- Introduction
- What is typeof operator in TypeScript?
- JavaScript typeof vs TypeScript typeof operator?
- How to use the typeof Operator in TypeScript?
- Getting the type of the object in TypeScript.
- Getting the type of the class object in TypeScript.
- Getting the type of the function object in TypeScript.
- Wrapping Up
Introduction
TypeScript is a superset of JavaScript that provides more strict type-checking functionality than JavaScript. TypeScript, like JavaScript, has an inbuilt typeof operator; we’ll look at the operator in TypeScript and how it works differently.
What is typeof operator in TypeScript?
The typeof keyword is a type-checking operator in TypeScript and JavaScript. Typescript typeof returns the data type of the operand passed to it.
The typeof syntax can be seen below:
typeof x // where x is the operand
The typeof operator can be written in both TypeScript and JavaScript as follows:
const myName = "Samuel"
console.log(typeof myName) // Output: string
The above expression will return string when executed:
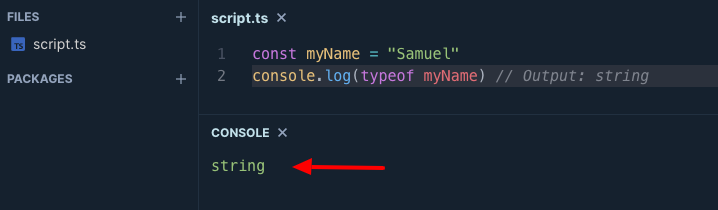
I’ll be making use of the playcode.io TypeScript playground throughout this article:
JavaScript typeof vs TypeScript typeof operator?
The typeof operator in TypeScript serves the same purpose as the operator in JavaScript, but with more features.
Learn more about the JavaScript typeof operator in the video below:
We’ll go over how to use the typeof operator in TypeScript (similar to JavaScript) and look at its additional features (Unique to TypeScript).
How to use the typeof Operator in TypeScript?
The typeof syntax in TypeScript is somewhat similar to that of JavaScript when type-checking data.
In TypeScript, typeof is helpful in two ways.
1. For type-checking data
console.log(typeof data)
Where variable data can be of any data type such as number, string, boolean, object, or array.
console.log(typeof 1) // number
console.log(typeof "hello, world") // string
console.log(typeof true) // boolean
console.log(typeof {}) // object
console.log(typeof []) // object
console.log(typeof undefined) // undefined
2. For extending the typeof of variables to create a custom type
Given that we have a variable called firstName with a string type, as shown below:
let firstName: string = "John"
console.log(typeof firstName)
We can extend the data type of firstName to another variable like lastName using the typeof operator:
let lastName: typeof firstName = "Doe"
console.log(typeof lastName)
When we execute the two expressions above, the data type of each variable will be displayed in the console.

This typeof will also ensure that the value assigned to lastName variable is the same as the firstName.
TypeScript will now throw an error when we assign a different data type to the lastName variable.
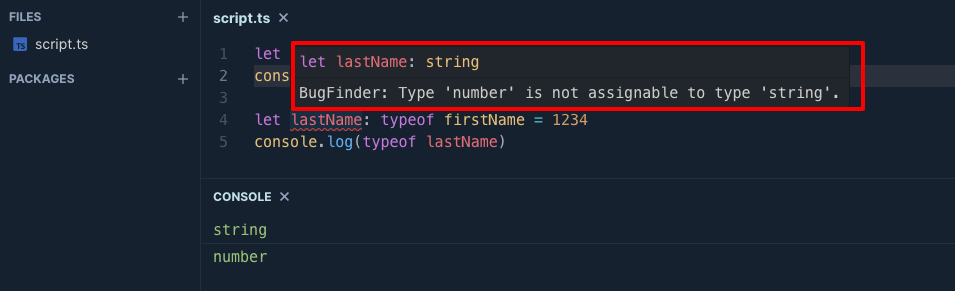
Getting the type of the object in TypeScript.
The typeof operator also works with objects and it can be used to determine if an item is a type of object or not.
const objectType = {}
console.log(typeof objectType) // Output object
When you execute the code above, the object type will be displayed on the console.
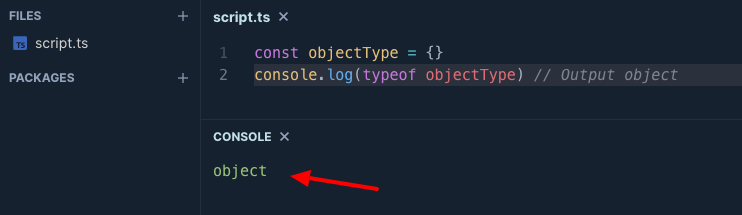
Typeof in TypeScript can also be used to create a custom type of object from variables.
Given that we have the following variables with different data types:
let firstName = "John" // string
let lastName = "Doe" // string
let course = "Computer Science" // string
let id = 33244 // number
let has_graduated = false // boolean
We can make use of the typeof operator to create a custom type of Student object whose properties should match the existing variables data types.
type Student = {
student_firstName: typeof firstName,
student_lastName: typeof lastName,
student_course: typeof course,
student_id: typeof id
has_graduated: typeof has_graduated
}
Getting the type of the class object in TypeScript.
Classes are templates for generating objects in JavaScript and are a type of “function”. We can make use of the typeof operator to determine if an item is a class or not.
class classType {}
console.log(typeof classType) // Output: "function"
The typeof class type will return a “function”, as shown below:
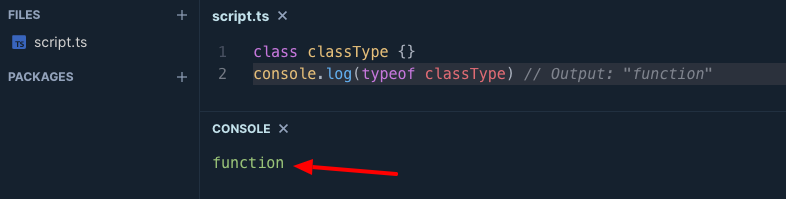
For example, if we have a class of Animals with the following structure:
class Animal {
name: string
height: number
constructor(name: string, height: number){
this.name = name
this.height = height
}
}
We can make use of typeof to create a factory function for creating new Animals objects:
function animalFactory(constructor: typeof Animal, name: string, height: number){
return new constructor(name, height)
}
The animalFactory function accepts 3 parameters, a constructor, the name, and the height of the new animal, like this:
const Dog = newAnimalFactory(Animal, "Dog", 2)
Now we can have a new dog object and display the output to the console:
console.log(Dog) // Output: {name: "Dog", height: 2}
The complete code will produce the output below:
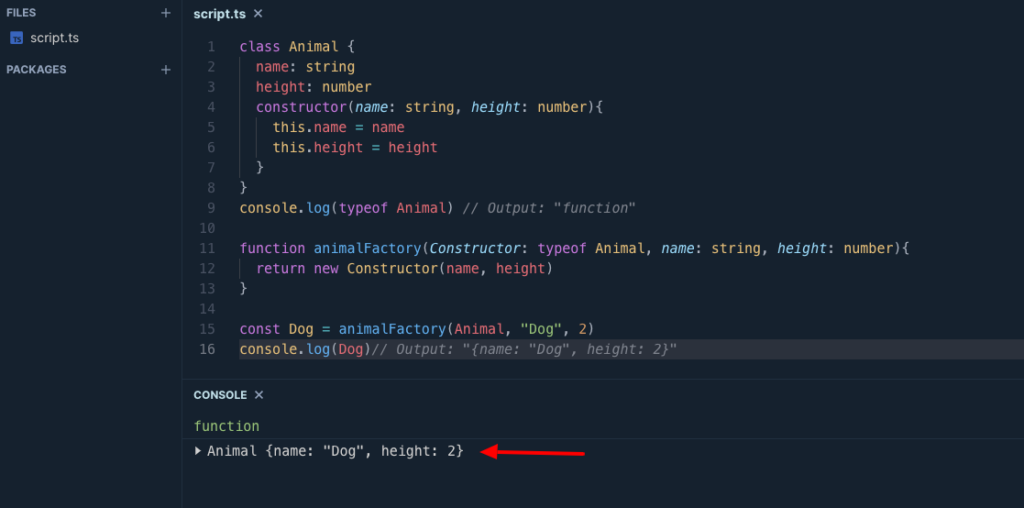
Getting the type of the function object in TypeScript.
Functions are an important concept in JavaScript, and we can use typeof to type-check a function as well.
function functionType(){}
console.log(typeof functionType) // Output: "function"
The output of the expression above will be “function” as shown in the image below:
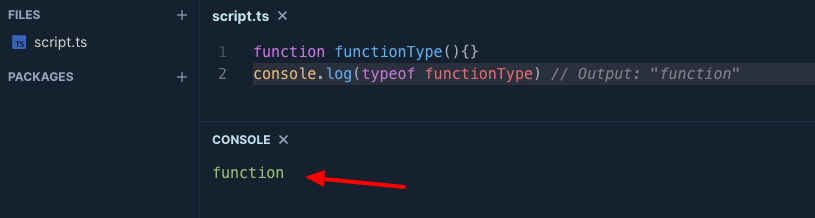
Apart from checking the type of function, the typeof operator is more useful in a function when combined with the ReturnType operator a Type which let us know the return type of a function.
Given that we have a function that converts strings to uppercase, it is ideal that the only valid data type that should be passed to the function is a string as declared below:
function stringToUpperCase (text: string){
return text.toUpperCase()
}
We can then pass a string to the function, like this:
console.log(stringToUpperCase("name")) // Output: "NAME"
The function will convert the passed string to an uppercase version as shown in the image below:
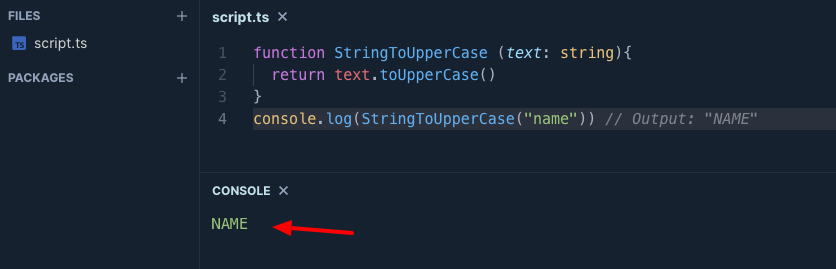
By combining the typeof and ReturnType we can define a custom type to a variable to only accept a data type that matches the return type of a specific function type, like this:
type funcTextType = ReturnType<typeof stringToUpperCase>
We’ll get an error message when we try to assign a different data type from the return type of the stringToUpperCase function:
let surName: funcTextType = 69
Since 69 is not a valid type of the stringToUpperCase function, TypeScript will throw an error within our code:
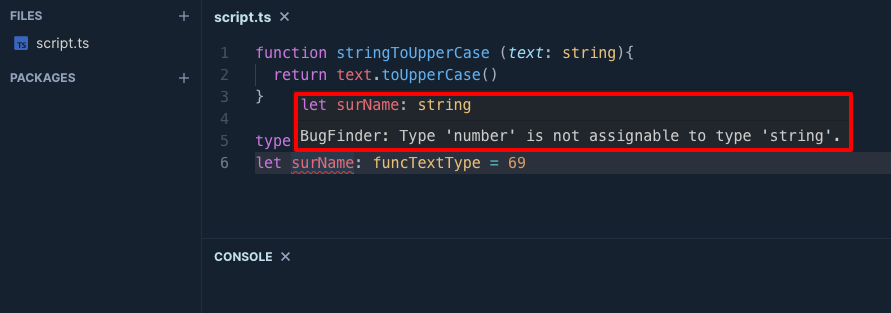
We recommend the video below if you want to learn more about TypeScript.
Wrapping Up
In this article, we’ve learned about the typeof operator in TypeScript and how it functions differently from the operator in JavaScript.
Finally, you can find more awesome articles like this on our blog at CopyCat. CopyCat is a tool that saves you about 35% of development time by converting your Figma files to an up-and-running React project. Check it out here.
Interesting Reads From Our Blogs
- All You Need to Know About TypeScript Types
- What to Read When Using Typescript In Your React App
- 10 CSS Frameworks for Frontend Developers in 2022
- Your Introductory Guide to React Intl
- The Top 16 Design System Examples
Check out the CopyCat plugin for React if you’re seeking amazing React tools to help you build component code faster and be production-ready than your competition!
Happy Coding!