- Introduction
- TypeScript forEach Definition and Usage
- How Does TypeScript forEach Work?
- Examples of TypeScript forEach Loop
- Using TypeScript forEach Loop With Set and Map Data Types
- When to Use TypeScript forEach Loop?
- TypeScript forEach() vs map() Operator: Which is Faster?
- Other Array Iteration Functions
- Conclusion
- Interesting Reads from Our Blog
Introduction
The forEach loop is one of the ways to iterate between the elements of an array. Array manipulation is one of the techniques developers use daily. Rendering data from an API call usually requires iteration between each element.
In this article, you’ll learn how TypeScript forEach loop works and when to use it. You’ll also see some examples of TypeScript forEach function. Before we get started, here is a brief intro to TypeScript.
TypeScript is a superset to JavaScript. It adds type-checking to your programs. And this helps developers minimize errors in their code. If you’re still new to TypeScript, the video below should get you up to speed.
With that out of the way, let’s get started with learning how forEach loop works in TypeScript.
TypeScript forEach Definition and Usage
The forEach loop is a JavaScript function that is used to iterate between array elements. If you have a list of items and want to perform operations on each array element, you can use the forEach loop. In TypeScript, the forEach loop has the same syntax as in JavaScript.
Basic Usage
Below is the basic syntax of the forEach loop in TypeScript.
array.forEach(callback[, thisObject])
Callback function: This is the function that operates on each array element.
thisObject: Used to reference the current state of the array element.
Note: There is no return value in the forEach loop. The function alters the original array by performing operation on each array element.
The callback function accepts three optional arguments: item, index, and array.
- item: The item is the individual elements of the array
- index: This is the position of each element present in the array.
- array: The array argument references the array you are iterating.
How Does TypeScript forEach Work?
You can use the forEach loop to iterate between the elements of an array, set, map, or list in TypeScript.
We call the forEach method to iterate between the elements of an array. This function takes a callback function. You can perform operations on each array element within the callback function. Here is an example.
const myList = [1,2,3,4,5]
myList.forEach(item => {
console.log(item)
})
In the code above, we have an array of numbers, myList. We then loop through it using the forEach function. We parse the individual array item within the callback function as an argument. Then log each item on the console. You should get the following output when you run the code snippet above.
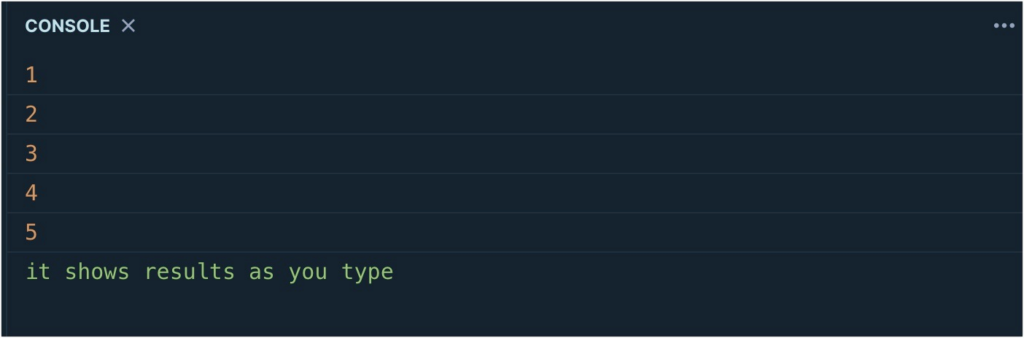
Examples of TypeScript forEach Loop
In this section, we’ll run through some examples of the forEach loop in TypeScript.
Requirements
To follow along with this guide, you’ll need to have npm installed on your machine. You also need to install TypeScript on your system. If you don’t have TypeScript installed, then run the npm command below.
npm install typescript
If you’d like to make TypeScript available globally, then use the code below to install it.
npm install -g typescript
Basic forEach Loop in TypeScript
With the forEach() function, you can copy elements from one array into a new empty array.
const oldArr:string[] = ["item 1", "item 2", "item 3"];
const newArr:string[] = [];
oldArr.forEach(item => {
newArr.push(item)
})
console.log(newArr);
When you run the code above, you’ll see a copy of the oldArr as the values for the newArr in the console.
Note: If you are using the forEach method to copy an array to a new variable in TypeScript, both variables need to be of the same type. Otherwise, you’d get a type error.
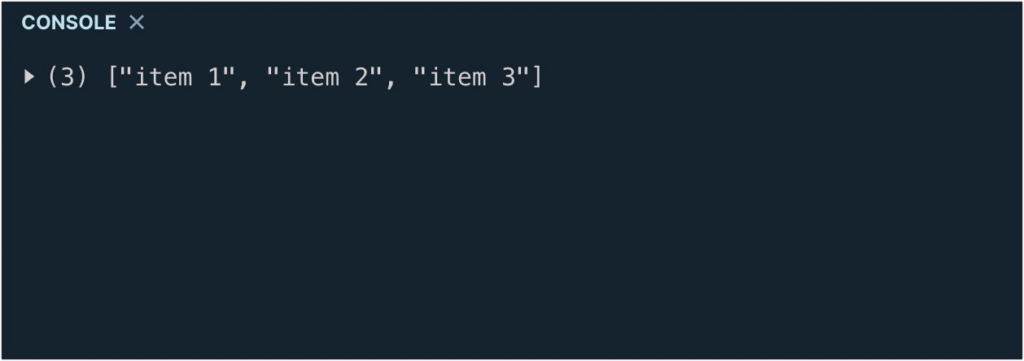
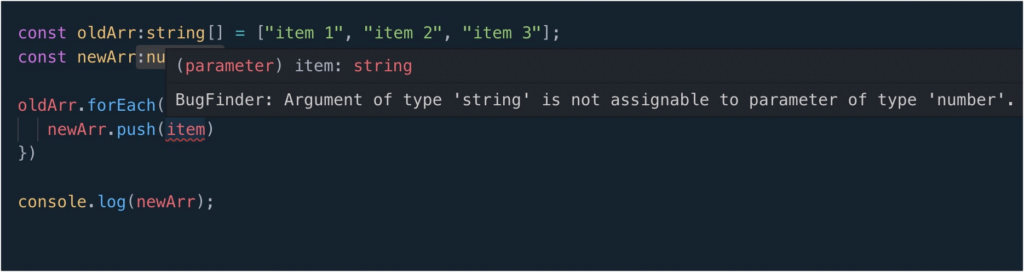
We can also access the index of an array by parsing the index argument into the callback function.
const arr:string[] = ['David', 'Samuel', 'Joan', 'Claire']
arr.forEach((name, index) => {
console.log(`Welcome ${name}, your index is ${index}`)
})
When you run the code, you should get the following names logged on the console and their index.
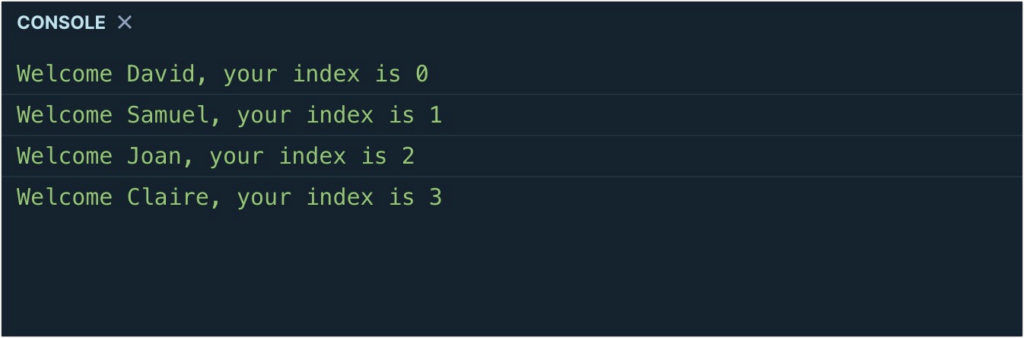
The forEach() method can also reference the array as a third argument. This means you can parse three arguments as parameters in the callback function. Let’s modify the code above to include the array parameter.
const arr:string[] = ['David', 'Samuel', 'Joan', 'Claire']
arr.forEach((name, index, array) => {
console.log(`Welcome ${name}, your index is ${index}`)
console.log(array)
})
The array parameter references the current state of the array object before the iteration. When you execute the code snippet above, it should display the array object on the console.
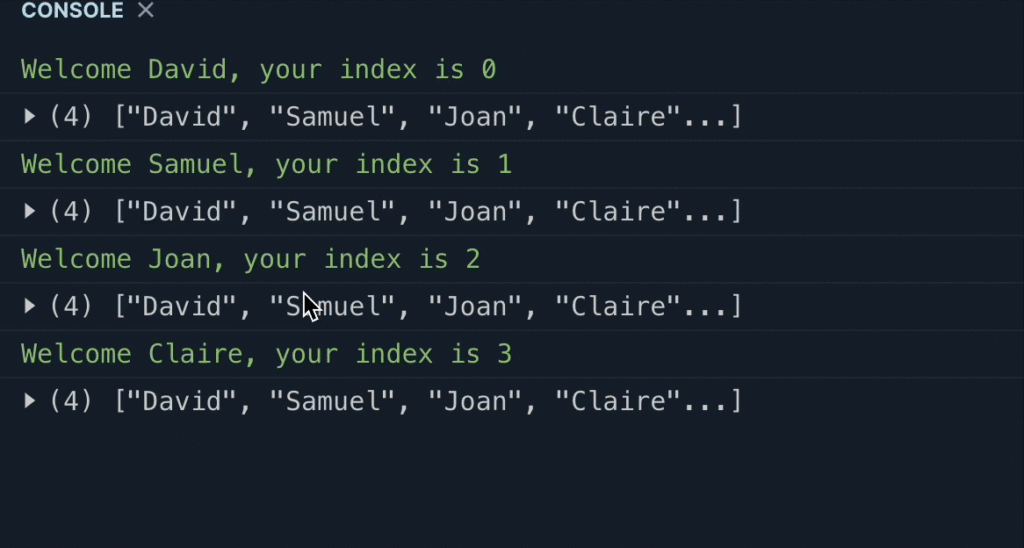
Using TypeScript forEach Loop With Set and Map Data Types
Before ES2015, you could only iterate arrays using the forEach loop. In ES2015, you can integrate forEach loop into the Map and Set data types. It also accepts three arguments: value, key, and DataType.
value: This is the value of the data you are looping through.
key: When using Map(), it references the key that is mapped to each value.
DataType: This is the data type for which the forEach loop is being called. It can be the Set() or Map() data type.
Set()
Let’s declare a new set and iterate over the elements in the set.
let evenNumbers = new Set<number>([2, 4, 6, 8, 10]);
evenNumbers.forEach((value, key) => {
console.log('the even numbers are', value, 'with key', key);
});
In the code above, we declare a new set of type number. It contains even numbers from 2-10. We can use the forEach method the same way as in an array to access individual elements of the set. When you execute the code above, you should get the following lines logged in the console.
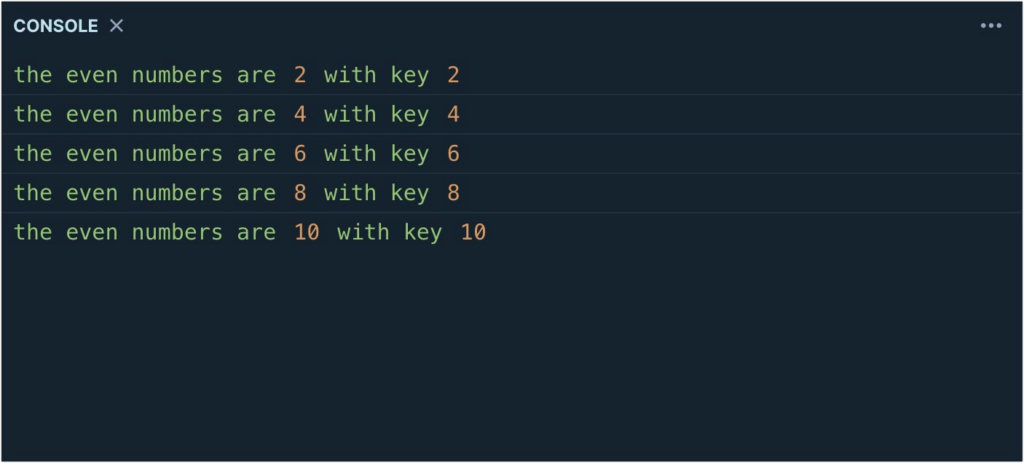
Note: In a Set, the value and key are the same.
Map()
The Map data type allows you to store key-value pairs. Similarly, you can iterate between the data in a Map.
let userName = new Map<string, string>([
["firstName", "David"],
["lastName", "Ozokoye"]
]);
userName.forEach((value, key) => {
console.log(`${key} is ${name}`)
})
In the code above, we create a Map to collect the user’s first name and last name. We can use the forEach function to display the key and the value of this Map.
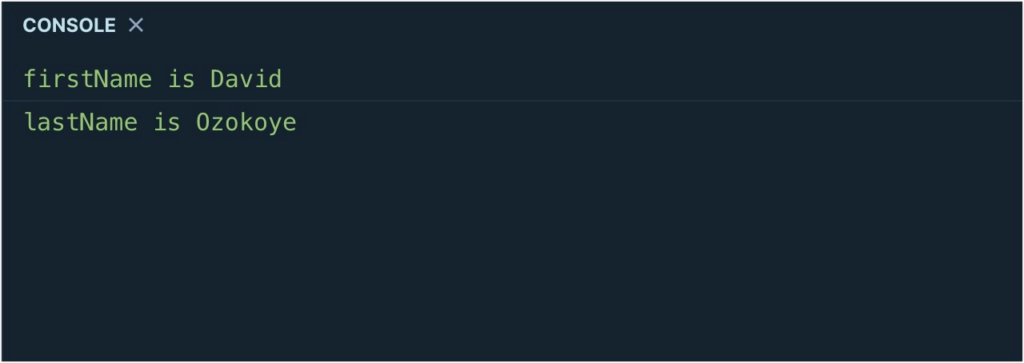
When to Use TypeScript forEach Loop?
There are other ways to iterate between elements of a list or an array, such as the map(), filter(), or reduce() function. However, forEach loop is preferred if you are iterating between array elements and wish to perform operations on each array element.
Additionally, if you want to modify the original array, then you should use the forEach loop in TypeScript. The forEach loop also doesn’t have a return value. Instead, it modifies the original array and updates its values with the new value.
You can integrate TypeScript into your React app. If you need to loop over a JSON response on your app, the forEach loop in TypeScript can be a handy combination.
Looking to build React UI faster? Our tool at CopyCat easily converts your Figma designs into functional React components. This would save you time and help you focus on building functionalities such as using the forEach loop to render data from an API.
TypeScript forEach() vs map() Operator: Which is Faster?
The forEach loop and the map operator are similar in that both can be used to iterate between an array. However, the main difference between them is that forEach doesn’t have a return value. The map() operator returns a new array by operating on each array element.
Also, because the map operator returns a new array, you can chain the return values and perform operations on each array element. In contrast, the forEach loop is not chainable since its return value is undefined.
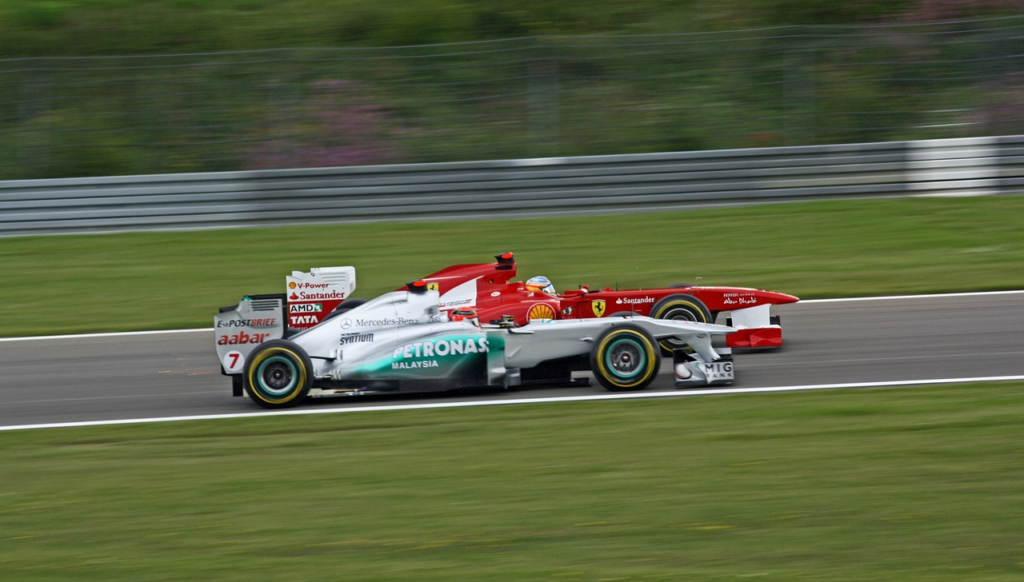
So which is faster or better? Well, it boils down to the use case. If you want to iterate over array elements and do not need a return value, then the forEach function is ideal.
However, if you need to operate on individual array elements while maintaining the original array, you should use the map function.
Other Array Iteration Functions
Aside from the forEach function, JavaScript and TypeScript have other array manipulation methods. Below are some of the most popular ones.
- map function
- filter function
- reduce function
- some function
- every function
- find function
If you’d like to learn how each method executes array iteration, check out this video tutorial from FreeCodeCamp.
Conclusion
That’s it! You now know how to use the forEach loop in TypeScript. We also explained the difference between the forEach loop and the map operator.
If you’d like to learn more about TypeScript, be sure to check our tutorial on the TypeScript Typeof operator.
Our tool at CopyCat helps developers to build User Interfaces faster. With our low-code React builder, you can create production-level code from your Figma designs.
Interesting Reads from Our Blog
Below are some links to other relevant tutorials about TypeScript.