- Is there a map in TypeScript?
- What is a TypeScript Map
- What is the purpose of map ()?
- Creating a Typescript Map
- Different ways to initialize a TypeScript Map object
- Retrieving Values from a TypeScript Map
- Using the
get()
method to retrieve a value based on its key: - Using the
has()
method to check if a key exists in the TypeScript Map object: - Using the
keys()
andvalues()
methods to get an array of keys or values from the TypeScript Map object: - Using the
entries()
method to get an array of key-value pairs from the TypeScript Map object: - How should I retrieve my data?
- - Entries()
- - Keys()
- - Values()
- - get()
- - has()
- Using the
- Removing Values with TypeScript Map
- Iterating Over the Keys and Values of a TypeScript Map
- When to use map TypeScript? (With Example)
- How to create array of map in TypeScript?
- Closing Thoughts
Is there a map in TypeScript?
Have you ever needed to store key-value pairs in your web application? If so, you’ve likely encountered the JavaScript object as a standard solution. However, as your application grows, the limitations of the object can become apparent. That’s where TypeScript Map comes in! TypeScript Map is a built-in data structure that provides a more robust alternative to the traditional JavaScript object.
We’ll dive into the benefits of TypeScript Map, how to create and initialize a TypeScript Map, how to add, retrieve, and remove values, and different ways to iterate over the keys and values. By the end of this article, you will have a solid understanding of how to use map in TypeScript in your web development projects and how it can help you best manage and easily manipulate complex data structures. Let’s explore how TypeScript Map can improve your web development experience!
What is a TypeScript Map
In TypeScript, a Map object is a built-in class that provides a way to map keys to values. It’s similar to an object, but with a few key differences. Firstly, a TypeScript Map type allows any value to be used as a key, including objects and functions. Secondly, a TypeScript Map maintains the order in which the keys are inserted. Finally, TypeScript Map objects have built-in methods for adding, retrieving, and removing key-value pairs, which makes them much more convenient than regular objects for storing data. This makes it an essential tool for managing and manipulating complex data structures in web development. Creating a new TypeScript Map object is easy and straightforward, and you can initialize the object with any number of key-value pairs.
Is TypeScript map a Hashmap?
Yes, TypeScript Map is a type of Hashmap. A Hashmap is a data structure that allows fast access to values based on their associated keys. In TypeScript, Map is a built-in class that implements the Hashmap data structure. It allows developers to store key-value pairs, where each key is unique and associated with a specific value. While Map shares similarities with Hashmap, it also has some differences, such as allowing non-string keys and offering a variety of built-in methods for easy manipulation of key-value pairs.
Is it better to use map or HashMap?
It’s worth noting that although the two data structures have some similarities, they have different use cases and choosing the right one depends on the requirements of your specific use case.
Here is a table comparing the two:
TypeScript Map | Hashmap | |
---|---|---|
Data Structure | Key-value pairs | Key-value pairs |
Key Types | Any | Any |
Value Types | Any | Any |
Order | Maintains insertion order | No order guaranteed |
Methods | Built-in methods to add, remove, and retrieve data | Basic methods to add, remove, and retrieve data |
Performance | Slower for small datasets, faster for larger datasets | Faster for small datasets, slower for larger datasets |
Memory Usage | More memory used to maintain insertion order | Less memory used, no order maintained |
Use Cases | Ideal for storing large datasets with ordered data retrieval | Ideal for small to medium datasets where order is not important |
In general, if you need to store key-value pairs in a collection and require fast lookups and insertions, both Map
and HashMap
can be good options. Map
provides more built-in functionality, such as the ability to iterate over the keys and values, while HashMap
may offer slightly better performance in some cases.
However, HashMap
is not a built-in data structure in TypeScript or JavaScript, so it would need to be implemented separately or through a third-party library. Additionally, HashMap
may not be as widely supported as Map
, so it may be more difficult to find resources and support for it. Ultimately, the choice between Map
and HashMap
depends on the specific requirements of the project and the trade-offs between functionality and performance.
But before we get into implementing Typescript Map, we need to talk about map().
What is the purpose of map ()?
The map()
function is a method that is available on the TypeScript Array type. It allows you to transform an array by applying a given function to each element of the array. The map()
function returns a new array containing the results of the function applied to each element of the original array. In TypeScript, you can use the map()
function on an array of any type, including arrays of objects and arrays of TypeScript Map objects. You can read more on the official documentation for more clarity.
The syntax for using map()
is simple. You call the map()
method on the array you want to transform and pass in a callback function as an argument. The callback function takes one argument, which is the current element of the array being processed. The function should return a new value that will be added to the new array that is being created.
map () example
Here’s an example of using the map()
function to transform an array of numbers:
const numbers = [1, 2, 3, 4, 5];
const doubledNumbers = numbers.map((num) => num * 2);
console.log(doubledNumbers); // [2, 4, 6, 8, 10]
In this example, we first define an array of numbers. We then call the map()
function on this array and pass in a callback function that doubles each element of the array. The resulting array is stored in the doubledNumbers
variable and is logged to the console.
You can also use the map()
function to transform an array of objects. For example, suppose you have an array of user objects, each with a firstName
and lastName
property. You could use the map()
function to transform this array into an array of full names:
const users = [
{ firstName: 'John', lastName: 'Doe' },
{ firstName: 'Jane', lastName: 'Smith' },
{ firstName: 'Bob', lastName: 'Johnson' },
];
const fullNames = users.map((user) => `${user.firstName} ${user.lastName}`);
console.log(fullNames); // ["John Doe", "Jane Smith", "Bob Johnson"]
In this example, we call the map()
function on the users
array and pass in a callback function that returns a string consisting of the user’s first name and last name separated by a space. The resulting array is stored in the fullNames
variable and is logged to the console.
The map()
function is a powerful tool for transforming arrays in TypeScript. It can be used to simplify your code and make it more expressive. When used correctly, the map()
function can help you write clean, concise, and maintainable code.
Creating a Typescript Map
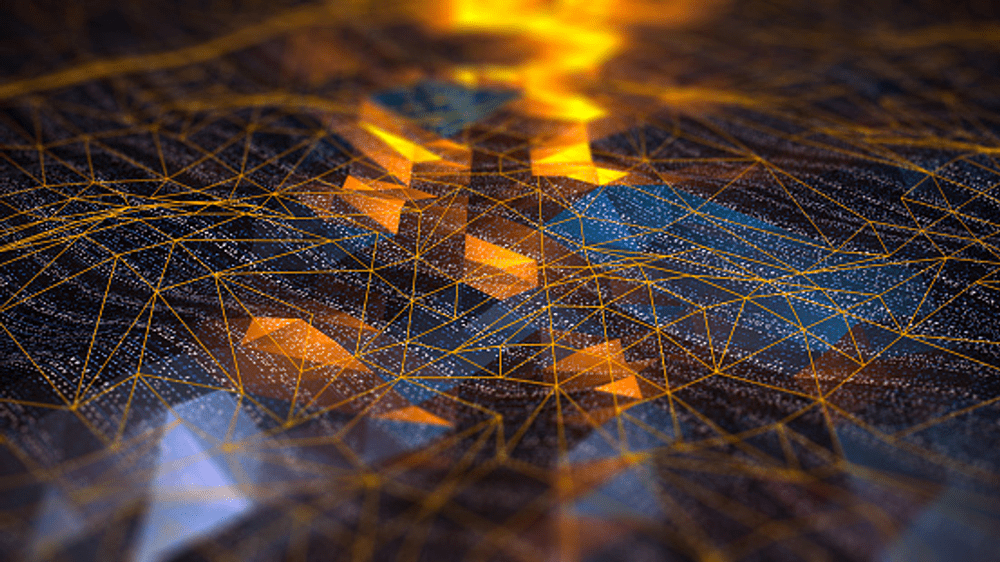
To create a TypeScript Map, you first need to define a new instance of the Map object. There are several ways to do this, including:
How do you use map () in TypeScript?
Using the new
keyword with the Map
constructor:
const myMap = new Map();
Using new Map()
to create a new Map
object can be beneficial if you need to create a map from scratch and have full control over the keys and values that will be added to the map. This method allows you to add and remove keys and values as needed, and gives you more flexibility and control over the map’s contents. Additionally, using new Map()
ensures that the resulting Map
object is a true instance of the Map
class, which may be important in some cases.
Creating a new Map from an existing object:
const myObject = { key1: 'value1', key2: 'value2', key3: 'value3' };
const myMap = new Map(Object.entries(myObject));
On the other hand, creating a new Map
from an existing object using the Map()
constructor and passing in the object as an argument can be beneficial if you already have an object with the desired keys and values, and you want to quickly and easily convert it into a Map
. This method allows you to add and remove keys and values as needed, giving you more flexibility and control over the Map’s contents. However, it is worth noting that the resulting Map
object will be a copy of the original object, rather than a true instance of the Map
class.
These are the most popular options to create a map, but check out this video to learn a third way:
Ultimately, the decision of which method to use should be based on your project’s specific needs and requirements. No matter your choice, you’ll end up with a new instance of a TypeScript Map that you can use to store and manipulate your data.
Different ways to initialize a TypeScript Map object
Initializing a TypeScript Map means defining and assigning an initial set of key-value pairs to the Map object when it’s created. This allows you to pre-populate the Map with some data at the outset, which can be helpful in many scenarios. There are various ways you can initialize a typescript map.
Array of Key-value pairs
You can initialize a TypeScript Map object with an array of key-value pairs, like this:
const myMap = new Map([
['key1', 'value1'],
['key2', 'value2']
]);
This will create a TypeScript Map object with two key-value pairs. Using the new
keyword with the Map
constructor and passing in an array of key-value pairs can be a more concise and readable way to initialize a Map, especially if you have a lot of data to add to the Map at once.
Array of values
Additionally, you can initialize a TypeScript Map object with an array of values and a function to generate keys shown below:
const myMap = new Map(
['value1', 'value2', 'value3'].map((value, index) => [index, value])
);
This will create a TypeScript Map object with three key-value pairs, where the keys are the array indices, and the values are the array values. This method can be helpful if you want to generate keys for the Map based on some logic or algorithm. By using a function to generate the keys, you can create more complex data structures tailored to your project’s needs.
Another Typescript Map object
Alternatively, you can initialize a TypeScript Map object with another TypeScript Map object:
const myMap1 = new Map([
['key1', 'value1'],
['key2', 'value2']
]);
const myMap2 = new Map(myMap1);
This will create a new TypeScript Map object, myMap2
, a copy of the first TypeScript Map object, myMap1
. This is useful if you want to copy an existing Map into a new Map or if you want to merge the key-value pairs from multiple Maps into a single Map. It works best for working with complex data structures and managing data across different application parts.
set() method
You can also create an empty Map and adding key-value pairs using the set()
method:
const myMap = new Map();
myMap.set('key1', 'value1');
myMap.set('key2', 'value2');
myMap.set('key3', 'value3');
Creating an empty Map and adding key-value pairs using the set()
method can be more flexible because you can add or remove key-value pairs at any time, not just at initialization. This can be helpful if you’re working with data that changes frequently or dynamically generating data to add to the Map.
Ultimately, how to create and initialize your TypeScript Map object will depend on your specific use case and personal preference. Understanding the various methods for creating and initializing TypeScript Maps will allow you to work with and manipulate complex data structures efficiently. The following section will delve into retrieving values with TypeScript Map to build more advanced functionality.
So, let’s move on to the next section.
Retrieving Values from a TypeScript Map
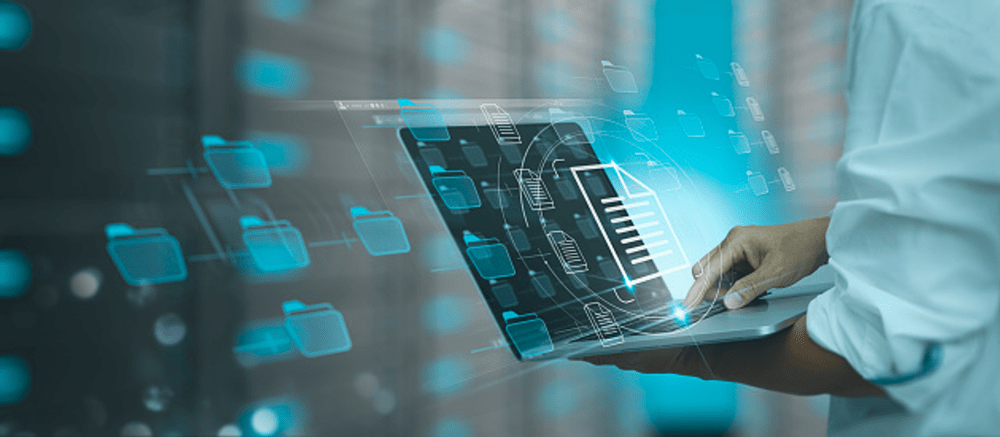
Retrieving values in a TypeScript Map refers to accessing the value associated with a specific key in the Map. This key feature makes it a useful data structure for managing and manipulating complex data, and this can be done using multiple methods.
Using the get()
method to retrieve a value based on its key:
You can retrieve a value from a TypeScript Map type using the get()
method. This method takes a key as an argument and returns the value associated with that key. Here’s an example:
let myMap = new Map();
myMap.set("key1", "value1");
myMap.set("key2", "value2");
let value = myMap.get("key1");
console.log(value); // output: "value1"
The get()
method returns the value associated with the key if it exists in the map, or undefined
if the key is not present.
Using the has()
method to check if a key exists in the TypeScript Map object:
You can check if a key exists in a TypeScript Map using the has()
method. This method takes a key as an argument and returns true
if the key exists, or false
otherwise. Here’s an example:
let myMap = new Map();
myMap.set("key1", "value1");
myMap.set("key2", "value2");
let hasKey = myMap.has("key1");
console.log(hasKey); // output: true
Using the keys()
and values()
methods to get an array of keys or values from the TypeScript Map object:
You can get an array of keys or values from a TypeScript Map function using the keys()
or values()
methods, respectively. Here’s an example:
let myMap = new Map();
myMap.set("key1", "value1");
myMap.set("key2", "value2");
let keys = Array.from(myMap.keys());
let values = Array.from(myMap.values());
console.log(keys); // output: ["key1", "key2"]
console.log(values); // output: ["value1", "value2"]
Using the entries()
method to get an array of key-value pairs from the TypeScript Map object:
You can also get an array of key-value pairs from a TypeScript Map function using the entries()
method. This method returns an iterator of key-value pairs. Here’s an example:
let myMap = new Map();
myMap.set("key1", "value1");
myMap.set("key2", "value2");
let entries = Array.from(myMap.entries());
console.log
How should I retrieve my data?
The best method for retrieving values from a TypeScript Map depends on the specific situation and the desired output. Here are some factors to consider when choosing a retrieval method:
– Entries()
If you need to get all the key-value pairs in a Map, use the entries()
method. This method returns an iterable that contains an array of [key, value]
pairs for each element in the Map. You can use the for...of
loop to iterate over the entries and access the keys and values:
const myMap = new Map([['foo', 1], ['bar', 2], ['baz', 3]]);
for (const [key, value] of myMap.entries()) {
console.log(key, value);
}
– Keys()
If you need to get all the keys in a Map, use the keys()
method. This method returns an iterable that contains all the keys in the Map. You can use the for...of
loop to iterate over the keys:
const myMap = new Map([['foo', 1], ['bar', 2], ['baz', 3]]);
for (const key of myMap.keys()) {
console.log(key);
}
– Values()
If you need to get all the values in a Map, use the values()
method. This method returns an iterable that contains all the values in the Map. You can use the for...of
loop to iterate over the values:
const myMap = new Map([['foo', 1], ['bar', 2], ['baz', 3]]);
for (const value of myMap.values()) {
console.log(value);
}
– get()
If you need to get a single value from a Map, use the get()
method. This method takes a key as an argument and returns the corresponding value, or undefined
if the key is not in the Map:
const myMap = new Map([['foo', 1], ['bar', 2], ['baz', 3]]);
const value = myMap.get('bar');
console.log(value);
– has()
If you need to check if a key is in a Map, use the has()
method. This method takes a key as an argument and returns true
if the key is in the Map, or false
if it is not:
const myMap = new Map([['foo', 1], ['bar', 2], ['baz', 3]]);
const hasKey = myMap.has('bar');
console.log(hasKey);
In general, it’s best to choose the method that provides the output you need with the least amount of code.
Removing Values with TypeScript Map
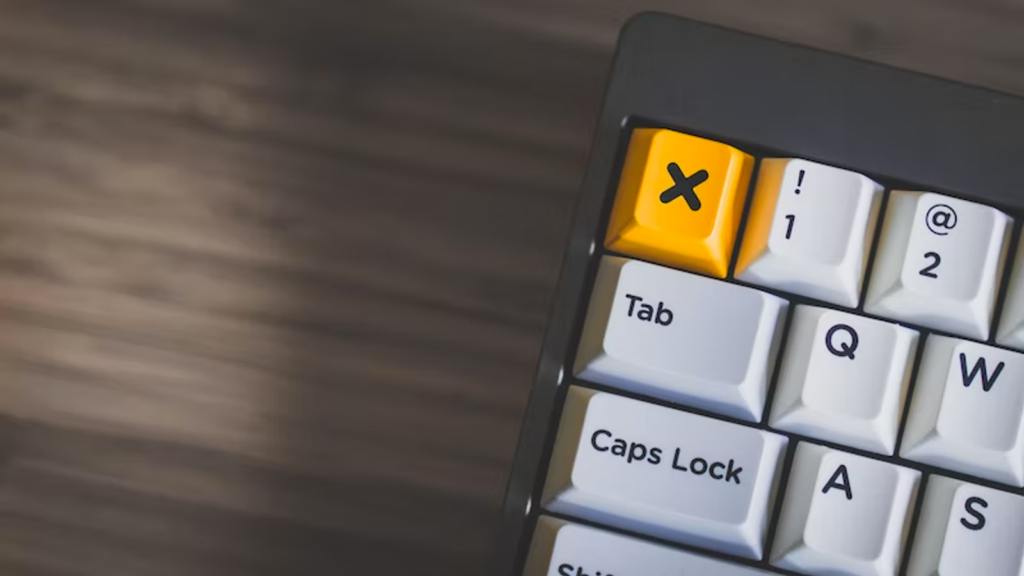
In addition to adding and retrieving values, it is also important to know how to remove values from a TypeScript Map to keep the map’s data accurate and up-to-date. As your application manipulates and manages data, you may need to remove specific entries from the map that are no longer required. Removing values that are no longer needed can free up memory and resources that can be used for other purposes. Additionally, suppose the values stored in the map are sensitive or confidential. In that case, removing them is important to ensure they do not remain on the map longer than necessary. There are several ways to remove key-value pairs from a TypeScript Map object:
delete() method:
This method accepts a key and removes the corresponding key-value pair from the map. For example, to remove the value associated with the key “foo”, you can use the following code:
myMap.delete("foo");
clear() method:
This method removes all key-value pairs from the map. For example, to remove all values from the map, you can use the following code:
myMap.clear();
It is important to note that both of these methods mutate the original map object.
To ensure that a key-value pair exists in a map before removing it, you can use the has() method to check whether the key exists in the map before attempting to delete it. For example, you can use the following code to remove a key-value pair only if it exists in the map:
if (myMap.has("foo")) {
myMap.delete("foo");
}
By using the methods outlined above, you can easily manage and manipulate data in a TypeScript Map object, adding and removing values as necessary.
In the next section, we will explore different ways to iterate over the keys and values in a TypeScript Map object.
Iterating Over the Keys and Values of a TypeScript Map
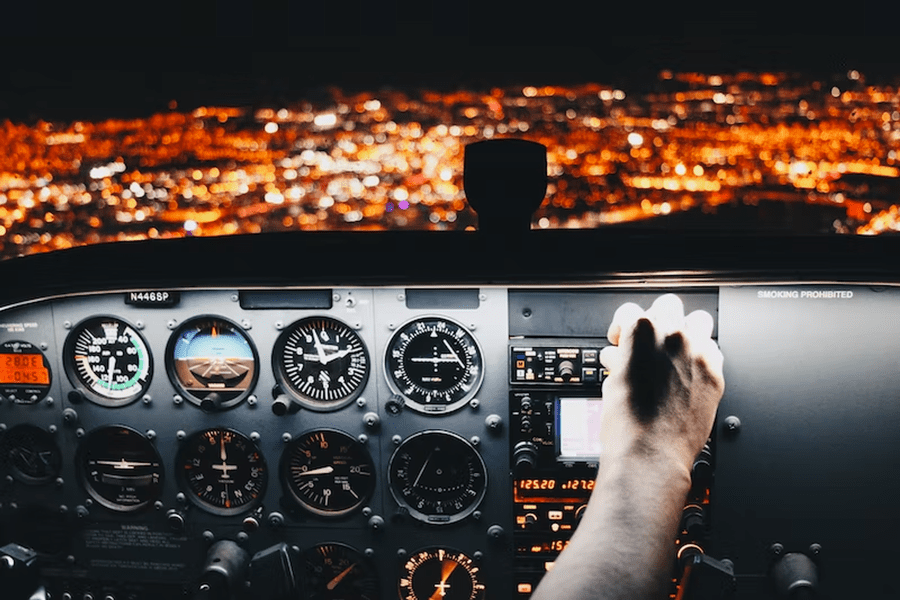
Iterating over the keys and values of a TypeScript Map refers to accessing each key-value pair stored in the Map and performing an operation on them. This process is typically used to perform operations such as filtering, sorting, or transforming the values of a Map. It enables you to access, manipulate and process the data stored on the Map. Using different iteration methods, you can achieve different results and perform various operations on the data. Here are four strategies to iterate over the keys and values of a TypeScript Map object:
Method 1: for…of loop
The for…of loop is a simple and easy way to iterate over the keys and values of a TypeScript Map object. In this method, we use the built-in entries()
method of a TypeScript Map object to get an iterator for the key-value pairs, and then use the for…of loop to loop over the pairs and extract the key and value. Here’s an example:
const myMap = new Map([
['key1', 'value1'],
['key2', 'value2'],
['key3', 'value3']
]);
for (const [key, value] of myMap.entries()) {
console.log(key, value);
}
In this example, the for...of
loop extracts the key
and value
from each key-value pair returned by myMap.entries()
, and logs them to the console.
Method 2: forEach() method
The forEach()
method is another way to iterate over the keys and values of a TypeScript Map object. In this method, we use the forEach()
method of a TypeScript Map object to loop over the key-value pairs and execute a callback function for each pair. Here’s an example:
const myMap = new Map([
['key1', 'value1'],
['key2', 'value2'],
['key3', 'value3']
]);
myMap.forEach((value, key) => {
console.log(key, value);
});
In this example, the forEach()
method executes the callback function for each key-value pair in myMap
, passing the value
and key
as arguments.
Method 3: Using keys() and values() methods
The keys()
and values()
methods of a TypeScript Map object can be used to get an iterator for the keys or values of the map, respectively. We can then use a for...of
loop to iterate over the keys or values. Here’s an example:
const myMap = new Map([
['key1', 'value1'],
['key2', 'value2'],
['key3', 'value3']
]);
for (const key of myMap.keys()) {
console.log(key);
}
for (const value of myMap.values()) {
console.log(value);
}
In this example, the first for...of
loop iterates over the keys of myMap
, and the second loop iterates over the values of myMap
.
Method 4: entries() method
The entries()
method of a TypeScript Map object returns an iterable object that contains an array of [key, value]
pairs for each element in the Map, in insertion order. Here’s an example of how to use it:
// create a new Map object
const myMap = new Map([
['key1', 'value1'],
['key2', 'value2'],
['key3', 'value3']
]);
// iterate over the entries of the Map using the entries() method
for (const [key, value] of myMap.entries()) {
console.log(`Key: ${key}, Value: ${value}`);
}
This will output the following:
Key: key1, Value: value1
Key: key2, Value: value2
Key: key3, Value: value3
As you can see, the entries()
method allows you to easily iterate over the key-value pairs of a Map object and extract both the key and value of each element.
If you’re not sure which method to use, refer to this comparison table:
Iterating Methods Comparison
Method | When to Use | Example | Returns |
keys() | When you only need the keys of the Map | for (let key of myMap.keys()) { console.log(key); } | An iterator of all keys in the Map |
values() | When you only need the values of the Map | for (let value of myMap.values()) { console.log(value); } | An iterator of all values in the Map |
entries() | When you need both keys and values of the Map | for (let [key, value] of myMap.entries()) { console.log(key, value); } | An iterator of arrays containing the key-value pairs of the Map |
forEach() | When you want to execute a function on each key-value pair of the Map | myMap.forEach((value, key) => { console.log(key, value); }); | undefined |
The best method to use depends on what you need to accomplish in your code. If you only need to iterate over the keys or values, then use keys()
or values()
. If you need both keys and values, then use entries()
. Finally, if you need to execute a function on each key-value pair, then use forEach()
. By selecting the appropriate method for the task at hand, you can improve the readability and efficiency of your code.
When to use map TypeScript? (With Example)
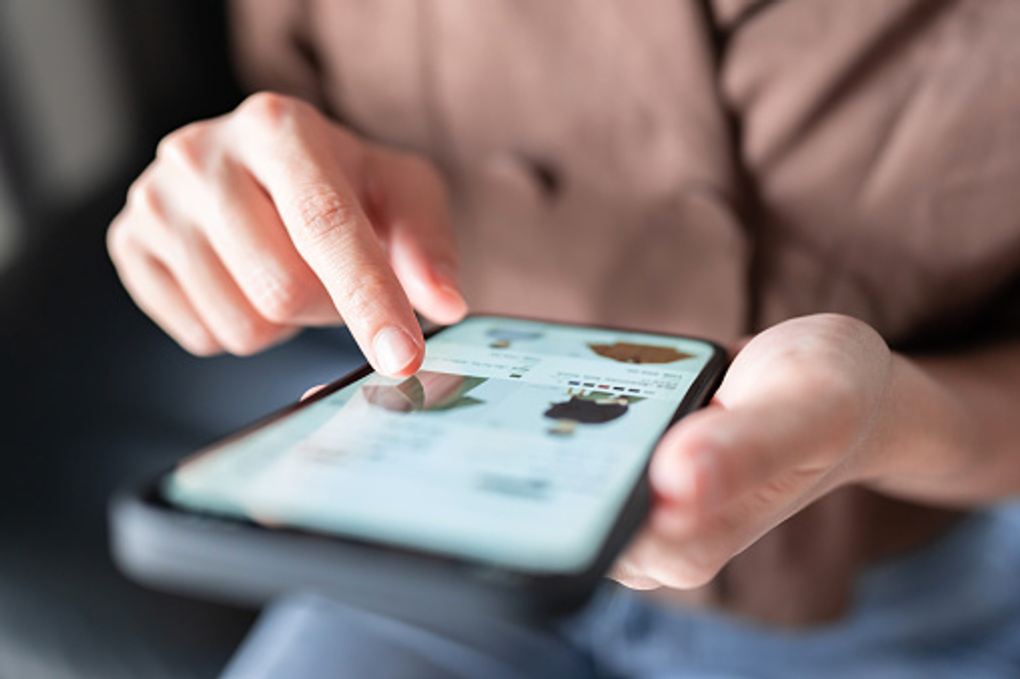
Here’s an example of how to create and use a TypeScript Map in a real-life scenario:
Let’s say you’re building an e-commerce website, and you want to keep track of the items that customers add to their cart. You decide to use a TypeScript Map to store this information because it provides a convenient way to store key-value pairs.
To create a map in TypeScript to store the cart items, you would use the following code:
const cart = new Map<number, {name: string, price: number, quantity: number}>();
In this example, we’re using a number as the key and an object as the value. The object contains the name, price, and quantity of the item.
To add an item to the cart, you would use the following code:
cart.set(1, {name: 'T-shirt', price: 19.99, quantity: 2});
This adds a T-shirt to the cart with a price of $19.99 and a quantity of 2. The key for this item is 1.
To retrieve an item from the cart, you would use the following code:
const item = cart.get(1);
This retrieves the item from the cart with the key of 1. You can then access the name, price, and quantity of the item using the dot notation:
console.log(item.name); // Output: T-shirt
console.log(item.price); // Output: 19.99
console.log(item.quantity); // Output: 2
To update an item in the cart, you would simply use the set() method again with the same key:
cart.set(1, {name: 'T-shirt', price: 19.99, quantity: 3});
This updates the quantity of the T-shirt to 3.
To remove an item from the cart, you would use the delete() method:
cart.delete(1);
This removes the item from the cart with the key of 1.
Overall, TypeScript Map provides a clean and easy-to-use way to manage a customer’s cart on an e-commerce website. It allows you to quickly add, retrieve, update, and remove items from the cart using a simple key-value pairing system.
How to create array of map in TypeScript?
To create an array of TypeScript Map objects, you can use the Array.map() method and pass a function that returns a new Map object for each element in the array. Creating Typescript array Map of objects in TypeScript can be helpful when storing and managing a collection of Maps. Here is an example of how you can create an array of Map objects in TypeScript with Typescript map type:
Step 1: Define the type of the Map
type MyMap = Map<string, number>;
Step 2: Initialize an array of Map objects
const mapArray: MyMap[] = [];
Step 3: Create a new Map object
const map1 = new Map<string, number>();
Step 4: Add key-value pairs to the Map object
map1.set("key1", 1);
map1.set("key2", 2);
Step 5: Add the Map object to the array of Map objects
mapArray.push(map1);
Step 6: Create another Map object and add it to the array
const map2 = new Map<string, number>();
map2.set("key3", 3);
map2.set("key4", 4);
mapArray.push(map2);
Now the mapArray
contains two Map
objects that can be accessed and manipulated like any other Map
.
To iterate over an array map, watch this quick tutorial:
Closing Thoughts
TypeScript Map is a powerful tool that can make managing complex data structures in web development projects much more manageable. With the ability to quickly and easily add, retrieve, and remove values and iterate over keys and values in multiple ways, TypeScript Map can significantly simplify your code and make it more maintainable. Taking advantage of TypeScript Map in your web development projects can improve your efficiency and streamline your code, ultimately saving time and resources. So why not try and see how it can benefit your development workflow?