- What are the Two Types of Components in React?
- Why Use React Functional Components?
- What is the difference between class component and functional component in React?
- How to Create a Functional Component in React
- React Functional Components and TypeScript
- Callback Functions in React Functional Components
- Should I use functional or class components?
- The Future of Functional Components
If you’re looking to build user interfaces using React, you’re likely already familiar with React components. Components are the building blocks of a React application, and there are two types: class components and functional components. While both can achieve similar functionality, functional components have become increasingly popular over the years due to their simplicity, reusability, and performance. In fact, functional components are the future of React development. Don’t take our word for it. According to a survey conducted by the State of JavaScript in 2020, 66.9% preferred using functional components over class components.
This article explores the advantages of using functional components over class components. We’ll dive deep into functional components and how they differ from class components and show you how to create them. We will compare the syntax, state, and lifecycle methods of the two types of components. We’ll also cover event handlers and callback functions in functional components and explain when to use functional components versus class components.
By the end of this article, you’ll have a comprehensive understanding of functional components in React and why they are the way forward in React development. Whether you’re a beginner or an experienced React developer, this article is a must-read. So, let’s dive into the world of functional components in React!
What are the Two Types of Components in React?
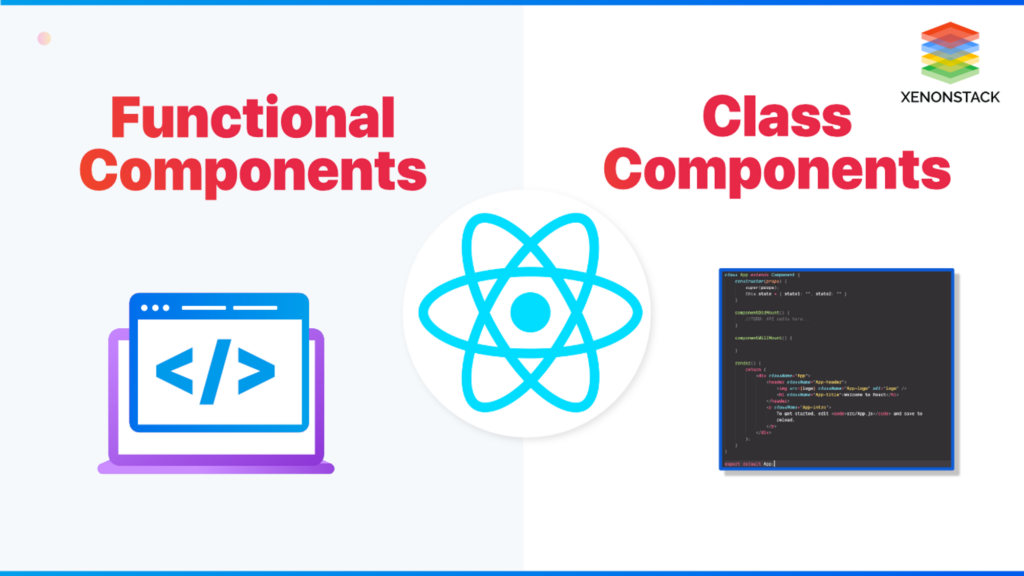
Now that we know the two types of components in React let’s take a closer look at functional components. We’ll explore what they are, how they differ from class components, and why you should use them in your React applications.
What are Functional Components in React?
Functional components are a type of React component that use JavaScript functions to define the structure of the component’s output. They were introduced in React 16.8 as part of the Hooks API. Functional components are simpler, more reusable, and more performant than class components.
Functional components take props (short for properties) as an input and return JSX (a syntax extension for JavaScript) to describe the structure of the component’s output. React functional components props are a way of passing data from a parent component to a child component.
Functional components can also use state and other React features using the useState and other Hooks provided by React.
Here’s 6-minute video by Codevolution that quickly explains React functional components:
What are Class Components?
Class components are a type of React component that uses JavaScript classes to define the structure of the component’s output. They extend the React.Component class and use the render method to define the component’s output.
Class components can have state and lifecycle methods, such as componentDidMount, componentDidUpdate, and componentWillUnmount. They also take props as input and can use them to define the structure of the component’s output.
Class components were the primary way of creating React components before introducing functional components and the Hooks API in React 16.8. However, functional components have become increasingly popular and are now considered the way forward in React development.
The next section will explore the advantages of using functional components over class components. We’ll dive deep into the benefits of functional components and how they can improve your React development experience.
Here’s another brilliant video by Codevolution that explains class components:
Why Use React Functional Components?
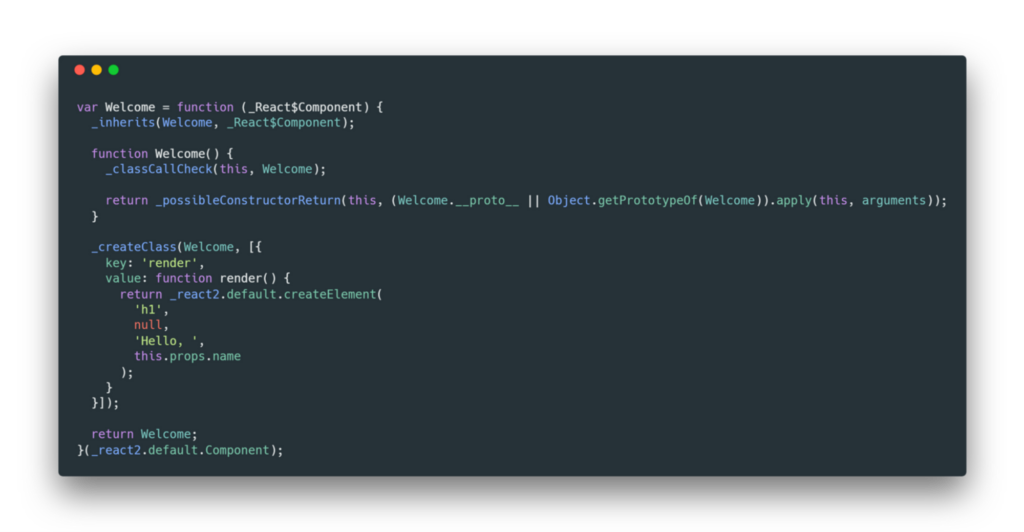
Functional components have gained popularity among React developers for several reasons. This section will explore the advantages of using functional components in React development and compare them to class components.
Advantages of Using Functional Components in React Development
One advantage of functional components is that they are simpler and easier to read than class components. Since they are plain JavaScript functions, they have a straightforward structure and are less verbose than class components. This makes them easier to maintain and debug, especially for smaller projects.
Functional components are also more reusable than class components. Since they do not have state or lifecycle methods, they can be easily shared between different parts of an application. This makes it easier to build modular and maintainable codebases.
Another advantage of functional components is that they are more performant than class components. Since they do not have the overhead of a class instance, they are faster to render and update. This is particularly important for large applications with complex UIs and data flows.
What is the difference between class component and functional component in React?
While class components are still used in many React applications, functional components offer several advantages over class components. Here are some key differences between the two:
- Structure: Functional components are more straightforward than class components.
- Reusability: Functional components are more reusable and can be easily shared between different parts of an application.
- Performance: Functional components are more performant than class components, especially for large applications.
To help illustrate these differences, we’ve created a comparison table of class components vs. functional components.
Table 1: React Functional Components vs Class Components
Let’s compare functional components to class components in more detail:
Feature | Class Components | Functional Components |
State | Have state and use this.setState() | Use state hook |
Lifecycle methods | Have lifecycle methods | Use effect hook |
Code structure | Use classes | Use functions |
Reusability | Less reusable | More reusable |
Performance | More overhead | Less overhead |
Learning curve | Steeper learning curve | Easier to learn |
Table 1: Comparison of Class Components vs. Functional Components
As you can see from the comparison, functional components have several advantages over class components. They are more straightforward, reusable, and performant, making them the future of React development.
In the next section, we’ll explore creating a functional component in React using TypeScript. We’ll examine the benefits of using TypeScript with functional components and how it can improve your development experience.
How to Create a Functional Component in React
Creating a functional component in React is a straightforward process. Here are the steps to follow:
- Import React at the top of your file:
import React from 'react';
- Define your functional component using an arrow function:
const MyComponent = (props) => {
// JSX code here
}
3. Use props to pass data from a parent component to a child component. React functional components props are passed as an object to the function:
const MyComponent = (props) => {
return <h1>{props.title}</h1>
}
4. Use JSX to define the structure of your component’s output:
const MyComponent = (props) => {
return (
<div>
<h1>{props.title}</h1>
<p>{props.description}</p>
</div>
)
}
5. Export your component using export default MyComponent;
That’s it! Your functional component is now ready to use in your React application.
In the next section, we’ll explore event handlers and callback functions in functional components after discussing React functional components and typescript.
React Functional Components and TypeScript
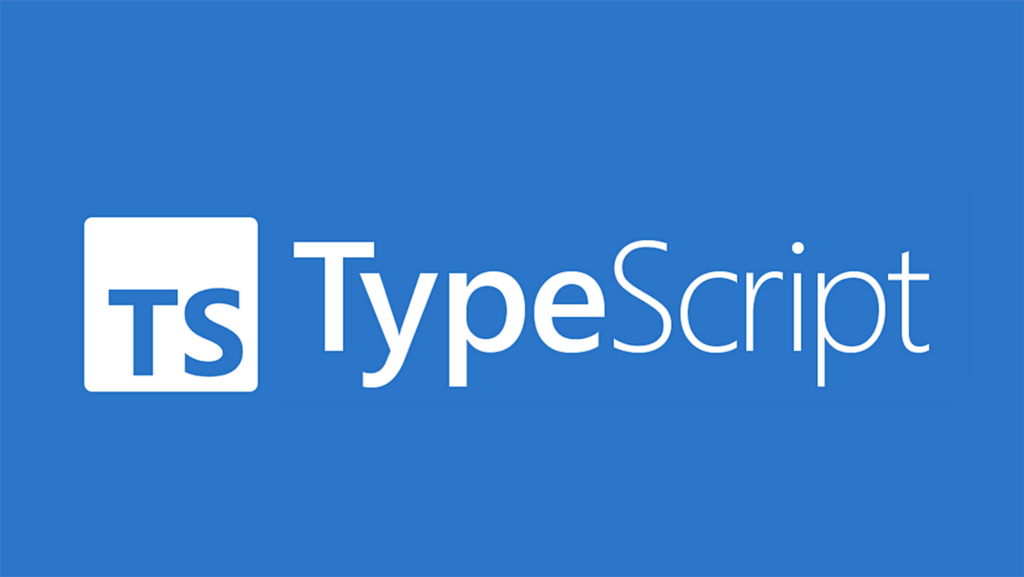
React functional components can be used with TypeScript, a popular statically-typed superset of JavaScript. TypeScript provides additional syntax and features that help catch errors early in development and make code easier to maintain.
One of the main benefits of using TypeScript with React is the ability to define interfaces for props and state. This helps ensure that components receive the correct data types and prevents common errors caused by passing the wrong data types.
To use TypeScript with React, you can define interfaces for props and state in your functional components:
interface Props {
name: string;
age: number;
}
const MyComponent: React.FC<Props> = ({ name, age }) => {
// component logic here
};
In this example, we’ve defined an interface called Props
that specifies the expected shape of the props
object passed to MyComponent
. We’ve also used the React.FC
type to indicate that MyComponent
is a functional component that takes Props
as its input.
TypeScript can also help catch errors related to event handlers and callback functions. For example, if you define an event handler function that takes an incorrect parameter type, TypeScript will catch this error at compile-time:
const handleClick = (event: MouseEvent<HTMLButtonElement>) => {
// handle click event here
};
// TypeScript will catch this error:
<button onClick={handleClick}>Click me</button>
In this example, we’ve defined a handleClick
function that takes a MouseEvent
parameter. If we try to use this function as an event handler for a non-MouseEvent
event (e.g. a KeyboardEvent
), TypeScript will catch this error and prevent it from causing a runtime error.
In summary, using TypeScript with React functional components can help catch errors early in the development process, make code easier to maintain, and improve the overall quality of your React applications.
Event Handlers in React Functional Components
Event handlers are a crucial part of any UI development. They allow us to respond to user interactions and create dynamic user interfaces. In React, event handling differs slightly from traditional JavaScript event handling.
In functional components, event handlers are defined as functions passed as props to the component. These functions are then called when the corresponding event occurs. For example, a click event on a button would trigger the function passed as the onClick prop.
Let’s take a look at an example:
import React from 'react';
function Button(props) {
return <button onClick={props.onClick}>Click me</button>;
}
function App() {
function handleClick() {
console.log('Button clicked');
}
return <Button onClick={handleClick} />;
}
In this example, a Button component takes an onClick prop. When the button is clicked, the function passed as the onClick prop is called. In the App component, we define a handleClick function that logs a message to the console. We pass this function as the onClick prop to the Button component.
In this way, we can define event handlers as standalone functions and pass them as props to the necessary components. This makes our code more modular and easier to reason about.
It’s worth noting that we can also define event handlers inline using arrow functions:
import React from 'react';
function Button(props) {
return <button onClick={() => props.onClick('Button clicked')}>Click me</button>;
}
function App() {
function handleClick(message) {
console.log(message
Callback Functions in React Functional Components
Callback functions are a critical part of React functional components. They handle user interactions and state changes in a React application. A callback function is a function that is passed as a prop to a child component and is called when an event occurs in the child component.
For example, suppose you have a button component that needs to trigger an action in the parent component when clicked. You would pass a callback function as a prop to the button component. The callback function will be executed in the parent component when the button is clicked.
Here’s an example of how you can use a callback function in a React functional component:
import React from 'react';
function Button(props) {
return (
<button onClick={props.onClick}>
{props.label}
</button>
);
}
function App() {
function handleClick() {
console.log('Button was clicked');
}
return (
<div>
<h1>Callback Functions in React Functional Components</h1>
<Button onClick={handleClick} label="Click me" />
</div>
);
}
export default App;
In this example, the Button
component accepts a prop called onClick
, a callback function called when the button is clicked. The App
component defines the handleClick
function, which logs a message to the console when called. When the Button
component is clicked, the handleClick
function is executed.
Using callback functions in React functional components allows you to write more modular and reusable code. You can pass a callback function as a prop to any component that needs to handle an event or state change, regardless of its position in the component tree.
Should I use functional or class components?
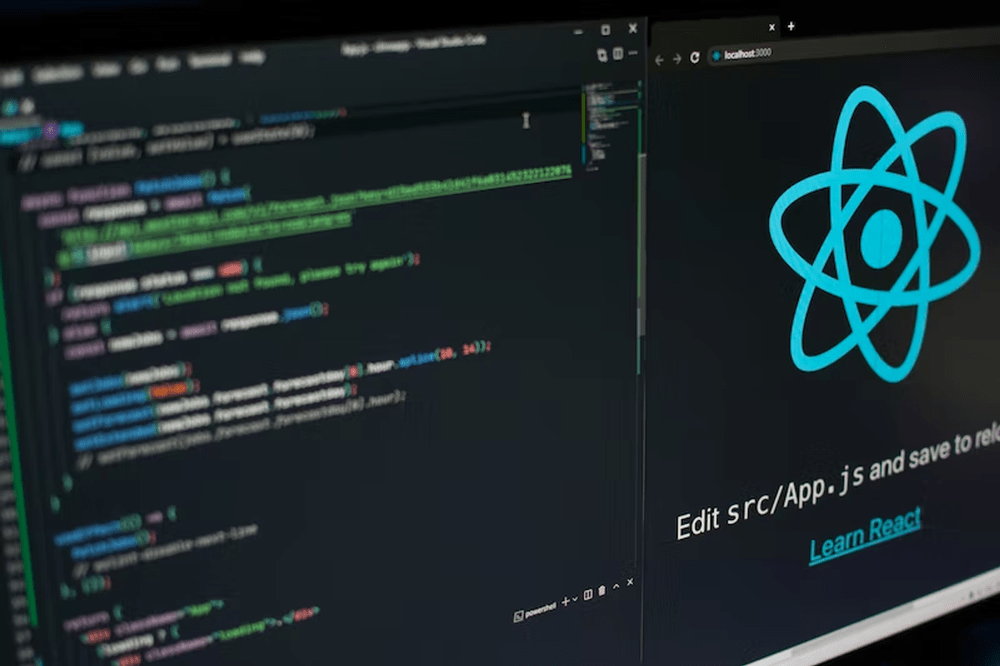
While functional components are generally preferred over class components, there are still some situations where class components may be more appropriate. Here are a few factors to consider when deciding whether to use functional or class components in your React application:
- Component complexity: If you’re building a relatively simple component that doesn’t need to manage state or implement lifecycle methods, a functional component may be the better choice. However, if you’re building a more complex component that requires state management and/or lifecycle methods, a class component may be more appropriate. One such case is when you need to use lifecycle methods, such as componentDidMount or componentDidUpdate.
- Team experience: If your team has more experience with class components or is more comfortable working with them, stick with class components for consistency and ease of maintenance.
- Existing codebase: If your application already has many class components, switching everything over to functional components may not make sense. In this case, sticking with class components for consistency may be better.
- TypeScript usage: If you’re using TypeScript in your application, functional components can provide additional benefits such as better type checking and improved code maintainability.
Ultimately, whether to use functional or class components depends on various factors and will vary from project to project. However, as a general rule, functional components are a great choice for building React’s simple, reusable, and performant components.
The Future of Functional Components
As React evolves, functional components will become even more central to React development. The increasing popularity of hooks and the simplicity and performance of functional components also means that they will likely become the default choice for React developers. With the introduction of features like Suspense and Concurrent Mode, functional components are set to become even more powerful and versatile. Whether you’re building a small React application or a large-scale enterprise solution, functional components are a powerful tool that should be in every React developer’s toolkit.