- What are Javascript Operators?
- JavaScript Operators Cheatsheet
- Javascript Arithmetic Operators
- Javascript Comparison Operators
- Javascript Conditional Operators
- Javascript Assignment Operators
- = (Simple Assignment)
- += (Addition Assignment Operator)
- = (Subtraction Assignment Operator)
- = (Multiplication Assignment Operator)
- /= (Division Assignment Operator)
- %= (Modulus Assignment Operator)
- <<= (Left Shift Assignment Operator)
- >>= (Right Shift Assignment Operator)
- &= (Bitwise AND Assignment Operator)
- |= (Bitwise OR assignment)
- ^= (Bitwise XOR assignment)
- Javascript Bitwise Operators
- Use This CheatSheet and Save Time
Working with JavaScript operators is a daily task. With so many operators available, it can be overwhelming and time-consuming to remember what each one does.
Consider a scenario where you are building a web application that requires a user to input their date of birth. You need to verify that the user is at least 18 years old before they can proceed to use your app. To achieve this, you can use the greater than operator (>) to compare the current date with the user’s date of birth. You can then use the logical AND operator (&&) to check if the difference between the current date and the user’s date of birth is greater than or equal to 18 years. Then, you can use an if statement to either allow or deny access to the app based on the result of the comparison.
With this simple example, it’s easy to see how a JavaScript operators cheatsheet can save time and improve the efficiency of your code.
What are Javascript Operators?
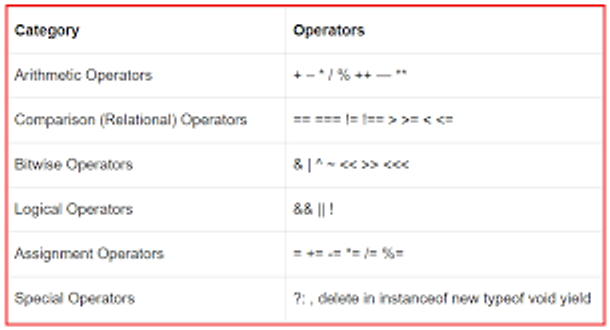
JavaScript operators are symbols or keywords that perform operations on one or more values (operands) and return a result. JavaScript provides various operators, including arithmetic, comparison, logical, bitwise, and more. These operators allow you to manipulate data, perform calculations, compare values, and control program flow.
When JavaScript encounters an expression containing multiple operators and operands, it uses a set of rules called operator precedence to determine the order of evaluation. Operator precedence defines the order in which JavaScript evaluates the operators and operands in an expression. This can be important because it can affect the result of an expression. For example, the expression 3 + 4 * 2
would evaluate to 11, not 14, because the multiplication operator (*) has a higher precedence than the addition operator (+). To override the default operator precedence, you can use parentheses to group the operators and operands in an expression in the order you want them to be evaluated.
What are the 4 types of JavaScript operators?
There are different types of JavaScript operators, including:
- Arithmetic operators: These operators are used to perform mathematical operations on numerical values.
- Comparison operators: These operators are used to compare two values and return a boolean value (true or false) based on the comparison result.
- Logical operators: These operators are used to combine multiple conditions and return a boolean value based on the result.
- Bitwise operators: These operators are used to manipulate the binary representation of numeric values.
Additionally, JavaScript has assignment operators, which assign values to variables. Some common assignment operators include =, +=, -=, *=, /=, %=, <<=, >>=, &=, |=, and ^=. Assignment operators combine the operation with the assignment, such as x += y, the same as x = x + y. There are conditional operators, also known as ternary operators, that allow you to perform an operation based on a condition.
How many operators are there in JavaScript?
There are 44 operators in JavaScript, which can be classified into arithmetic, comparison, logical, bitwise, assignment, and conditional operators. These operators perform various operations on values and variables in JavaScript, such as addition, subtraction, comparison, and logical operations. It’s essential to understand these operators well to write efficient and effective code in JavaScript.
Here’s a crash course on all you need to know about Javascript operators:
JavaScript Operators Cheatsheet
One of the most challenging aspects of working with JavaScript operators is keeping track of each type’s syntax, symbols, and rules. To help make your coding experience more efficient, we’ve created JavaScript operators cheat sheet that you can download and use as a reference.
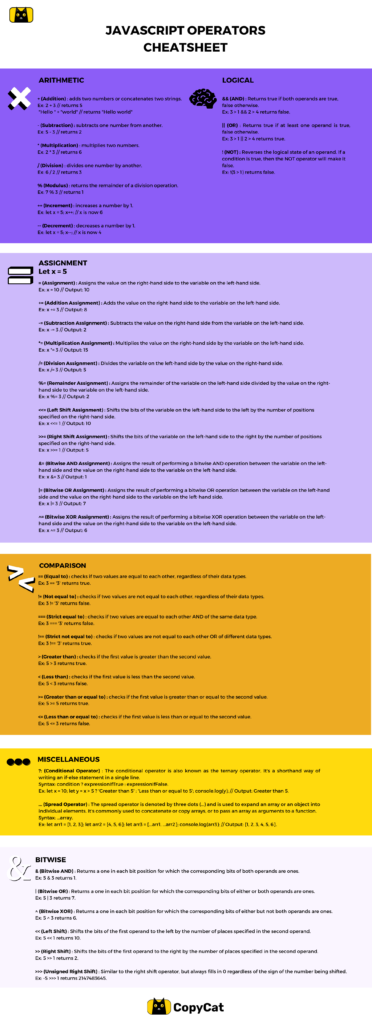
The cheatsheet provides an at-a-glance overview of all the different types of JavaScript operators, including arithmetic, comparison, logical, conditional, assignment, and bitwise operators. Here’s a breakdown of each type:
Javascript Arithmetic Operators
Arithmetic operators allow you to perform mathematical calculations in your code. This includes addition, subtraction, multiplication, and division, as well as more advanced calculations such as exponentiation and modulus. The JavaScript arithmetic operators include +, -, *, /, %, ++, and –.
Operator | Description |
---|---|
+ | Adds two values or concatenates two strings |
– | Subtracts one value from another |
* | Multiplies two values |
/ | Divides one value by another |
% | Returns the remainder of one value divided by another |
++ | Increments a value by 1 |
— | Decrements a value by 1 |
+ (Addition)
The addition operator (+) is used to add two or more values together. When used with numeric values, it returns the sum of the values. When used with strings, it concatenates the values together.
Example:
let a = 2;
let b = 3;
let sum = a + b; // sum = 5
let name = "John";
let greeting = "Hello";
let message = greeting + " " + name; // message = "Hello John"
– (Subtraction)
The subtraction operator (-) is used to subtract one value from another. It returns the difference between the values.
Example:
let a = 5;
let b = 2;
let difference = a - b; // difference = 3
* (Multiplication)
The multiplication operator (*) is used to multiply two or more values together. It returns the product of the values.
Example:
let a = 3;
let b = 4;
let product = a * b; // product = 12
/ (Division)
The division operator (/) is used to divide one value by another. It returns the quotient of the values.
Example:
let a = 6;
let b = 3;
let quotient = a / b; // quotient = 2
% (Modulus)
The modulus operator (%) is used to find the remainder when one value is divided by another. It returns the remainder of the division operation.
Example:
let a = 7;
let b = 3;
let remainder = a % b; // remainder = 1
++ (Increment)
What does ++ mean in JavaScript? The increment operator (++) is used to add 1 to a variable. It can be used either as a prefix (++a) or a postfix (a++) operator. When used as a prefix, it increments the variable before it is used in an expression. When used as a postfix, it increments the variable after it is used in an expression.
Example:
let a = 5;
a++; // a = 6
let b = ++a; // b = 7, a = 7
— (Decrement)
The decrement operator (–) is used to subtract 1 from a variable. It can be used either as a prefix (–a) or a postfix (a–) operator. When used as a prefix, it decrements the variable before it is used in an expression. When used as a postfix, it decrements the variable after it is used in an expression.
Example:
let a = 5;
a--; // a = 4
let b = --a; // b = 3, a = 3
Javascript Comparison Operators
Comparison operators allow you to compare two values and return a boolean result. This includes checking for equality, inequality, greater than, less than, and more. The JavaScript comparison operators include ==, !=, ===, !==, >, <, >=, and <=.
Operator | Description |
---|---|
== | Compares two values for equality, converting data types if necessary |
!= | Compares two values for inequality, converting data types if necessary |
=== | Compares two values for equality without type coercion |
!== | Compares two values for inequality without type coercion |
> | Checks if the value on the left is greater than the value on the right |
< | Checks if the value on the left is less than the value on the right |
>= | Checks if the value on the left is greater than or equal to the value on the right |
<= | Checks if the value on the left is less than or equal to the value on the right |
== (Equality)
The equality operator (==) compares two values for equality. It returns true if the values are equal, and false if they are not. If the two values being compared are of different types, JavaScript will attempt to convert one or both of the values to a common type before making the comparison.
Example:
console.log(2 == 2); // true
console.log("2" == 2); // true
console.log(2 == "3"); // false
console.log(null == undefined); // true
console.log(true == 1); // true
!= (Inequality)
The inequality operator (!=) is the opposite of the equality operator. It returns true if the values being compared are not equal, and false if they are equal.
Example:
console.log(2 != 3); // true
console.log("2" != 2); // false
console.log(2 != "3"); // true
console.log(null != undefined); // false
console.log(true != 1); // false
=== (Strict Equality)
The strict equality operator (===) compares two values for equality, but unlike the equality operator, it does not perform type coercion. This means that if the types of the two values are different, the operator will always return false.
Example:
console.log(2 === 2); // true
console.log("2" === 2); // false
console.log(2 === "2"); // false
console.log(null === undefined); // false
console.log(true === 1); // false
!== (Strict Inequality)
The strict inequality operator (!==) is the opposite of the strict equality operator. It returns true if the values being compared are not equal, and false if they are equal. It also does not perform type coercion.
Example:
console.log(2 !== 3); // true
console.log("2" !== 2); // true
console.log(2 !== "2"); // true
console.log(null !== undefined); // true
console.log(true !== 1); // true
> (Greater Than)
The greater than operator (>) compares two values and returns true if the first value is greater than the second value.
Example:
console.log(2 > 1); // true
console.log("2" > 10); // false
console.log(2 > "3"); // false
< (Less Than)
The less than operator (<) compares two values and returns true if the first value is less than the second value.
Example:
console.log(2 < 10); // true
console.log("2" < 1); // false
console.log(2 < "3"); // true
>= (Greater Than or Equal To)
The greater than or equal to operator (>=) compares two values and returns true if the first value is greater than or equal to the second value.
Example:
console.log(2 >= 2); // true
console.log("2" >= 10); // false
console.log(2 >= "3"); // false
<= (Less Than or Equal To)
The less than or equal to operator (<=) compares two values and returns true if the first value is less than or equal to the second value.
Example:
console.log(2 <= 2); // true
console.log("2" <= 1); // false
console.log(2 <= "3"); // true
Javascript Logical Operators
Logical operators allow you to create logical statements in your code that evaluate to true or false. This includes AND (&&), OR (||), and NOT (!) operators. Logical operators are commonly used to evaluate multiple conditions and control program flow.
Operator | Description |
---|---|
&& | Logical AND operator, returns true if both operands are true |
! | Logical NOT operator, returns the opposite boolean value of the operand |
&& (Logical AND)
The logical AND operator (&&) returns true if both operands are true, and false otherwise. It is often used in conditional statements to check if multiple conditions are true.
Example:
console.log(true && true); // true
console.log(true && false); // false
console.log(false && false); // false
console.log(2 > 1 && "hello" !== "world"); // true
console.log(2 > 3 && "hello" !== "world"); // false
|| (Logical OR)
The logical OR operator (||) returns true if at least one of the operands is true, and false otherwise. Like the logical AND operator, it is often used in conditional statements.
Example:
console.log(true || true); // true
console.log(true || false); // true
console.log(false || false); // false
console.log(2 > 1 || "hello" !== "world"); // true
console.log(2 > 3 || "hello" === "world"); // false
! (Logical NOT)
The logical NOT operator (!) is a unary operator that returns the opposite of the boolean value of its operand. If the operand is true, it returns false. If the operand is false, it returns true.
Example:
console.log(!true); // false
console.log(!false); // true
console.log(!(2 > 1)); // false
console.log(!("hello" === "world")); // true
There are great ways you can use logical operators to help in complex situations. Learn all about it here:
Javascript Conditional Operators
Conditional operators, also known as ternary operators, are a type of operator that is used to evaluate a condition and return one of two values based on the result of the evaluation.
The conditional operator consists of three operands: a condition followed by a question mark (?), then an expression to execute if the condition is true, followed by a colon (:), and finally, an expression to execute if the condition is false. The syntax of the ternary operator is condition ? expressionIfTrue : expressionIfFalse
.
let age = 20;
let message = (age < 18) ? "You are too young to vote." : "You can vote!";
console.log(message); // output: "You can vote!"
In the example above, the condition (age < 18)
is evaluated first. If the condition is true, the value “You are too young to vote.” is assigned to the variable message
. Otherwise, the value “You can vote!” is assigned to the variable message
. The ternary operator is a shorthand way of writing an if-else
statement and is often used in place of if-else
statements for simple conditions. It can make code shorter and easier to read.
Javascript Assignment Operators
Assignment operators in JavaScript are used to assign values to variables. They allow you to modify a variable’s value without reassigning the entire value. This can make your code more concise and readable. Assignment operators combine the operation with the assignment, such as x += y
, which is the same as x = x + y
. There are several assignment operators in JavaScript, including =
, +=
, -=
, *=
, /=
, %=
, <<=
, >>=
, &=
, |=
, and ^=
. These operators perform a wide range of operations, from simple assignment to bitwise operations.
Operator | Example | Equivalent to |
---|---|---|
= | x = y | x = y |
+= | x += y | x = x + y |
-= | x -= y | x = x – y |
*= | x *= y | x = x * y |
/= | x /= y | x = x / y |
%= | x %= y | x = x % y |
<<= | x <<= y | x = x << y |
>>= | x >>= y | x = x >> y |
&= | x &= y | x = x & y |
= | x | |
^= | x ^= y | x = x ^ y |
= (Simple Assignment)
The simple assignment operator assigns a value to a variable. The value can be a constant or the result of an expression. For example, x = 10
assigns the value 10 to the variable x
. It can also assign the value of the right operand to the left operand.
let x;
x = 10; // assigns the value 10 to x
let x = 5;
let y = 10;
x = y;
console.log(x); // Output: 10
+= (Addition Assignment Operator)
What += means in JavaScript? The addition assignment operator adds the value of the right operand to the left operand and assigns the result to the left operand. For example, x += 5
is the same as x = x + 5
.
let x = 10;
x += 5; // adds 5 to x and assigns the result (15) to x
= (Subtraction Assignment Operator)
The subtraction assignment operator subtracts the value of the right operand from the left operand and assigns the result to the left operand. For example, x -= 5
is the same as x = x - 5
.
let x = 10;
x -= 5; // subtracts 5 from x and assigns the result (5) to x
= (Multiplication Assignment Operator)
The multiplication assignment operator multiplies the value of the right operand by the left operand and assigns the result to the left operand. For example, x *= 5
is the same as x = x * 5
.
let x = 10;
x *= 5; // multiplies x by 5 and assigns the result (50) to x
/= (Division Assignment Operator)
The division assignment operator divides the value of the left operand by the right operand and assigns the result to the left operand. For example, x /= 5
is the same as x = x / 5
.
let x = 10;
x /= 5; // divides x by 5 and assigns the result (2) to x
%= (Modulus Assignment Operator)
The modulus assignment operator divides the value of the left operand by the right operand and assigns the remainder to the left operand. For example, x %= 3
is the same as x = x % 3
.
let x = 10;
x %= 3; // divides x by 3 and assigns the remainder (1) to x
<<= (Left Shift Assignment Operator)
The left shift assignment operator left shifts the value of the left operand by the number of bits specified by the right operand and assigns the result to the left operand. For example, x <<= 2
is the same as x = x << 2
.
let x = 10; // binary representation: 1010
x <<= 2; // left shifts x by 2 bits (resulting in 101000) and assigns the result (40) to x
>>= (Right Shift Assignment Operator)
The right shift assignment operator right shifts the value of the left operand by the number of bits specified by the right operand and assigns the result to the left operand. For example, x >>= 1
is the same as x = x >> 1
.
let x = 10; // binary representation: 1010
x >>= 1; // right shifts x by 1 bit (resulting in 101) and assigns the result (5) to x
&= (Bitwise AND Assignment Operator)
The bitwise AND assignment operator (&=) performs a bitwise AND operation between the binary representations of the left and right operands and then assigns the result to the left operand.
Example:
let x = 5;
let y = 10;
x &= y;
console.log(x); // Output: 0
|= (Bitwise OR assignment)
The bitwise OR assignment operator (|=) performs a bitwise OR operation between the binary representations of the left and right operands and then assigns the result to the left operand.
Example:
let x = 5;
let y = 10;
x |= y;
console.log(x); // Output: 15
^= (Bitwise XOR assignment)
The bitwise XOR assignment operator (^=) performs a bitwise XOR operation between the binary representation of its operands and assigns the result to the left operand. It returns the new value of the left operand after the assignment.
let a = 5; // 101 in binary
let b = 3; // 011 in binary
a ^= b; // a becomes 110 in binary (6 in decimal)
console.log(a); // output: 6
Javascript Bitwise Operators
Javascript Bitwise operators allow you to manipulate binary data in your code. This includes operations such as AND (&), OR (|), XOR (^), left shift (<<), right shift (>>), and unsigned right shift (>>>). While Javascript bitwise operators are less commonly used than arithmetic, comparison, or logical operators, they are essential for certain programming tasks.
& (Bitwise AND)
The bitwise AND operator (&) performs a bitwise AND operation on the binary representations of its operands. It returns a new binary number with a 1 bit in each position where both operands have a 1 bit.
Example:
console.log(5 & 3); // 1
// binary 5: 101
// binary 3: 011
// result: 001
| (Bitwise OR)
The bitwise OR operator (|) performs a bitwise OR operation on the binary representations of its operands. It returns a new binary number with a 1 bit in each position where at least one of the operands has a 1 bit.
Example:
console.log(5 | 3); // 7
// binary 5: 101
// binary 3: 011
// result: 111
^ (Bitwise XOR)
The bitwise XOR operator (^) performs a bitwise XOR (exclusive OR) operation on the binary representations of its operands. It returns a new binary number with a 1 bit in each position where exactly one of the operands has a 1 bit.
Example:
console.log(5 ^ 3); // 6
// binary 5: 101
// binary 3: 011
// result: 110
<< (Left Shift)
The left shift operator (<<) shifts the binary representation of its left operand to the left by the number of bits specified by its right operand. It returns a new binary number with the shifted bits filled in with zeros.
Example:
console.log(5 << 1); // 10
// binary 5: 101
// result: 1010
>> (Right Shift)
The right shift operator (>>) shifts the binary representation of its left operand to the right by the number of bits specified by its right operand. It returns a new binary number with the shifted bits filled in with zeros on the left and the rightmost bits truncated.
Example:
console.log(5 >> 1); // 2
// binary 5: 101
// result: 10
>>> (Unsigned Right Shift)
The unsigned right shift operator (>>>) shifts the binary representation of its left operand to the right by the number of bits specified by its right operand. It returns a new binary number with the shifted bits filled in with zeros on the left and the rightmost bits truncated, but it treats the leftmost bit as a regular bit instead of a sign bit.
Example:
console.log(-5 >>> 1); // 2147483645
// binary -5: 11111111111111111111111111111011
// result: 01111111111111111111111111111101
What does 3 dots mean in JavaScript?
The three dots in JavaScript, also known as the spread operator, are used to spread the elements of an array or an object. When used in an array, the spread operator can be used to concatenate arrays or add elements to an existing array. When used with an object, it can be used to create a new object that includes the properties of an existing object.
For example, let’s say we have two arrays and we want to concatenate them using the spread operator:
const arr1 = [1, 2, 3];
const arr2 = [4, 5, 6];
const arr3 = [...arr1, ...arr2];
console.log(arr3); // Output: [1, 2, 3, 4, 5, 6]
In this example, we used the spread operator to spread the elements of arr1
and arr2
into a new array called arr3
.
The spread operator can also be used with objects:
const obj1 = { a: 1, b: 2 };
const obj2 = { c: 3, d: 4 };
const obj3 = { ...obj1, ...obj2 };
console.log(obj3); // Output: { a: 1, b: 2, c: 3, d: 4 }
In this example, we used the spread operator to create a new object called obj3
that includes the properties of obj1
and obj2
.
Use This CheatSheet and Save Time
You now have a handy reference to the most commonly used operators, including their descriptions and examples of how to use them. You’ll have a quick and easy reference for all the syntax, symbols, and rules associated with each type of operator. Whether a beginner or an experienced developer, the cheat sheet will help you save time and avoid mistakes when working with JavaScript operators. So keep it handy and refer to it whenever you need to work with operators in JavaScript.