- Introduction
- What is an enum in TypeScript?
- Should you use enums in TypeScript?
- How to Assign Enum Value in TypeScript
- Best Practices Using Typescript Enums
- How to use string enum in TypeScript?
- Why Use String Enums Over Regular Enums?
- Why Use Enums Instead of Strings?
- What is the difference between enum and object in TypeScript?
- Closing Remarks
Introduction
Are you tired of writing code that’s difficult to read and maintain? Do you struggle to keep track of the values and options used throughout your web applications? If you’re nodding your head in agreement, you’re in the right place. Meet TypeScript enum – a powerful tool for creating readable, maintainable, and error-free code. Using enums, you can easily assign names to sets of related values and refer to them throughout your code.
In this article, we’ll take you on a journey about TypeScript enums by covering everything you need to know about them. We’ll address some of the most common questions, including what an enum is, how to use and assign enum values when to use string enums, why enums are better than strings, and the differences between an enum and an object. So, if you’re ready to supercharge your TypeScript coding skills and write code that’s a breeze to read and maintain, let’s dive in!
What is an enum in TypeScript?

TypeScript enums are a powerful feature that allows you to define a set of named constants. In other words, an enum is a data type that lets you assign names to sets of related values, providing a way to associate names with values, making your code more readable and maintainable. Enums in TypeScript are a bit different from enums in other programming languages. For example, TypeScript enums and enums in C# are similar in many ways. Both data types allow you to define a set of named constants that represent related values. However, there are some key differences between the two.
Differences between Typescript Enum and C# Enums
First, enums in TypeScript are essentially a set of named constants that have corresponding numeric values. These numeric values can be auto-assigned, or you can specify them explicitly. This means that you can use enums in place of hard-coded numeric values throughout your code. On the other hand, enums in C# can have arbitrary values of any underlying integral type (such as int or byte), and they cannot be implicitly converted to other types.
For instance, you can use an enum to assign a name to a set of related options, such as the days of the week or the different statuses of an order. You can also use an enum to define the different states of a component, such as a loading spinner or a button. Similarly, you can use an enum to define the different types of users, such as admins, editors, and viewers.
Second, enums in TypeScript are structurally typed. This means that two enum types with the same members are considered equal, regardless of where they were defined. In C#, however, enum types are distinct, and cannot be compared for equality with other enum types, even if they have the same members and values.
Finally, enums in TypeScript can be defined using string values instead of numeric values. This is called a “string enum”, and it allows you to assign names to values that would otherwise be hard-coded strings. This feature is not available in C#.
To summarize, TypeScript enums and C# enums share some similarities, but differ in their handling of underlying values, their structural typing, and the ability to use string values.
The benefits of using TypeScript enum
There are many benefits of using Typescript enum:
First, they make your code more readable by giving names to otherwise arbitrary numeric values.
Second, they help prevent bugs and errors in your code by providing type safety and making it easier to catch mistakes.
Third, they make your code more maintainable by providing a central place to update values or options.
Finally, they improve code organization by grouping related options together under a single name.
Should you use enums in TypeScript?
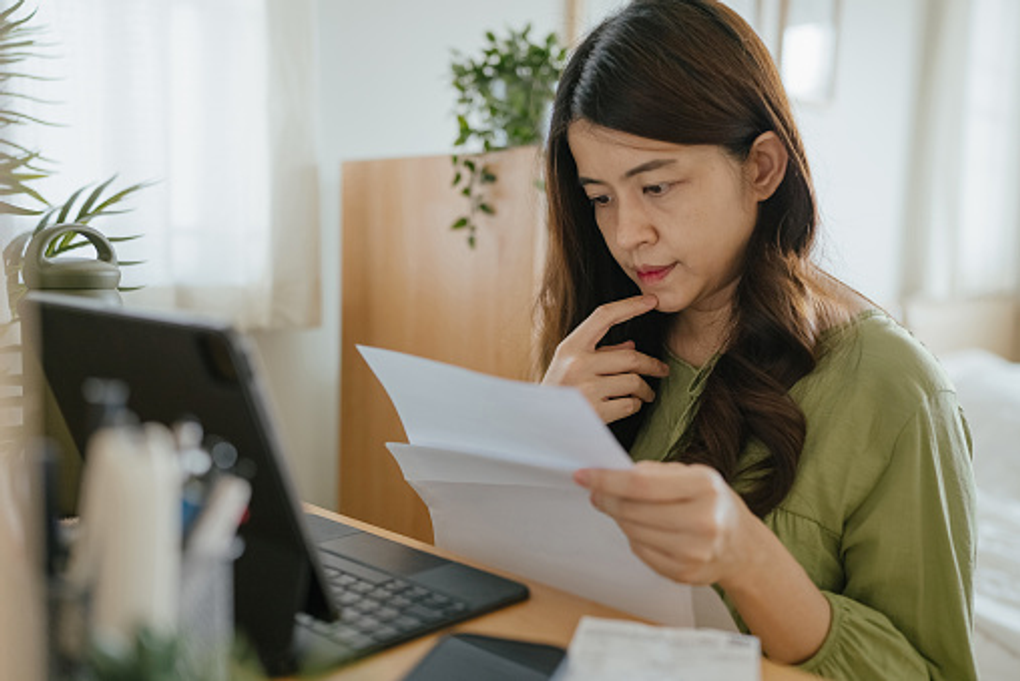
Enums can be a powerful tool in TypeScript, but they’re not always the right choice. While they can provide many benefits to your code, including improved readability, type safety, maintainability, and organization, they can also add unnecessary complexity and overhead to your program. Here are some factors to consider when deciding whether to use enums:
Advantages of using TypeScript enum:
- Make your code more readable by giving names to otherwise arbitrary numeric values.
- Provide type safety and make it easier to catch mistakes.
- Make your code more maintainable by providing a central place to update values or options. This is particularly useful when the set of options or values is expected to change over time, since it avoids having to update multiple places in your code.
- Improve code organization by grouping related options together under a single name. This makes your code more modular and easier to understand.
- Enums are self-documenting, meaning that they make it easier for other developers (or even your future self) to understand the purpose and meaning of the options or values in your code.
- Can help to prevent hard-to-debug errors that can occur when using magic numbers or string literals in your code. By using enums, you can avoid typos, case-sensitivity issues, or other mistakes that can arise when using raw strings or numbers.
- Improve code maintainability and readability by allowing you to define specific subsets of values or options. For example, you can define a specific subset of options that apply only to a certain part of your code, and then use that subset consistently throughout your program.
- Enums can make your code more concise and less error-prone by reducing the need for repetitive and verbose if/else or switch statements that would otherwise be required to check multiple related options or values.
Disadvantages of using enums in TypeScript:
- Can add complexity to your code, especially when dealing with large or complicated sets of options or values. This can make your code harder to understand and maintain.
- Enums can be overkill for simple sets of options or values, and may add unnecessary overhead to your program. For example, if you only have two or three options to choose from, an enum may be more trouble than it’s worth.
- Enums can be inflexible, and may not be able to handle all use cases. For example, enums are inherently static, meaning that they cannot be changed at runtime. This can be a limitation in certain situations, especially when dealing with dynamic or unpredictable data.
- Less performant than other data types, especially when dealing with large or complex sets of options or values. This is because each option or value in an enum is essentially a separate instance of an object, which can be slower to create and access than other data types.
- Less portable than other data types, especially when dealing with cross-platform or cross-language development. This is because enums are specific to TypeScript, and may not be supported or easily translated into other languages or platforms.
- Enums can add unnecessary restrictions to your code, and may not be appropriate for all programming styles or paradigms. For example, if you prefer functional programming or declarative programming, enums may not fit well with your coding style.
Here’s a great video arguing against enums:
When to use and not use enums in TypeScript:

How to Assign Enum Value in TypeScript
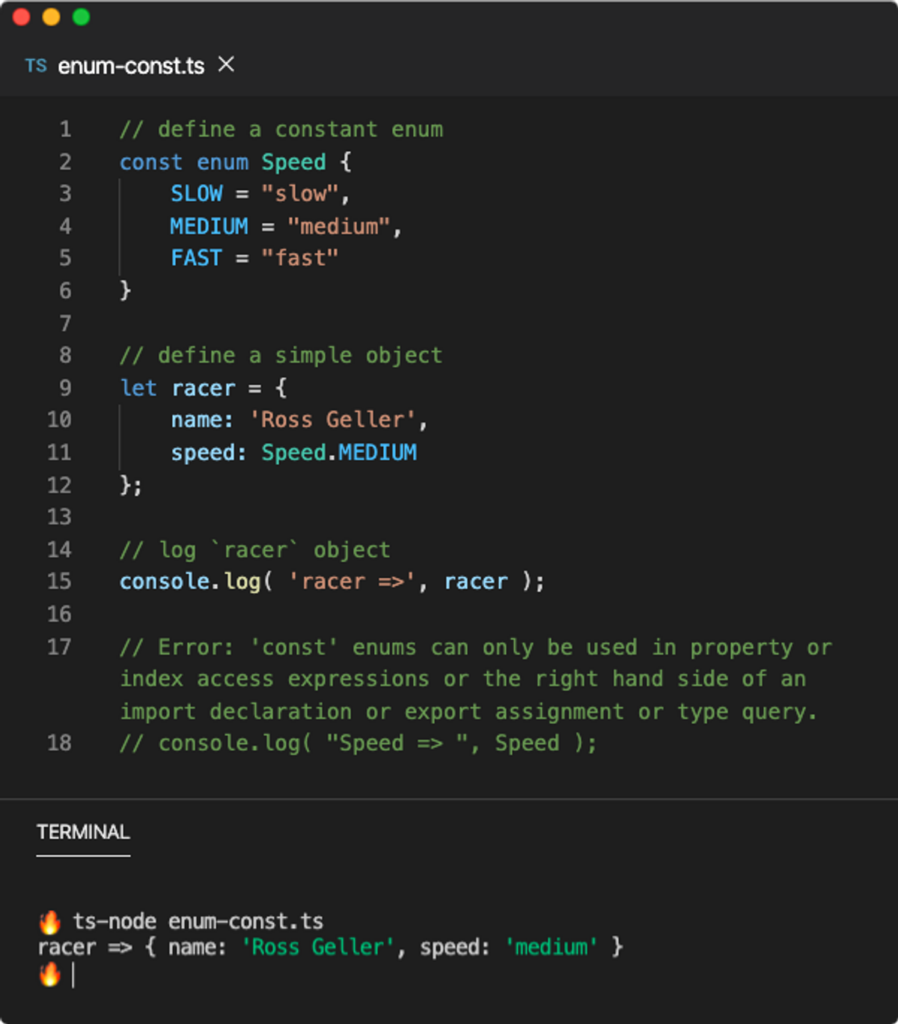
Enums in TypeScript are created using the enum
keyword, which is followed by the name of the enum and a list of its values in braces. Each value is assigned a number by default, starting with 0 for the first value, 1 for the second value, and so on. However, you can also assign specific values to each enum value using the =
operator.
Here is an example of how to create an enum in TypeScript:
enum Color {
Red,
Green,
Blue
}
In this example, the Color
enum has three values: Red
, Green
, and Blue
. By default, Red
has the value 0
, Green
has the value 1
, and Blue
has the value 2
.
To assign specific values to enum values, you can use the =
operator, as in the following example:
enum Direction {
Up = 1,
Down,
Left,
Right
}
In this example, the Direction
enum has four values: Up
, Down
, Left
, and Right
. The Up
value has the specific value 1
, and the subsequent values are assigned values of 2
, 3
, and 4
, respectively.
To assign an enum value to a variable, you can reference the enum name and the value you want to assign, as in the following example:
let myColor: Color = Color.Red;
In this example, the myColor
variable is assigned the value Red
from the Color
enum.
When naming and organizing enums, it’s important to choose descriptive and intuitive names that accurately reflect the values they represent. Here are some best practices to use when using enums:
Best Practices Using Typescript Enums

Use singular naming:
When naming enums, use singular names for the enum type rather than plural names. For example, use Direction
rather than Directions
. This makes the enum easier to read and understand.
Use uppercase naming convention:
Use uppercase naming convention for the enum values to distinguish them from other variables and constants in your code. For example, use RED
instead of red
.
Use descriptive names:
Use descriptive names for enum values to make it clear what they represent. Avoid using single letters or short abbreviations that may not be immediately obvious to others reading your code.
Use consistent naming:
Be consistent with the naming convention you choose for enums and their values. This makes it easier to read and understand your code, and reduces the risk of errors.
Use enum for fixed options:
Use enums when you have a fixed set of options or values that are unlikely to change. This can make your code more readable and maintainable.
Avoid overusing enums:
Avoid using enums for values that may change frequently or for values that are not clearly defined or well understood. Overusing enums can make your code more complex and harder to maintain.
How to use string enum in TypeScript?
A string enum in TypeScript is a type of enum where the values are initialized with string literals instead of numeric literals. Like regular enums, string enums are used to represent a set of related values in a type-safe way. However, string enums provide several benefits over regular enums. They offer more descriptive values that are easier to read and understand, and they can be extended with additional values without breaking existing code. Additionally, string enums can be used as keys in objects, which can be useful in certain scenarios. Overall, string enums offer greater flexibility and more readable code than regular enums.
Here’s an example of how to create and use a string enum in TypeScript:
enum Direction {
Up = "UP",
Down = "DOWN",
Left = "LEFT",
Right = "RIGHT"
}
function move(direction: Direction) {
// ...
}
move(Direction.Up);
In this example, we define a Direction
string enum with four values, each initialized with a string literal. We then use the move
function to move in a certain direction based on the enum value passed to it.
Using string enums has several benefits over regular enums.
Why Use String Enums Over Regular Enums?
There are several benefits of using string enums over regular enums in TypeScript. One major advantage is that string enums provide more descriptive values that are easier to read and understand. With regular enums, the values are typically represented as numeric literals, which can be more difficult to interpret. On the other hand, string enums use string literals to represent values, which can be much more descriptive and meaningful.
Another advantage of string enums is that they can be extended with additional values without breaking existing code. With regular enums, once the enum is defined, the set of values is fixed and cannot be changed without potentially breaking existing code. However, with string enums, new values can be added to the set without affecting existing code that uses the enum. This makes string enums more flexible and adaptable than regular enums.
Finally, string enums can also be used as keys in objects, which can be useful in certain scenarios. For example, if you have an object that stores data for different types of vehicles, you could use a string enum as the key to represent the type of vehicle, rather than using a string literal. This makes the code more readable and maintainable, and also provides type-safety by ensuring that the key can only have certain values. Overall, using string enums in TypeScript can lead to more flexible, readable, and maintainable code.
Regular Enum vs String Enum Table
Type | Advantage | Disadvantage | When to Use |
Regular Enum | Type-safety and more concise than using string literals | Numeric values can be less descriptive and harder to interpret | Use when working with a set of related numeric values that represent a single concept |
String Enum | More descriptive values that are easier to read and understand | Numeric values can be less descriptive and harder to interpret | Use when working with a set of related string values that represent a single concept, or when you need to use the enum as a key in an object |
Note that these are general guidelines, and there may be situations where either type of enum is appropriate. The key is to choose the type that best represents the data you’re working with and makes the code easier to read and understand.
Why Use Enums Instead of Strings?
Now, time to argue the other side. While it’s possible to use strings to represent a set of related values, using enums in TypeScript can provide several advantages in some instances. One major advantage of enums is type safety since TypeScript can enforce that only valid enum values are used, whereas strings are more prone to typos and other errors. In addition, enums can be more readable and expressive than strings since they allow you to give descriptive names to a set of related values, making your code easier to understand and maintain.
For example, imagine you’re working on a project that involves colors. You could represent the colors using strings, like this:
const RED = "red";
const GREEN = "green";
const BLUE = "blue";
However, this approach has some downsides. For one, there’s nothing stopping you from accidentally using a string that’s not one of the valid colors, like “rad” instead of “red”. This can lead to bugs that are hard to catch and fix. In addition, it’s not immediately clear what the values represent, since they’re just arbitrary strings.
By contrast, using an enum can make the code more type-safe and readable:
enum Color {
Red = "red",
Green = "green",
Blue = "blue",
}
Now, TypeScript will ensure that only valid colors are used, and the values are self-documenting, since each color is given a clear, descriptive name. This can make your code more maintainable and less error-prone over time.
Of course, there are situations where using strings is appropriate, especially if the values you’re working with are not fixed or well-defined. However, in cases where you have a set of related, discrete values, using an enum can provide a number of benefits that make your code more robust and easier to understand.
What is the difference between enum and object in TypeScript?
TypeScript has both enums and objects, which can be used to group related values together. However, there are important differences between the two.
An enum in TypeScript is a set of named values that are assigned to a numeric type. Enums are useful for grouping related values together and providing a clear and concise way to reference those values. On the other hand, an object is a collection of key-value pairs where the keys are strings and the values can be of any type.
One key difference between enums and objects is that enums provide type safety, while objects do not. This means that with enums, the compiler can check that you are using a valid value, which can help catch errors before they occur. Additionally, enums are more readable than strings, since they provide a clear and concise way to reference values.
However, there are some scenarios where using an object can be more appropriate than using an enum. For example, if you have a large and complex set of values that do not fit neatly into a simple set of named values, an object may be a better choice. On the other hand, if you have a small and simple set of values that need to be referenced in a clear and concise way, an enum may be the best choice. Ultimately, the decision between an enum and an object depends on the specific requirements of your project.
Here’s a video on how to pick between the two:
Closing Remarks
Enums in TypeScript are powerful data types that can simplify code and improve readability. They provide a simple and concise definition of a set of related constants. This article has covered what enums are in TypeScript, when to use them, how to create and assign enum values, and the differences between string and regular enums. We also discussed the advantages of using enums over strings and provided some best practices for working with enums in TypeScript. Additionally, we explored the difference between an enum and an object in TypeScript and discussed some specific scenarios where it makes sense to use one over the other. Overall, enums are a valuable tool for any TypeScript project, and we encourage readers to use them to improve their code.