- Prerequisites
- What is a TypeScript Class?
- Should I use classes in Typescript?
- Is there a class type in TypeScript?
- Can I use TypeScript class in JavaScript?
- Class inheritance in TypeScript
- Access Modifiers
- Creating an Object of Class
- Using Classes as Types
- Getters and Setters Methods in TypeScript
- Defining Class properties in Constructors
- Conclusion
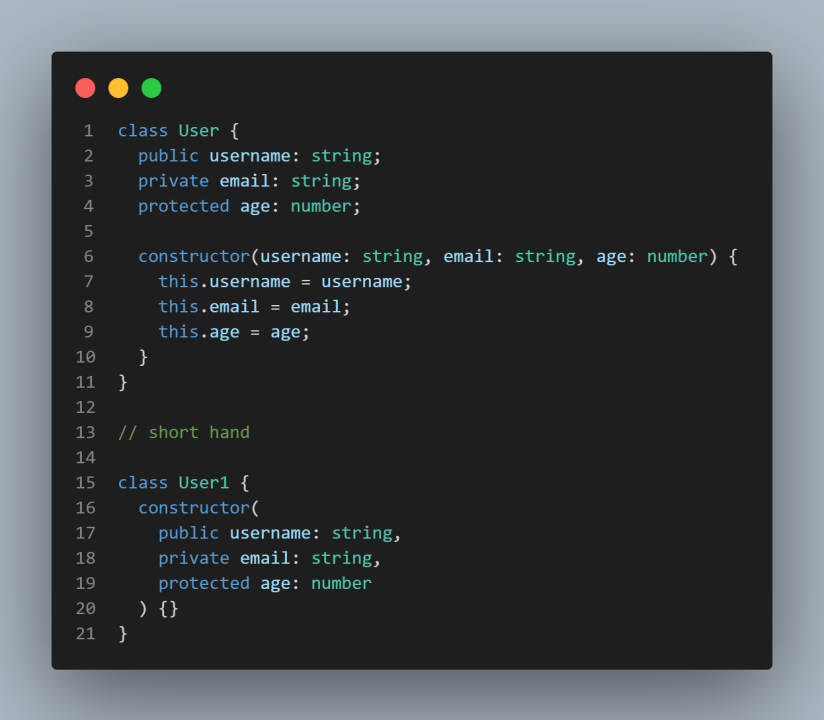
Microsoft created and maintains the well-known programming language known as Typescript. It is a tight superset of JavaScript in terms of syntax and gives the language the option of static typing. Classes, which gives you a mechanism to build reusable parts into your code, is one of Typescript’s main features.
This article will teach the idea of classes in Typescript and describe how to utilize them in your applications.
A tool for encapsulating data and action in Typescript is the class. They offer a mechanism to build and work with objects. They also let you specify the attributes and procedures that make up an object’s behavior. Classes are an effective tool for writing adaptable and reusable code since they can be tweaked and expanded.
With their aid, you may write more extensible, modular code that will be simpler to maintain and update over time.
Before we go on if you’re searching for a fantastic React tool to help you build component code quicker and be production ready, check out the CopyCat plugin for React.
Prerequisites
To benefit more from this article you must have;
- Basic knowledge and understanding of Javascript ES6
- Basic knowledge and hands-on experience with Typescript
What is a TypeScript Class?
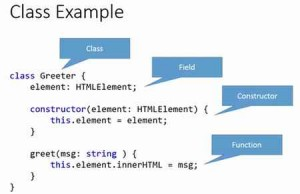
The blueprint for building things with certain features and behaviors is a TypeScript class. A class is a template in TypeScript that specifies the state (properties) and operation (methods) of a collection of objects.
Classes in TypeScript are comparable to classes in Java or C#, two major object-oriented programming languages. They make it simpler to construct and deal with complicated data structures. You’ll be able to specify the structure and behavior of objects in a clear and orderly fashion.
Here is a straightforward TypeScript class code illustration:
class Person {
firstName: string;
lastName: string;
constructor(firstName: string, lastName: string) {
this.firstName = firstName;
this.lastName = lastName;
}
sayHello() {
console.log(`Hello, my name is ${this.firstName} ${this.lastName}.`);
}
}
FirstName and LastName are two attributes and one method of the Person class in this example (sayHello). When a new object is generated from the class, a particular function called the constructor is invoked. A greeting message may be printed on an object by using the normal method sayHello.
You may implement a new object and invoke the methods of the Person class to use it. See the example below:
const person = new Person('John', 'Doe');
person.sayHello(); // Output: "Hello, my name is John Doe."
In this illustration, the person variable is given a new Person object, which is generated. Then the person object’s sayHello function is invoked, which outputs the welcome message to the console.
Overall, the structure and behavior of objects in your application may be defined with robust and adaptable TypeScript classes.
Should I use classes in Typescript?
The decision to utilize classes in TypeScript or not mostly depends on your preferences and the demands of your project. Classes help you organize your code and can make it simpler to construct and operate with complicated data structures.
Classes are not, however, always the ideal option for every circumstance. You might not even need to utilize classes if you are working on a small or straightforward project. Especially, if you prefer a more functional programming approach.
Additionally, you must bear in mind that TypeScript is a superset of JavaScript. This means you can employ either class-based or non-class-based techniques in your TypeScript code. You can utilize classes when they are the best for the task and select the best strategy for your project.
In summary, your personal tastes and the unique requirements of your project will determine whether or not you should utilize classes in TypeScript. It is important to weigh the benefits and drawbacks of classes before determining whether to use them in your project.
Is there a class type in TypeScript?
The answer is that TypeScript has a class type. To describe the type of a variable that will hold an instance of the class, use the class type, which symbolizes the constructor function of a class.
To guarantee that objects have a specific structure, you may use this class type in the same way you would use the type of any other object. Additionally, to guarantee that an object has specific properties or to describe the kinds of those attributes, you can use the class type. In doing so, you may ensure that the objects derived from your classes adhere to a particular amount of structure and consistency.
Here is an illustration of how to apply TypeScript’s class type:
class Person {
firstName: string;
lastName: string;
constructor(firstName: string, lastName: string) {
this.firstName = firstName;
this.lastName = lastName;
}
sayHello() {
console.log(`Hello, my name is ${this.firstName} ${this.lastName}.`);
}
}
const person: Person = new Person('John', 'Doe');
The Person class is defined in this instance as usual, with a constructor and a method. Then, a new Person object is created and given a person variable. The person variable’s type is determined by the Person class type, ensuring that it can only contain Person objects.
Overall, this method of using the class type prevents type mistakes and guarantees type safety in your code.
Can I use TypeScript class in JavaScript?
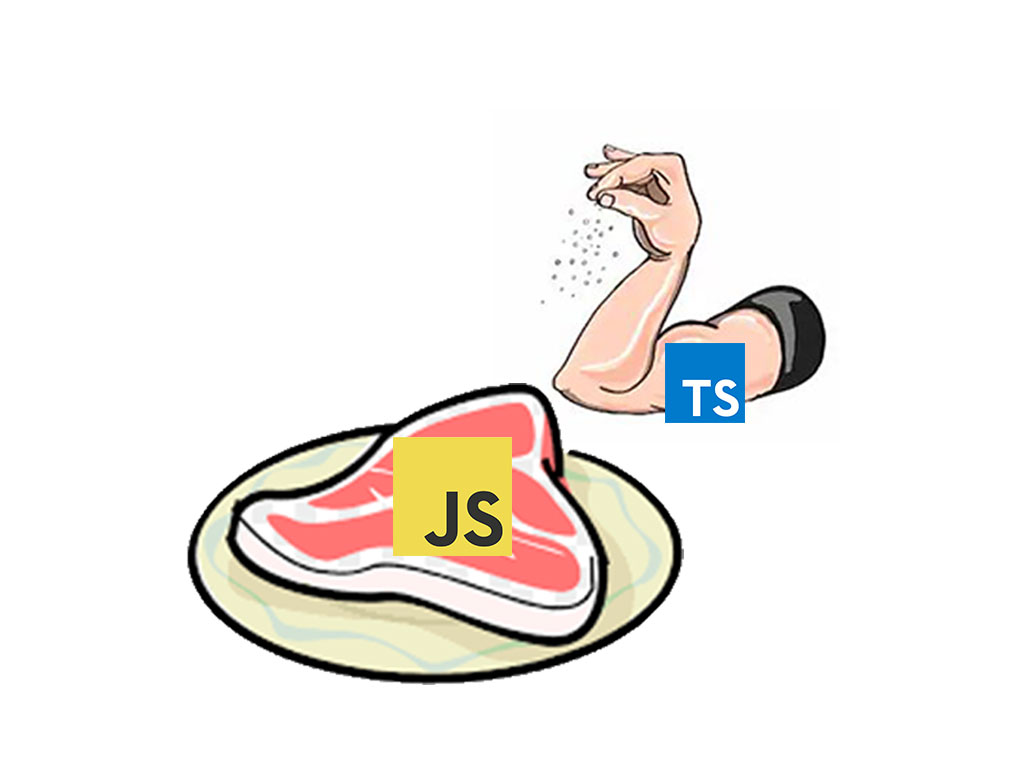
A TypeScript class may be used in JavaScript, but you must first translate your TypeScript code into JavaScript. Because TypeScript is a superset of JavaScript, it expands upon and gives the latter extra functionality. However, TypeScript code must be transpiled (translated) into JavaScript before it can be run in a JavaScript environment since it is not directly compatible with JavaScript.
To utilize a TypeScript class in a JavaScript environment, your TypeScript code must first be transpiled into JavaScript. Your code is automatically transpiled using a build tool like webpack or gulp, or using the TypeScript compiler (tsc).
You may utilize the resultant JavaScript code in a JavaScript environment just like any other JavaScript code once your TypeScript code has been transpiled into JavaScript. Please be aware, though, that the transpiled code could not be 100% identical to the original TypeScript code and might not have all of the features and capabilities of the TypeScript class.
In conclusion, even though TypeScript classes cannot be used directly in JavaScript, you may use the TypeScript compiler to translate your TypeScript code into JavaScript and then utilize the generated JavaScript code in a JavaScript environment.
Class inheritance in TypeScript
Classes in TypeScript are able to take on members from base classes. The functionality of the parent class may be reused and extended by the child classes thanks to the ability to build a parent class with properties and methods that can be inherited.
Here is an illustration of a basic class named Animal, which just includes the field “name” and the function “speak()”:
class Animal {
name: string;
constructor(name: string) {
this.name = name;
}
speak() {
console.log(`${this.name} makes a sound.`);
}
}
Using the extends keyword, you can construct a child class that derives from Animal as seen here:
class Cat extends Animal {
constructor(name: string) {
super(name);
}
}
The name attribute and the talk() function are inherited by the Cat class from the Animal class. Using the super keyword, which is used to call the constructor function of the parent class and supply any required parameters, the constructor() method of the Cat class also calls the constructor() method of the Animal class.
Then, after making an instance of the Cat class, you can use its talk() function to output the string “makes a sound” and the cat’s name, as follows:
const cat = new Cat("Tiger");
cat.speak(); // Output: Tiger makes a sound.
Class inheritance in TypeScript enables you to build more specialized classes with access to their parent class’s properties and methods while also giving you the option to add your own custom properties and methods. You may be able to design apps that are more well-organized and manageable as a result and make it simpler to reuse and expand your code.
The class keyword has also been a topic of discussion in this article, it is important to note that, in typescript, an abstract class may be defined using the abstract keyword, which is also primarily used for inheritance so that other classes can derive from it.
Access Modifiers
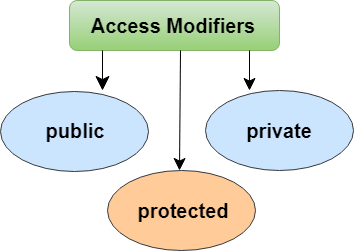
You may manage the visibility and accessibility of class members in TypeScript by using access modifiers as keywords. In TypeScript, there are three types of access modifiers: public, private, and protected.
- Public: Members of a class have access to their members by default. Anywhere outside the class, including instances of the class and derived classes, can access members declared public.
- Private: members may only be accessed from the class in which they are declared. A compile-time error will appear if an outsider tries to access a private member of the class.
- Protected: only the class in which they are declared or a class that is descended from it may access members that are designated as protected. A compile-time error will appear if you try to access a protected member from outside the class or from a non-derived class.
Here is an illustration of how these access modifiers may be used in a TypeScript class:
class BankAccount {
private balance: number;
constructor(initialBalance: number) {
this.balance = initialBalance;
}
public deposit(amount: number) {
this.balance += amount;
}
public withdraw(amount: number) {
if (this.balance - amount >= 0) {
this.balance -= amount;
} else {
console.log("Insufficient funds");
}
}
protected getBalance() {
return this.balance;
}
}
The balance attribute in this example is designated as private, making it only accessible from within the BankAccount class. The deposit and withdraw methods can be invoked on instances of the BankAccount class since they are declared as public. Because the getBalance function is protected, only instances of the BankAccount class or related classes may access it.
Creating an Object of Class
Generally, we would agree that a data structure that has data fields and methods that work with those fields is called an object. A class, which serves as a model or template for building objects, is an instance of an object.
In TypeScript, you must define a class and all of its members before creating objects of that class. For instance:
class BankAccount {
private balance: number;
constructor(initialBalance: number) {
this.balance = initialBalance;
}
public deposit(amount: number) {
this.balance += amount;
}
public withdraw(amount: number) {
if (this.balance - amount >= 0) {
this.balance -= amount;
} else {
console.log("Insufficient funds");
}
}
protected getBalance() {
return this.balance;
}
}
Using the new keyword, the class name, and any constructor parameters, if any, you may create an object of a class after it has been declared. For instance:
// Create an object of the BankAccount class with an initial balance of 1000
let myAccount = new BankAccount(1000);
After that, you may use the dot notation to invoke the class’s methods on the object. For instance:
// Deposit 500 into the account
myAccount.deposit(500);
// Withdraw 200 from the account
myAccount.withdraw(200);
// Print the current balance
console.log(myAccount.getBalance()); // 1300
If you take note, you would have seen that the getBalance function in this example is protected, therefore it can only be accessed from the BankAccount class or a derived class because of this. Consequently, a compile-time error will be returned if you attempt to invoke the getBalance function directly on the myAccount object.
Using Classes as Types
Similar to other programming languages, TypeScript allows you to utilize classes as types. To do this, you must first establish a class, after which you may declare a variable, provide the type of a function parameter, or indicate the type of a return value using the class name.
Here is an illustration of how to create a TypeScript class called Person and use it as the type for a variable:
class Person {
name: string;
age: number;
constructor(name: string, age: number) {
this.name = name;
this.age = age;
}
}
let person1: Person;
Name and age are the two attributes of the Person class in this example. When a new Person object is created, its constructor is used to initialize these attributes. Since the person1 variable is of type Person, any object that is an instance of the Person class may be assigned to it.
Classes are a type in TypeScript function signatures as well. Here is an illustration of how to use the Person class as a function’s return type:
class Person {
name: string;
age: number;
constructor(name: string, age: number) {
this.name = name;
this.age = age;
}
}
function getPerson(): Person {
let person = new Person('John Doe', 30);
return person;
}
Getters and Setters Methods in TypeScript
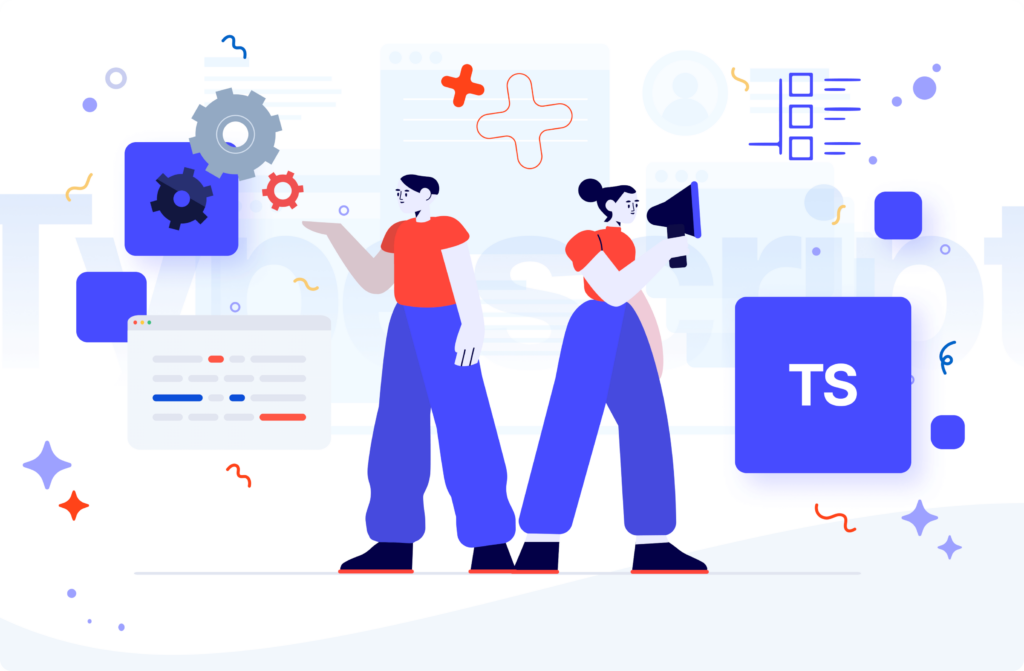
The values of an object’s properties are created and modified in TypeScript using getter and setter methods. While setters are used to change a property’s value, getters are used to retrieve the property’s original value.
You could, for instance, construct a setter method named “setName” that allows you to change the value of an object’s “name” property and a getter method called “getName” that retrieves the value of the “name” field. That would seem like this in TypeScript:
class MyClass {
private _name: string;
// Getter method
public getName(): string {
return this._name;
}
// Setter method
public setName(name: string): void {
this._name = name;
}
}
The getName() and setName() methods are used to access and change the value of the name property. To use the getter and setter methods, you must first create an instance of the MyClass class.
let myObj = new MyClass();
// Use the getter method to get the value of the name property
console.log(myObj.getName()); // Output: undefined
// Use the setter method to set the value of the name property
myObj.setName("John Doe");
// Use the getter method to get the updated value of the name property
console.log(myObj.getName()); // Output: "John Doe"
Getters and setters can help you impose limitations on the values of an object’s properties in addition to helping you write more understandable and legible code. For instance, you could use a getter method to calculate something depending on the value of a property or a setter function to guarantee that a property is always set to a legitimate value.
Defining Class properties in Constructors
Similar to JavaScript, TypeScript has a constructor-based method for creating class properties. The `this` keyword is used to refer to the current object when defining a property in a constructor. This is followed by the property name, the property type, and the value you wish to assign to the property.
Here is an illustration of a TypeScript constructor that defines a class property:
class MyClass {
constructor() {
this.myProperty: string = "some initial value";
}
}
In this example, a class called MyClass with a single property named myProperty has been defined. We refer to the current object in the constructor method using the keyword this, and we then give the myProperty property an initial value of “some starting value.”
Keep in mind that we also use a type annotation in TypeScript to describe the property’s type. We have said that the myProperty property is a string in this instance. As a result, the TypeScript compiler may check for type problems and give the class type information.
The property must be declared as a component of the class, much like in JavaScript, before it can be initialized in the constructor. Because the myProperty field is not declared in the MyClass class, the following code, for instance, would not function:
class MyClass {
constructor() {
this.myProperty: string = "some initial value"; // this will throw an error
}
}
Rather, before you could initialize it in the constructor, you would need to specify the myProperty field in the class, like follows:
class MyClass {
myProperty: string; // define the property
constructor() {
this.myProperty = "some initial value"; // initialize the property
}
}
The myProperty property may now be initialized in the class constructor since it is now a class member of the MyClass class. The TypeScript compiler will also be able to offer type information and check for type problems because we have declared the type of the property using a type annotation.
Conclusion
Finally, Typescript classes offer a strong and adaptable mechanism for integrating object-oriented programming ideas into your applications. Typescript classes can assist you in writing more scalable and maintainable code because of their robust type checking, support for inheritance, interfaces, and other sophisticated features.
However, this article has gone over and through everything, you need to know about typescript class, its applications, and implementations. This means you can be able to work with typescript classes confidently now.
Do you know that our Copycat plugin tool enables you to create working React applications from your Figma designs while saving you 35% on development costs? More intriguing articles from our blog are available at Copycat click here.
If you would also love to learn deeper about typescript classes, you can check out the video resource below;