- Introduction
- Identifying Performance Issues
- A. Using developer tools to measure performance
- B. Identifying common performance bottlenecks in React apps and how to solve them
- C. Strategies for tracking performance issues over time and which projects they pair best with
- Performance Optimization in React Applications
- A. Reducing the number of re-renders:
- Conclusion
Introduction
React is a popular JavaScript library for building user interfaces, known for its efficiency and flexibility. However, as applications grow in complexity, performance issues can arise. Slow load times, stuttering animations, and other user-facing problems can negatively impact the user experience. Ensuring that a React application is fast and responsive is crucial for providing a good user experience. This article will explore identifying performance-related issues in a React application and strategies for performance optimization and improvement. We will discuss how to use developer tools to measure performance, identify common performance bottlenecks, and track performance issues over time. Additionally, we will explore various techniques for optimizing a React application, such as reducing the number of re-renders, improving rendering performance, lazy loading and code splitting, and optimizing assets. The information will equip you to identify and optimize performance issues in your React applications.
Identifying Performance Issues
Identifying performance issues is an essential step in optimizing a React application because it allows you to pinpoint the source of the problems. You need to identify the specific points to know where to focus your optimization efforts, and you may make changes that don’t improve performance. Using developer and performance monitoring tools, you can gather data on the application’s load time, runtime performance, and other metrics that can help you identify bottlenecks.
Additionally, by identifying common performance issues such as overuse of state and props, unoptimized rendering with React hooks, and large component trees, you can focus your optimization efforts on the areas that will most impact the performance of the application. Furthermore, having a strategy for tracking performance issues over time, like setting up monitoring and logging, can help you detect any performance degradations that might happen in the future and also see if your optimization efforts are paying off and where you should focus next. In the following sections of this article, we will delve deeper into each of these three key areas – using developer tools to measure performance, identifying common performance bottlenecks, and tracking performance issues over time – to provide a comprehensive understanding of how to identify performance issues in a React application and make data-driven optimization decisions.
A. Using developer tools to measure performance
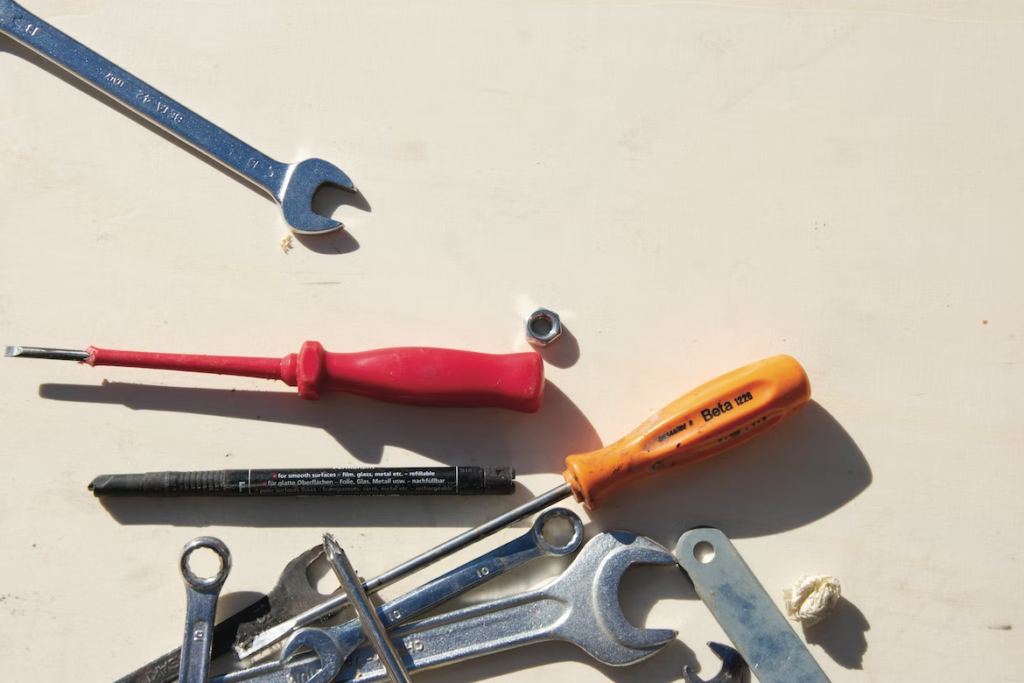
The first step in identifying performance issues is to measure the application’s performance. The browser’s built-in developer tools, such as the performance tab, provide valuable information about the application’s load time and runtime performance. The performance tab allows you to record a browser session, analyze the data and identify where the bottlenecks are.
Additionally, there are several third-party tools available. Below are some of the popular tools:
- Chrome DevTools: Chrome DevTools is a set of web development tools built into Google Chrome. It includes a performance tab that allows you to record a browser session, analyze the data, and identify where the bottlenecks are. You can access the DevTools by opening Chrome and pressing F12 or by right-clicking on a page and selecting “Inspect.”
- React DevTools: React DevTools is a browser extension that allows you to inspect the component tree, observe component props and state, and track the component’s lifecycle methods. This can help you identify where performance issues are stemming from. It is available for Chrome and Firefox.
- Lighthouse: Lighthouse is an open-source, automated tool for improving the quality of web pages. It has a performance audit that can be run on any web page, and it generates a report on load performance, accessibility, best practices, and more. It can be run from within Chrome DevTools or as a command line tool.
- WebPageTest: WebPageTest is a web performance testing tool that allows you to test the load time and performance of a web page from multiple locations around the world using real browsers. It provides a detailed report with a filmstrip view and can be run from the command line or through a web interface.
B. Identifying common performance bottlenecks in React apps and how to solve them
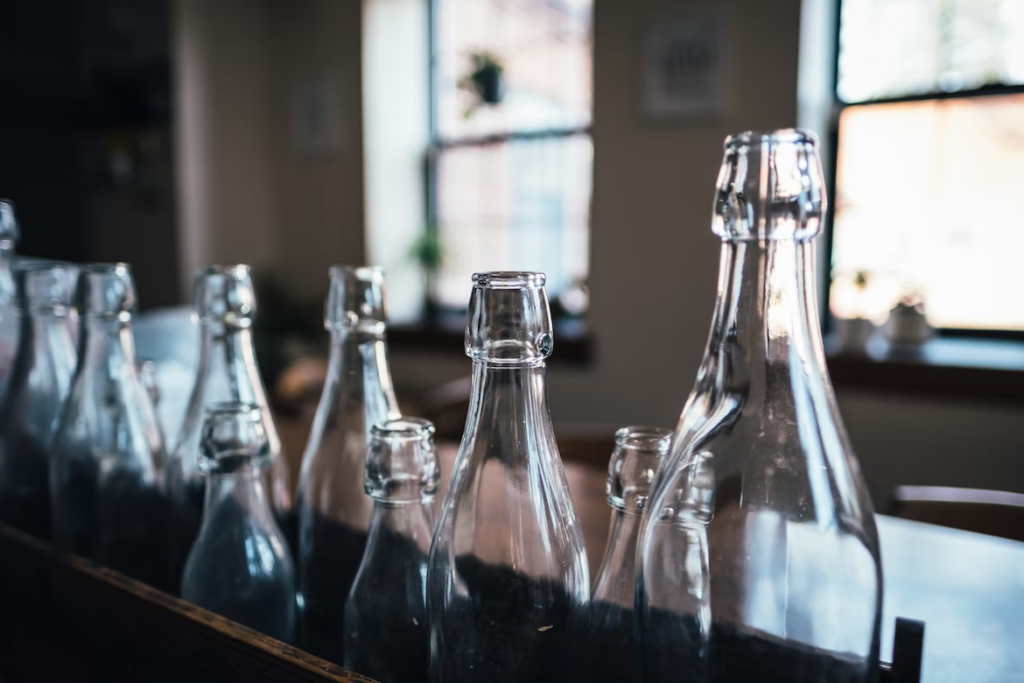
Once you have gathered performance data, the next step is identifying the performance bottlenecks. Some common performance issues in React applications include overuse of state and props, unoptimized rendering with React hooks, large component trees, and more. We will discuss each point in more detail, along with solutions.
Overuse of state and props
React’s architecture is based on a unidirectional data flow, where the parent component passes data down to its children via props. When a prop or a state changes, the component that depends on it will re-render. Overuse of state and props can cause unnecessary re-renders, slowing down the application’s performance.
Solution: You can use techniques such as lifting state up, and also consider using the useReducer hook instead of useState when you have complex state logic. Additionally, you can avoid passing unnecessary props to child components. Watch a quick tutorial on how to use useReducer.
Unoptimized rendering with React Hooks
React hooks provide a way to use state and other features in functional components. However, if they are not used correctly, they can cause unnecessary re-renders and negatively impact performance.
Solution: You can use the useMemo hook to memoize expensive calculations. However, be careful with hooks such as useEffect that can lead to unnecessary re-renders and limit the number of hooks used in a component.
Large component trees
As an application grows in complexity, the component tree can become large and unwieldy. When a component re-renders, React will also re-render all of its children. If a component has a large number of children, this can slow down the application’s performance.
Solution: You can split a large component into smaller, more focused components, reducing the number of re-renders and improving the application’s performance. Additionally, you can use the React Developer Tools extension to identify components that have many children and consider refactoring them.
Unnecessary re-renders
When a component re-renders, React will also re-render all of its children. Unnecessary re-renders can slow the application’s performance and cause the user interface to stutter. This can be caused by incorrectly using the setState method or having a component that updates too frequently.
Solution: You can use techniques such as React.memo and React.useCallback to memoize components and callback functions, respectively. This can prevent unnecessary re-renders when props or states haven’t changed. You can also use the shouldComponentUpdate lifecycle method to control when a component should re-render. Learn how to use React.useCallback in 8 minutes:
Poorly optimized images and videos
Large, unoptimized images and videos can significantly slow down the load time of an application. This can be caused by not compressing images or videos before they are loaded or by using images and videos much larger than they need to be.
Solution: You can use tools like image compression libraries and image optimization tools to compress and optimize images and videos before loading them. This can significantly reduce the file size of images and videos and improve the application’s load time.
Inefficient use of the DOM
The Document Object Model (DOM) is a programming interface for HTML and XML documents. React uses the DOM to update the user interface, and if the updates are not optimized, it can slow down the application’s performance. This can happen when a component updates the DOM excessively or when a component inefficiently updates the DOM.
Solution: You can use techniques such as shouldComponentUpdate, useMemo or useCallback hooks to control when a component updates the DOM. Additionally, you can use the React Developer Tools extension mentioned earlier to identify components that are updating the DOM excessively or that are updating it inefficiently.
C. Strategies for tracking performance issues over time and which projects they pair best with
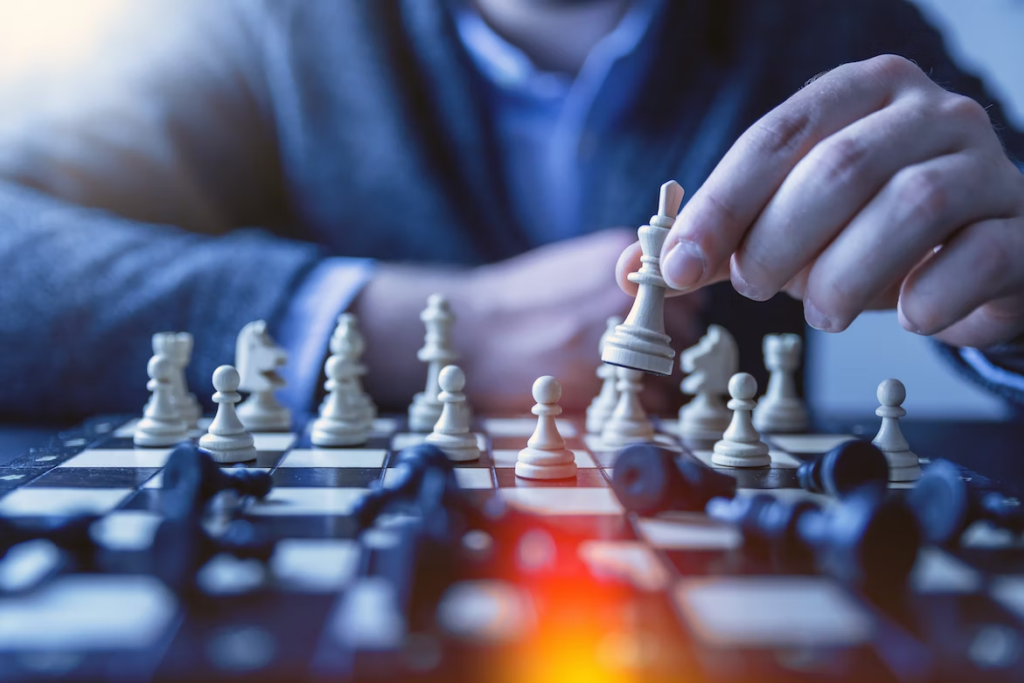
Once you have identified performance issues, it’s important to have a strategy for tracking them over time. There are several strategies you can use to best track performance issues over time. Read on to learn about each one and which is best for certain projects.
Setting up monitoring and logging
Setting up monitoring and logging systems can help you automatically detect performance degradation and notify you when they occur. There are several tools you can choose from,
New Relic: New Relic is a performance monitoring tool that can be used to track a wide range of performance metrics, including response time, error rate, and throughput. It also offers a range of additional features, such as real-user monitoring and error tracking.
Google Analytics: A free web analytics service that tracks and reports website traffic. It can also be used to track performance metrics such as page load time and bounce rate.
AppDynamics: A performance monitoring tool that can track performance metrics in real time and provides deep visibility into the underlying infrastructure.
Datadog: Datadog is a performance monitoring tool that allows you to collect, store and analyze performance data from various sources, including servers, databases, and applications.
These tools track performance metrics such as load time, response time, and error rate over time and also replay user sessions to understand how the application is being used. These can help you identify performance issues that may take time to be obvious.
Best for: Larger, more complex projects where the volume of data and the number of users can make it difficult to track performance issues manually. Automated monitoring and logging systems can help you automatically detect performance degradation and notify you when they occur.
Analyzing performance metrics
Analyzing performance metrics such as load time, response time, and the error rate can help you identify trends and patterns in the performance of your application. This can help you determine when performance issues are starting to occur and take action before they become critical.
Best for: Projects of any size, as it can help you identify trends and patterns in the performance of your application. Set up performance metrics that are meaningful for your specific application and track them regularly.
A/B testing
A/B testing allows you to test different application versions and compare their performance. This can help you identify which changes have the most significant impact on performance and make data-driven decisions.
Best for: Projects looking to make specific changes to the application, such as redesigning a user interface or adding new features. A/B testing can help you identify which changes have the most significant impact on performance and make data-driven decisions.
User Feedback
User feedback can be a valuable source of information when tracking performance issues over time. Collecting user feedback can help you identify problems that may take time to be obvious, such as slow load times, poor user experience, and other performance-related problems.
Best for: Projects that rely heavily on user feedback, such as consumer-facing applications. Collecting user feedback can help you identify issues that may not be immediately obvious, such as slow load times, poor user experience, and other performance-related problems.
Regular performance testing
Regularly testing your application’s performance can help you identify issues early and take action before they become critical. This can include automated and manual testing, such as load testing, stress testing, and end-to-end testing.
Best for: Projects of any size, as it can help you identify issues early and take action before they become critical. This can include automated and manual testing, such as load testing, stress testing, and end-to-end testing.
Performance Optimization in React Applications
Optimizing React applications is essential because it ensures that the application is fast and responsive for the end user. Poorly optimized applications can lead to slow load times, poor user experience, and high bounce rates. Optimizing an application can improve the application’s performance, reduce the number of re-renders, improve rendering performance, lazy loading and code splitting, and optimize assets. Additionally, performance optimization is an ongoing process. It’s essential to regularly monitor and analyze performance metrics to ensure that the application remains fast and responsive over time. Optimizing React applications can also lead to increased user engagement, higher conversion rates, and, ultimately, better business results. Below, we discuss some ways how to optimize your react application best.
A. Reducing the number of re-renders:
Reducing the number of re-renders is one of the most effective ways to improve the performance of a React application. There are several techniques you can use to minimize re-renders, including React.memo, React.useCallback, and splitting components into smaller ones.
- React.memo: React.memo is a higher-order component that can be used to wrap a functional component. It memoizes the component, meaning it will only re-render it if its props have changed. This can significantly reduce the number of unnecessary re-renders and improve the application’s performance. Learn how to use React Memo with examples.
- React.useCallback: React.useCallback is a hook that can be used to memoize a callback function. This means that the function will only be recreated if its dependencies have changed. This can be particularly useful for passing callback functions as props to child components, as it can prevent unnecessary re-renders. Learn how to use React.useCallback hook with examples.
- Splitting components into smaller ones: A common performance bottleneck in React applications is large component trees. When a component re-renders, React will also re-render all of its children. By splitting a large component into smaller, more focused components, you can reduce the number of re-renders and improve the application’s performance.
Improving rendering performance
Another way to improve the performance of a React application is by optimizing the rendering performance. The virtual DOM is a crucial feature of React that helps to improve the performance of an application by minimizing the number of changes that need to be made to the actual DOM. Additionally, optimizing component logic, like avoiding unnecessary calculations, can help improve performance.
Lazy loading and code splitting:
Lazy loading and code splitting can be used to improve the performance of a React application by loading only the code needed for a specific route or feature. This can significantly reduce the application’s initial load time and improve users’ performance. Read a great guide to learn how to use React Lazy Loading and Code Splitting best.
Optimizing assets:
As mentioned in the previous section, optimizing assets such as images and videos is another way to improve the performance of a React application. By compressing images and videos, you can reduce their file size and improve the application’s load time. Minimizing and bundling JavaScript and CSS files can also improve the application’s performance.
Conclusion
This article discussed various techniques for identifying and optimizing performance issues in a React application. We covered how to use developer tools to measure performance, identify common performance bottlenecks, and track performance issues over time. Additionally, we explored various techniques for performance optimization in a React application, such as reducing the number of re-renders, improving rendering performance, lazy loading and code splitting, and optimizing assets.
Our article will equip you to identify and optimize your React applications’ performance issues. However, performance optimization is an ongoing process, and monitoring and analyzing performance metrics is essential to ensure that the application remains fast and responsive. To make performance optimization process smoother, we recommend using CopyCat, a tool that helps you focus on making your application the best it can be while it takes care of the mundane React UI building work. Try it for yourself!
Related Reads from Our Blog: