTax = this.calculateTax(discountedPrice);
total += finalPriceWithTax;
});
console.log("Total: " + total);
}
render() {
return (
<div>
<button onClick={() => this.handleCheckout(this.props.items)}>Checkout</button>
In the first example, the function calculateDiscount
is performing two actions, calculation of discount and calculation of tax. This makes it difficult to understand the flow of the code, and in case if we want to change the tax calculation, we have to go through the entire function.
In the second example, the function calculateDiscount
is performing only one action, calculation of discount. The function calculateTax
is performing only one action, calculation of tax. This makes it much easier to understand the flow of the code, and if we want to change the tax calculation, we can simply go to the calculateTax
function and make the change there. Also, these smaller, reusable functions can be used in other parts of the application, making the code more modular and maintainable.
Additionally, by breaking down the code into smaller, reusable functions, it’s easier to test and debug each function individually. This makes it easier to identify and fix bugs, and also makes it easier to add new features to the application.
In summary, breaking up the code into smaller, reusable functions makes it more organized, easier to read and understand, and more maintainable in the long run.
3. Follow a Consistent Code Style
Follow a consistent code style throughout the codebase. This ensures that the code is easy to read and understand and makes it easier for other developers to navigate the application.
Example:
// Bad
class ShoppingCart extends React.Component{
render(){
return (
<div>
<h2>Your Cart</h2>
{this.props.items.map((item, index)=>
<div key={index}>
{item.name} - {item.price}
</div>
)}
</div>
);
}
}
// Good
class ShoppingCart extends React.Component {
render() {
return (
<div>
<h2>Your Cart</h2>
{this.props.items.map((item, index) => (
<div key={index}>
{item.name} - {item.price}
</div>
))}
</div>
);
}
}
In the first example, the indentation, braces and parenthesis are not consistent, and this makes it difficult to read and understand the code. In the second example, the indentation, braces, and parenthesis are consistent, and this makes it easier to read and understand the code.
By following a consistent code style, it ensures that the code is easy to read and understand, and makes it easier for other developers to navigate the application. It also helps to maintain the codebase, as it’s easy to spot any inconsistencies in the code. Consistency in code style also makes it easier to work in a team, as it ensures that everyone is using the same conventions and that the codebase is easy to read and understand for everyone.
4. Create Modular and Reusable Components
Create modular and reusable components that can be used in different parts of the application. This makes the code more maintainable by breaking down the application into smaller, reusable parts.
Example:
// Bad
class ItemList extends React.Component {
render() {
return (
<div>
{this.props.items.map((item, index) => (
<div key={index}>
<h2>{item.name}</h2>
<p>{item.description}</p>
<p>Price: {item.price}</p>
<button onClick={() => this.props.addToCart(item)}>Add to Cart</button>
</div>
))}
</div>
);
}
}
// Good
class Item extends React.Component {
render() {
return (
<div>
<h2>{this.props.name}</h2>
<p>{this.props.description}</p>
<p>Price: {this.props.price}</p>
</div>
);
}
}
class AddToCartButton extends React.Component {
render() {
return <button onClick={() => this.props.addToCart(this.props.item)}>Add to Cart</button>
The first example shows a component that is tightly coupled and does multiple things, rendering the list of items, displaying item details and handling the “add to cart” functionality. This makes it difficult to reuse and maintain. The second example shows a separation of concerns, where the code is broken down into smaller, reusable components: Item
and AddToCartButton
. This separation makes it more modular and reusable, easier to maintain, and improves the application’s overall performance.
5. Follow the Principle of Single Responsibility
Each component should be responsible for a specific task, this will make it easier to understand the flow of the application and maintain the code.
Example:
// Bad
function Item(props) {
const handleClick = () => {
// do something
};
return (
<div>
<h2>{props.name}</h2>
<p>{props.description}</p>
<p>Price: {props.price}</p>
<button onClick={handleClick}>Add to Cart</button>
</div>
);
}
// Good
function Item(props) {
return (
<div>
<h2>{props.name}</h2>
<p>{props.description}</p>
<p>Price: {props.price}</p>
</div>
);
}
function AddToCartButton(props) {
const handleClick = () => {
// do something
};
return <button onClick={handleClick}>Add to Cart</button>;
}
This example illustrates the principle of single responsibility, by separating the “add to cart” functionality into a separate component, AddToCartButton
. The Item
component is responsible for displaying the item name, description, and price, while the AddToCartButton
component is responsible for handling the “add to cart” functionality. This separation of concerns makes the components more modular and reusable. The Item
component can be reused in other parts of the application, such as in a product detail page, while the AddToCartButton
component can be reused in other parts of the application where an “add to cart” functionality is required. This makes it easy to maintain and improve the application and also makes it easy to reuse the codebase.
Watch this video from an ex-Googler about their three tips to writer cleaner code:
Version Control and Collaboration
- Use a version control system such as Git to keep track of changes to the codebase and collaborate with other team members.
- Create clear and descriptive commit messages to make it easy for other team members to understand the changes made.
- Use branches to work on different features or bug fixes in parallel, and merge changes back into the main branch when they are ready.
- Use pull requests to review and merge code changes, and make sure that code changes are reviewed by at least one other team member before they are merged.
Example:
Let’s say a team is working on a feature that includes updating the layout of a specific page in the application. The team leader could create a new branch called “feature/update-layout” for this feature. Team members can work on this branch and make commits to it.
When a team member finishes working on a specific task related to this feature, they can create a pull request to merge their changes into the “feature/update-layout” branch. The team leader or another team member will review the changes before merging them into the branch. This way, the team can work together on the feature in parallel, and ensure that changes are reviewed before they are merged into the main codebase.
By using version control and collaboration, it makes it easy to track changes to the codebase and collaborate with other team members. It also makes it easy to roll back changes if necessary, and makes it easy to see who made changes to the codebase and when.
Documenting Your Code
Include Clear Explanations of How the Code Works
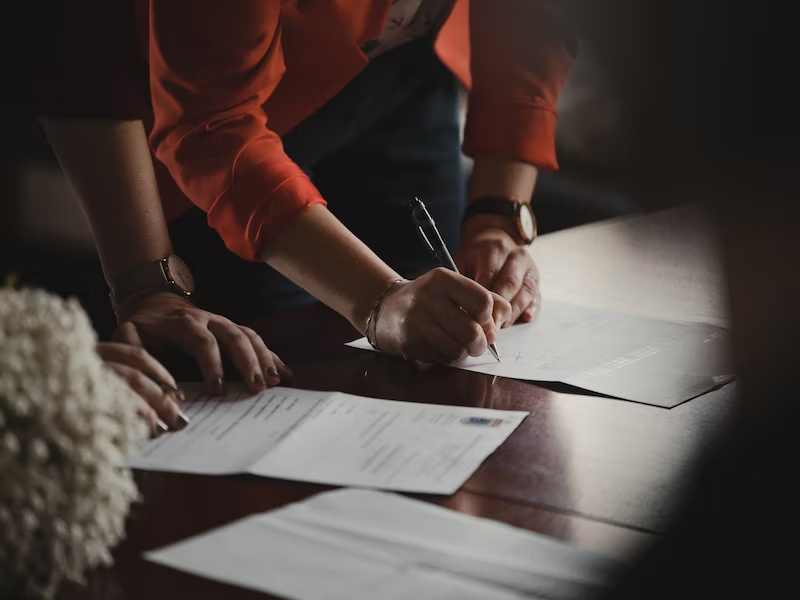
Including clear explanations of how the code works is essential for ensuring that other developers can understand how the application works. This includes providing information on the inputs, outputs, and any edge cases that the code handles. It also includes providing information on the algorithm used and any data structures used.
Example:
// This function takes in an array of numbers and returns the sum of all the numbers
function sumNumbers(numbers) {
let total = 0;
// Using a for loop to iterate through the array
for (let i = 0; i < numbers.length; i++) {
total += numbers[i];
}
return total;
}
Describe the Purpose of Each Function or Component
Describing the purpose of each function or component ensures that developers understand the role that each part of the code plays in the application. This includes providing information on what the function or component does and how it is used in the application.
// The Item component displays information about an item on the products page
function Item(props) {
return (
<div>
<h2>{props.name}</h2>
<p>{props.description}</p>
<p>Price: {props.price}</p>
</div>
);
}
By following these best practices for documenting your code, it ensures that other developers can understand the decisions and trade-offs that were made when building the application
Testing and Debugging
Testing and debugging are essential for maintaining React applications. Regular testing ensures that the application is running optimally and that any bugs or issues are caught early. To test and debug React applications effectively, beginner React developers should:
A. Write Unit Tests
Writing unit tests is an important step in testing React applications. Unit tests allow developers to test individual components or functions in isolation, and ensure that they work as expected. This allows developers to catch bugs early in the development process and make it easier to fix them.
Example:
import { sumNumbers } from './math-functions';
test('sumNumbers should return the sum of an array of numbers', () => {
expect(sumNumbers([1, 2, 3])).toBe(6);
});
B. Use a Debugging Tool
Using a debugging tool is an important step in debugging React applications. A debugging tool allows developers to step through the code and understand how the application works. This allows developers to catch bugs and fix them.
Example: In Google Chrome browser, you can use the developer tool to debug your react application. The developer tool allows you to see the component tree, inspect elements, and see the state and props of the component.
C. Monitor Application Performance
Monitoring application performance is an important step in maintaining React applications. It allows developers to see how the application is performing, and identify areas of improvement. This includes measuring the time it takes for the application to load, and monitoring the memory usage.
Example: In Google Chrome browser, you can use the developer tool to monitor the performance of your react application. The developer tool allows you to see the time it takes for the application to load, and monitor the memory usage.
By following these best practices for testing and debugging, it allows developers to catch and fix bugs and ensure that the application works as expected, and also allows for performance monitoring and optimization.
Understanding the Overall Architecture and Design
Understanding a React application’s overall architecture and design is essential for maintaining it effectively. It allows developers to understand how the different parts of the codebase work together and how the application is structured. To understand the overall architecture and design of a React application, beginner React developers should:
- Review the codebase
- Ask questions to other team members
- Understand the design principles and patterns used in the application
- Create flow charts for your understand for future references
Reviewing the codebase allows developers to understand how the different parts of the codebase work together. Asking questions to other team members allows developers to gain a deeper understanding of the application. Understanding the design principles and patterns used in the application ensures that developers understand how the application is structured and how it works.
Here’s a quick video about understanding different React architectures:
Conclusion
Maintaining React applications can be challenging for beginner developers, but by following best practices for writing clean and organized code, using version control and collaboration, staying up-to-date with React and related technologies, documenting your code, testing and debugging, understanding the overall architecture and design, and communicating effectively, beginner React developers can effectively maintain and improve React applications. Remember to commit often, use descriptive variable names, break up code into smaller, reusable functions and follow a consistent code style, use version control software like Git, stay informed about updates and changes to React, document your code, test and debug frequently and effectively, understand the architecture and design of the application, and communicate clearly and actively listen to other team members. By following these guidelines, beginner React developers can elevate their skills and become more proficient in maintaining React applications.