- The Pitfalls of Not Understanding typeof Javascript
- Understanding the Basics of JavaScript typeof Operator
- Tips for using typeof Javascript effectively in your code
- Common Pitfalls with JavaScript typeof
- Comparing JavaScript typeof to Other Type Checking Methods
- With great power comes great responsibility (and great debugging skills)!
You’ve likely encountered the typeof operator in your code. Whether you’re checking variable types or manipulating expressions, typeof is an essential tool for any developer working with JavaScript. However, despite its ubiquity, the typeof operator can be challenging to understand fully. In this article, we’ll explore the basics of typeof and dive into some advanced techniques for mastering its usage. But before we get into that, let’s begin with a quick anecdote about the importance of understanding typeof in practice.
The Pitfalls of Not Understanding typeof Javascript
Imagine you’re working on a JavaScript project that requires you to parse and manipulate data from a REST API. You’ve written a script that retrieves the data and are ready to start processing it. However, when you run your code, you encounter an error: “TypeError: Cannot read property ‘length’ of undefined.” After some investigation, you realize that the error is caused by a null variable instead of an array, causing your code to fail. This scenario exemplifies the pitfalls of needing to understand typeof and the importance of using it correctly in your code. In the following sections, we’ll explore the basics of typeof and provide some tips and best practices for using it effectively in your JavaScript projects.
Understanding the Basics of JavaScript typeof Operator
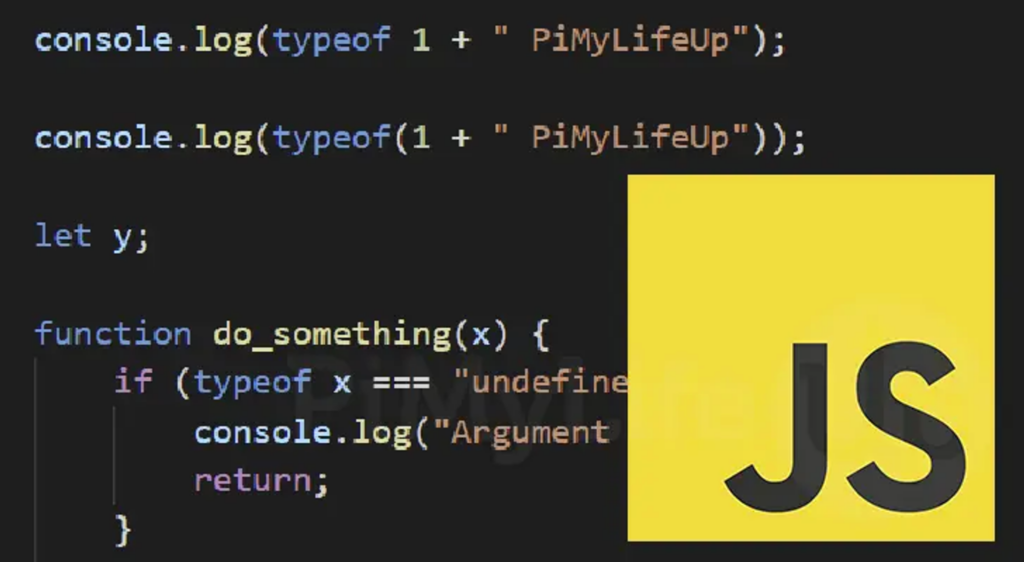
What is typeof in javascript?
The typeof Javascript operator is a built-in operator in JavaScript that returns a string indicating the type of a variable or expression. It can be used to check whether a variable is a number, string, boolean, object, or function. The typeof Javascript operator takes a single operand, which can be either a variable, an expression, or a literal value. So, does typeof return a string JavaScript? Yes, it does.
How to check type of data in JavaScript?
To use typeof, you simply pass a variable or expression as an argument. For example, if you have a variable called “age” that contains a number, you can use typeof Javascript to check its type like this:
const age = 27;
console.log(typeof age); // output: "number"
Similarly, if you have a function called “sayHello” that logs a greeting to the console, you can use typeof to check its type like this:
function sayHello() {
console.log("Hello, world!");
}
console.log(typeof sayHello); // output: "function"
Understanding the output of typeof Javascript with different data types (number, string, object, etc.)
When you use typeof, it returns a string representing the type of the variable or expression you passed in. The following table shows the different types of values that typeof Javascript can return and some examples, including javascript typeof array and javascript typeof function, of how to use it:
Type | Example code | typeof output |
---|---|---|
Number | const age = 27; | “number” |
String | const name = "John"; | “string” |
Boolean | const isStudent = true; | “boolean” |
Undefined | let x; | “undefined” |
Null | const y = null; | “object” |
Object | const person = { name: "John", age: 27 }; | “object” |
Array | const numbers = [1, 2, 3]; | “object” |
Function | function sayHello() { console.log("Hello, world!"); } | “function” |
It’s worth noting that typeof returns “object” for both objects and arrays. To determine whether a variable is an object or an array, you can use the Array.isArray() method. Brush up your learning with a 4-minute overview of typeof:
Tips for using typeof Javascript effectively in your code
Here are some tips for using typeof effectively in your JavaScript code:
Always use parentheses with typeof
Although typeof Javascript works without parentheses, it’s always best to use them for consistency and readability. For example, console.log(typeof age);
is better than console.log(typeof age);
.
Understand the limitations of typeof
As mentioned earlier, typeof returns “object” for both objects and arrays, so you may need to use other methods (like Array.isArray() or instanceof) to determine the actual type. Additionally, typeof doesn’t distinguish between different types of objects (like Date objects, RegExp objects, etc.).
Use typeof in combination with other operators or methods
To perform more complex type checking, you can combine typeof with other operators or methods. For example, to check if a variable is an array, you can use Array.isArray()
in conjunction with typeof like this:
const numbers = [1, 2, 3];
if (typeof numbers === 'object' && Array.isArray(numbers)) {
console.log('numbers is an array');
}
Or, to check if a variable is a Date object, you can use the instanceof operator like this:
const today = new Date();
if (today instanceof Date) {
console.log('today is a Date object');
}
Use typeof for input validation
One common use case for typeof is to validate user input. For example, if you’re building a form that requires a user to enter a number, you can use typeof to check whether the input is valid like this:
const ageInput = document.getElementById('age-input');
const ageValue = Number(ageInput.value);
if (typeof ageValue === 'number' && !isNaN(ageValue)) {
console.log('ageInput is a valid number');
} else {
console.log('ageInput is not a valid number');
}
In this example, typeof is used to check whether the input is a number, while isNaN()
is used to check whether the input is a valid number.
Use typeof to handle optional function arguments
When you define a function with optional arguments, you can use typeof to check whether the argument was provided or not. For example, if you have a function that calculates the area of a rectangle and accepts an optional third argument for units, you can use typeof to check whether the units were provided like this:
function calculateArea(width, height, units) {
let area = width * height;
if (typeof units !== 'undefined') {
area += ' ' + units;
}
return area;
}
In this example, the typeof
operator is used to check whether the units
argument was provided. If it was not provided, the typeof
operator will return 'undefined'
, and the if
statement will not execute. If the units
argument was provided, the typeof
operator will return 'string'
, and the if
statement will add the units to the calculated area.
Using typeof
in this way can help make your code more flexible and robust, allowing users to optionally provide arguments and avoiding errors caused by missing arguments.
It’s important to note that typeof
cannot distinguish between an argument that was not provided and an argument that was explicitly set to undefined
. To handle this scenario, you can use a combination of typeof
and the logical OR (||
) operator like this:
function calculateArea(width, height, units) {
let area = width * height;
units = units || 'square units';
if (typeof units === 'string') {
area += ' ' + units;
}
return area;
}
In this example, the ||
operator is used to set the default value for units
if it was not provided or was explicitly set to undefined
. Then, the typeof
operator is used to check whether units
is a string before adding it to the calculated area.
Overall, using typeof
to handle optional function arguments can help make your code more flexible and avoid errors caused by missing arguments. However, it’s important to be aware of its limitations and use it in combination with other techniques to handle edge cases.
Common Pitfalls with JavaScript typeof
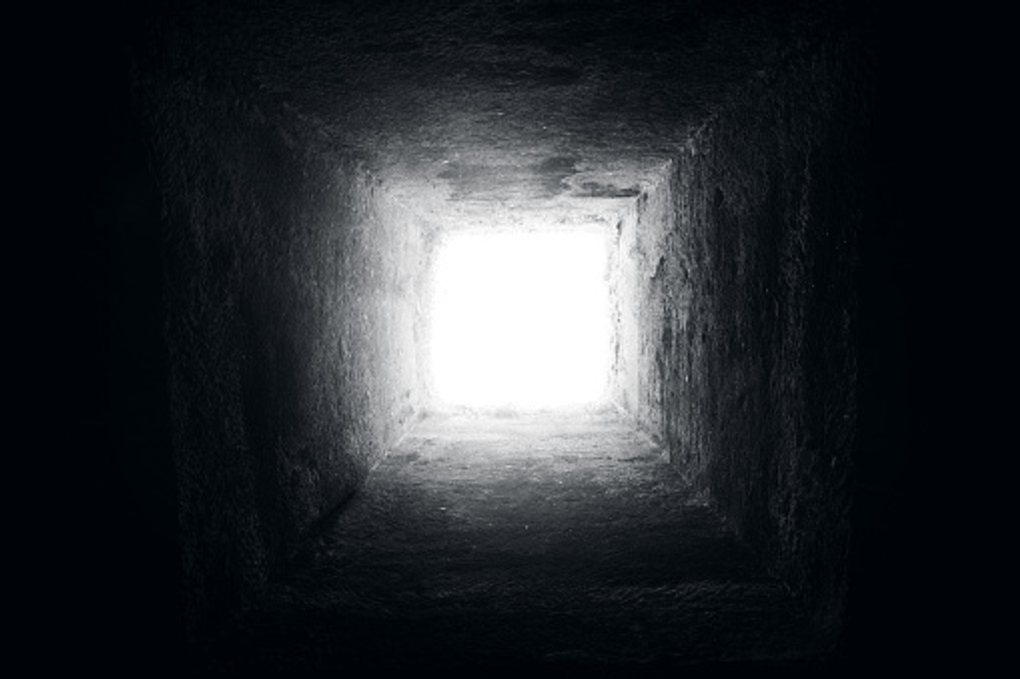
JavaScript typeof is a powerful operator, but there are some common pitfalls that developers should be aware of. In this section, we’ll explore some of these pitfalls and how to avoid them. But before that, let’s discuss misleading results with typeof.
Misleading Results
In some cases, typeof Javascript may return results that are not what you expect, leading to errors or unexpected behavior in your code. Some scenarios where typeof may not behave as expected include:
- Javascript typeof null returns “object”: This is a known bug in JavaScript that has existed since the early days of the language. When you use typeof on a null value, it will return “object” instead of “null”. To check for null values, you can use the strict equality operator (===) instead.
- typeof NaN returns “number”: NaN (Not a Number) is a special value in JavaScript that represents an invalid number. However, when you use typeof on NaN, it returns “number”. This can be confusing if you are using typeof to check for actual numbers. To check for NaN, you can use the isNaN() function instead.
- Javascript typeof array and objects: When you use typeof on an array or an object, it will return “object”. This is because arrays and objects are both considered to be objects in JavaScript. To check whether a variable is an array, you can use Array.isArray() instead.
Typeof is just one of several type checking methods available in JavaScript, and it’s often a good idea to use other methods in conjunction with typeof to ensure accurate type checking.
Type Coercion
One common pitfall with typeof is type coercion, which can lead to unexpected results when checking the type of a value. For example:
typeof NaN; // returns "number"
typeof null; // returns "object"
typeof []; // returns "object"
typeof {}; // returns "object"
In each of these cases, the typeof operator returns a value that may not be what you expect. To avoid this pitfall, it’s important to use strict equality (===) when comparing types, and to be aware of the limitations of typeof.
Checking for Objects
Another common pitfall with typeof is when checking for objects. The typeof operator will return “object” for any value that is an object, including arrays, functions, and null. For example:
typeof []; // returns "object"
typeof function() {}; // returns "function"
typeof null; // returns "object"
To avoid this pitfall, it’s important to use other techniques, such as the instanceof operator or Object.prototype.toString, to check whether a value is a specific type of object.
Edge Cases
There are some edge cases where typeof may not behave as expected. For example, typeof returns “function” for functions, but it may also return “object” if the function is created using certain techniques, such as object constructors. Similarly, typeof returns “undefined” for variables that are not defined, but it may also return “undefined” for variables that are explicitly set to undefined.
To avoid these edge cases, it’s important to be aware of how typeof works and to test your code thoroughly to ensure that it behaves as expected in all scenarios.
Variable Scoping
Another pitfall to be aware of when using typeof is variable scoping. If you use typeof to check the type of a variable that has not been declared, you will get a ReferenceError. For example:
typeof x; // throws ReferenceError: x is not defined
To avoid this pitfall, always declare your variables before using them in your code.
Type Checking vs Value Checking
Finally, it’s important to understand the difference between type checking and value checking when using typeof. typeof is designed to check the type of a value, not its actual value. For example:
typeof true; // returns "boolean"
typeof 1; // returns "number"
In both of these cases, typeof returns the type of the value, not the actual value itself. To check the actual value of a variable, you will need to use other comparison operators, such as == or ===.
By being aware of these common pitfalls and taking steps to avoid them, you can use typeof effectively and confidently in your JavaScript code.
Comparing JavaScript typeof to Other Type Checking Methods
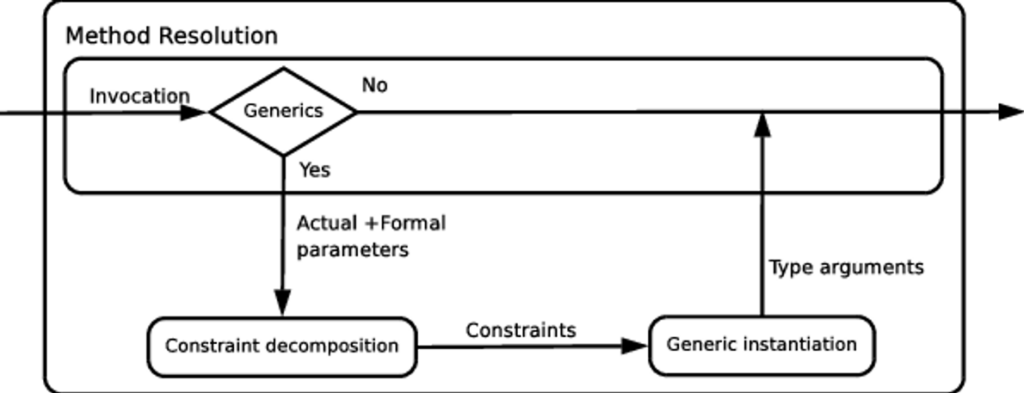
In addition to typeof, there are other methods for checking the type of a variable in JavaScript. The most common alternatives are the instanceof operator and Object.prototype.toString. Here’s a comparison of the three methods:
typeof
As we’ve seen, typeof is a built-in operator in JavaScript that returns a string representation of the type of a variable. Some of the strengths of using typeof for type checking include:
- Simplicity: typeof is a simple and straightforward way to check the type of a variable.
- Speed: typeof is a fast operation and has minimal impact on performance.
- Built-in: typeof is a built-in operator in JavaScript, so it doesn’t require any external libraries or dependencies.
However, there are also some limitations to using typeof, such as:
- Limited functionality: typeof is limited to checking basic data types like numbers, strings, and objects. It may not work as expected for more complex types like dates, regular expressions, or custom objects.
- Potential for misunderstandings: As we discussed in the previous section, there are some common misunderstandings or mistakes that can occur when using typeof.
instanceof
The instanceof operator is another method for checking the type of an object in JavaScript. It works by checking whether an object is an instance of a particular constructor. Here are some of the strengths and weaknesses of using instanceof:
- Strengths:
- More precise: instanceof can be more precise than typeof, as it checks whether an object is an instance of a specific constructor.
- Works with custom objects: instanceof can be used to check whether an object is an instance of a custom constructor function.
- Weaknesses:
- Limited functionality: instanceof is still limited to checking whether an object is an instance of a particular constructor. It may not work as expected for other types of objects.
- May not work across frames: instanceof may not work across different frames in a browser.
Object.prototype.toString
Object.prototype.toString is a method that returns a string representation of the object’s constructor. It can be used to check the type of an object by calling Object.prototype.toString.call(obj). Here are some of the strengths and weaknesses of using Object.prototype.toString:
- Strengths:
- Works with all data types: Object.prototype.toString can be used to check the type of any data type in JavaScript, including custom objects.
- More precise: Like instanceof, Object.prototype.toString can be more precise than typeof, as it checks the object’s constructor rather than just its type.
- Weaknesses:
- More complex: Object.prototype.toString is a more complex method than typeof or instanceof and requires more code to use.
- May be slower: Object.prototype.toString may be slower than typeof or instanceof due to its complexity.
Here’s a table comparing all three:
Method | Usage | Strengths | Weaknesses |
---|---|---|---|
typeof | Checking for basic types such as string, number, object, undefined, and function | Easy to use, returns a string indicating the type of the operand | May return “object” for null and functions, doesn’t work for more complex objects like arrays and dates |
instanceof | Checking if an object is an instance of a specific constructor function | Works with custom classes, allows for inheritance checking | Only works with objects created with a constructor function, doesn’t work with primitive types |
Object.prototype.toString | Returns a string representation of an object’s type | Works with all objects, allows for checking specific built-in types | Can be slower than typeof , requires calling the method on the object directly using call() or apply() |
Recommendations for When to Use Each Method
So, when should you use typeof, instanceof, or Object.prototype.toString for type checking in JavaScript? Here are some general recommendations:
- Use typeof for checking basic data types like numbers, strings, and objects.
- Use instanceof for checking whether an object is an instance of a particular constructor, especially for custom objects.
- Use Object.prototype.toString to check the type of an object when typeof and instanceof are insufficient, or when you need more precise information about the object’s constructor.
Ultimately, the best method for type checking in JavaScript will depend on your project’s specific needs and the variables you need to check.
Find out from this video about the easiest type checking method:
With great power comes great responsibility (and great debugging skills)!
By mastering the basics and advanced techniques of typeof
Javascript, you can achieve more complex functionality and avoid common pitfalls. You can also compare typeof
with other type checking methods, such as instanceof
and Object.prototype.toString
, to make informed decisions about which method to use in different scenarios.
So, if you want to write efficient and effective JavaScript code, it’s crucial to learn and practice using typeof
. With the tips, best practices, and examples we’ve covered, you’ll be well on your way to becoming a confident and skilled JavaScript developer. And who knows, you may even find yourself enjoying the art of debugging!