- Introduction
- Typescript: A Brief Summary
- Getting Started With Arrays In Typescript
- Features of an Array
- How to Declare or Initialize a Typescript Array
- Typescript Array Types
- Array Destructuring
- Storing Values of Mixed Types
- Accessing Array Elements
- Typescript Array Traversal using the "for" loop
- Typescript Array Methods and Properties
- FAQ
- Conclusion
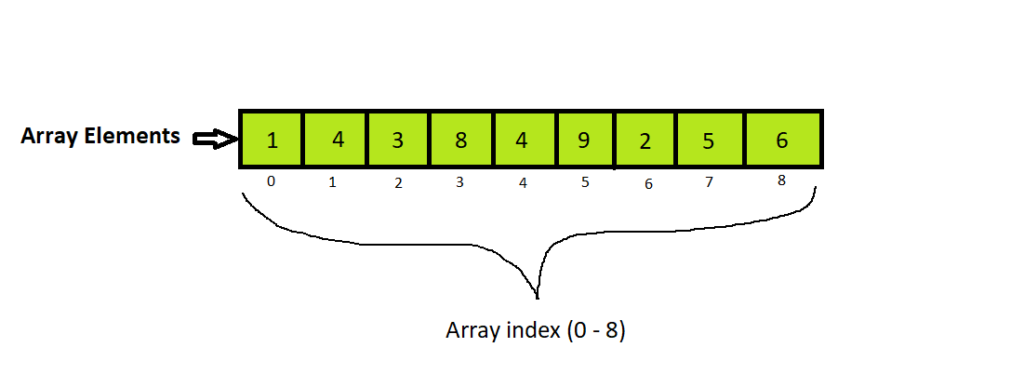
Introduction
Arrays are an important concept and data structure in programming.
Being the conventional and most popular structure, especially in front-end development, it is very important to be familiar with it.
In this article, we will discuss Typescript arrays in detail, their syntax, and apply them in different use cases.
However, before we continue, I’d like to introduce you to CopyCat. CopyCat is a cool tool that you can use to speed up your development. It does this by converting your Figma files to up-and-running React applications. Not only that, but it also has support for your favorite tools like Typescript, Bootstrap, and TailwindCSS, amongst others! Check it out here!
Typescript: A Brief Summary
TypeScript is an open-source programming language built and maintained by Microsoft.
According to the Stack Overflow Developer Survey 2022, it is the fifth most popular technology in software engineering.
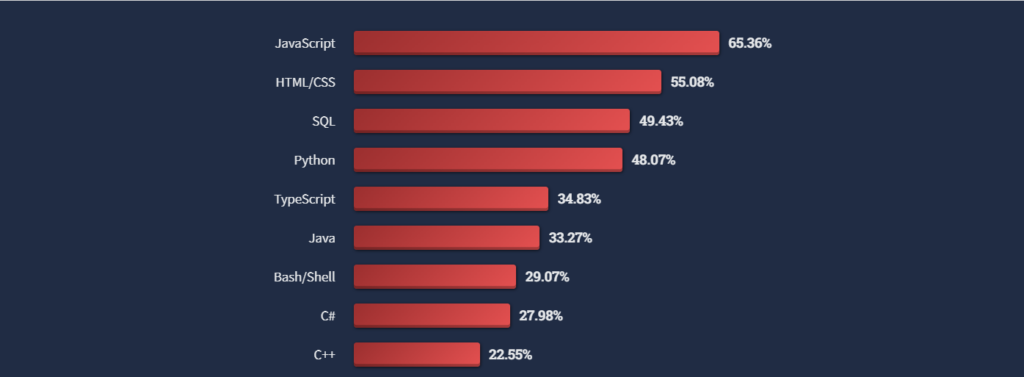
It is a strict syntactical superset of JavaScript that extends the language with optional static typing. It transpiles to JavaScript and is intended for large-scale application development. Existing JavaScript programs are also valid in TypeScript because it is a superset of JavaScript.
Here are a few resources to get you familiar with Typescript if you want to learn more:
- TypeScript Documentation and Playground
- Learn TypeScript – Full Tutorial – YouTube
- TypeScript Tutorial for Beginners [2022] – YouTube
- What to Read When Using Typescript In Your React App
- All You Need to Know About TypeScript Types
Getting Started With Arrays In Typescript
An array is a user-specified data type. It is a group of related data elements kept in close proximity to one another in memory.
It is the most basic data structure because each element in the array can be retrieved directly using only its index number. The first element has the lowest index i.e. 0, while the last element has the highest index, i.e. “n” (where “n” is the length of the array).
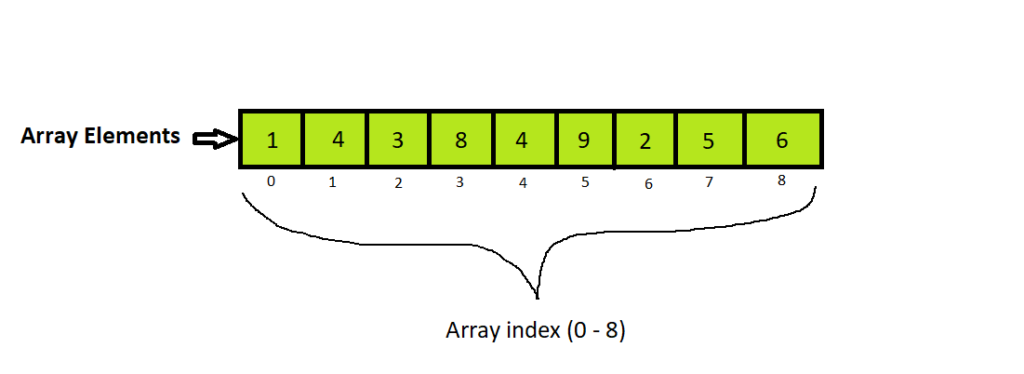
Features of an Array
Here is a list of an array’s features:
- An array declaration creates sequential memory chunks.
- Arrays are fixed and static. This indicates that an array cannot be resized once it has been initialized.
- An array element is represented by each memory block.
- Array items are identified by a unique number known as the element’s subscript or index.
- Arrays, much like variables, must be declared before they can be used.
- Initializing an array involves filling it with elements.
- The numeric value of an array’s element can be changed or updated but not deleted.
How to Declare or Initialize a Typescript Array
There are two ways to declare a Typescript array. They are as follows;
Square Notation
This is the conventional method of declaring an array both in Javascript and Typescript.
let names = ['Anna', 'Jane', 'Bob'];
Generic Array Typing
You can also declare a Typescript array by using the Typescript generic Array type. The type operator is usually separated from the array constant by a semi-colon.
We can demonstrate this with a simple example;
let fruits: Array<any> = ['Strawberry', 'Pineapple', 'Apple'];
It’s important to note that an array can be initialized and declared separately like so;
let fruits: Array; // declaration
fruits = ['Strawberry', 'Pineapple', 'Apple']; // initialization
Type Inference
Citing our “names” example above, if we declared our arrays like above, we would not be maximizing the power of Typescript’s type system because right now, if we push a number into our names array like so,
let names: Array<any> = ['Anna', 'Jane', 'Bob'];
names.push(2);
it would work just fine. However, the number “2” is not a fruit. This can cause errors later on in our code.
So how do we prevent this?
We can take our array declaration a step further by strictly typing the array so that it only takes elements of a specific type.
Using the square notation, we could type our “names” array to only accept strings like so;
let names: string[] = ['Anna', 'Jane', 'Bob'];
By simply adding “string” before our square brackets, we tell Typescript that our “names” array is a Typescript array that only contains and accepts strings.
With the Typescript generic Array type notation, we can add the preferred array type using the Array<ElementType> format like below;
let names: Array<string> = ['Anna', 'Jane', 'Bob'];
Now, when we try to add a number or any other data type asides from a string to our “names” array,
names.push(2);
Typescript throws an error that reads “Argument of type ‘number’ is not assignable to parameter of type ‘string'” like so;
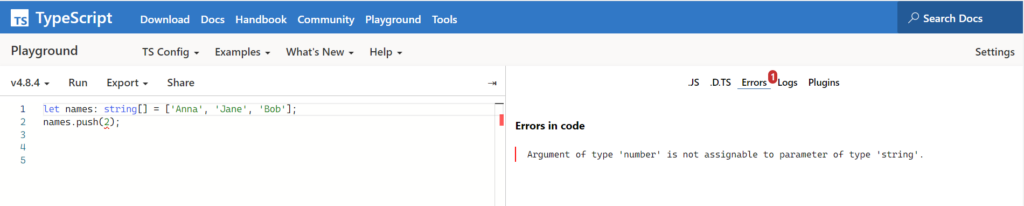
To learn more about Types in Typescript, check out this article by CopyCat – All You Need to Know About TypeScript Types.
Typescript Array Types
There are two different types of arrays in Typescript and, generally, in programming. They are;
- Single-dimensional Array
- Multi-dimensional Array
Single-dimensional Array
A single-dimensional array, often known as a one-dimensional array, is essentially a linear list of elements or items. It is the simplest type of array since the items are evaluated sequentially.
A single-dimensional array can also be defined as a set of variables with comparable data types that enables you to evaluate each element by referring to its index.
Below is an example of a single-dimensional array;
let names: Array<string> = ['Anna', 'Jane', 'Bob'];
Multi-dimensional Array
Simply put, a multidimensional array is an array of arrays.
In a multi-dimensional Typescript array, data is stored in rows and columns, just like in a matrix. Therefore, we can leverage multidimensional arrays to create matrices and data structures.
A two-dimensional array, for example, would look like this;
let cars: Array<string> = [
["Mercedes Benz", "Porsche"],
["Ferrari", "BMW"]
]
Depending on how many nested levels exist in a multidimensional array, we can have two-dimensional arrays, three-dimensional arrays and even more.
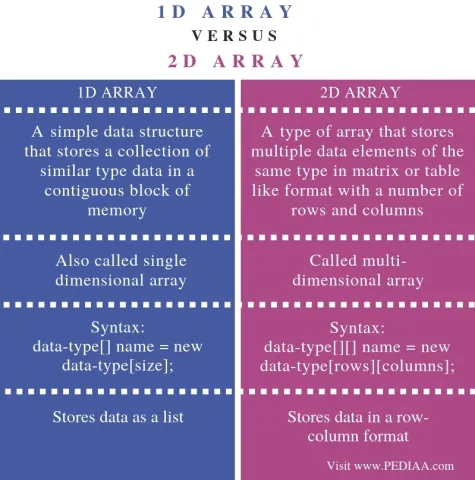
Array Destructuring
Destructuring is an important feature of arrays. It’s a clean way to extract values from an array. Typescript also supports array destructuring.
We can demonstrate this below;
var arr:string[] = ["Anne","Jeff"];
var[x,y] = arr;
console.log(x);
console.log(y);
What the Typescript compiler does is assign the value of the exact index to the corresponding variable. i.e., x will be assigned to Anne while y will be assigned to Jeff.
So, when we log both to the console, we get;
"Anne"
"Jeff"
Storing Values of Mixed Types
Typescript also allows for mixed-type arrays, i.e., arrays that take values of different types.
In this case, all the types specified are separated by a “|,” with each type matching the preceding element.
Example to store multiple values;
var Random: Array < string | number >
= ['Jane', 6, 'Anna', 3, 12, 'Sophia', 8];
Accessing Array Elements
Accessing Array Elements is done by using the index or subscript of the element using the ArrayName[index] format. Since the first array index is zero, the index of the first element is zero, the index of the second element is one, and so on.
Example;
let names: Array<string> = ['Anna', 'Jane', 'Bob'];
console.log(name[0]); // 'Anna'
console.log(name[1]); // 'Jane'
console.log(name[2]); // 'Bob'
Typescript Array Traversal using the “for” loop
One of the major features of arrays is their iterability. This means we can loop through an array, performing various types of computations on all or some of the elements in that array.
A classic example is an iteration via the “for” loop. We can demonstrate this below;
let fruits: string[] = ['Strawberry', 'Pineapple', 'Apple'];
for(var i = 0; i < fruits.length; i++)
{
console.log(fruits[i]); // output: Strawberry Pineapple Apple
}
Another variation of the for loop is the “for…in” loop and it would give us the same result as above;
for(var index in fruits)
{
console.log(fruits[index]); // output: Strawberry Pineapple Apple
}
Typescript Array Methods and Properties
Arrays are capable of undergoing so many computations and operations, and one way we can perform these operations with them is via array methods and properties. They are;
concat()
concat() creates a new array by joining the current array with another array(s) and/or a value (s).
every()
every() returns true if every element in the array satisfies the provided testing function.
filter()
filter() produces a new array containing all of this array’s items for which the specified filtering function returns true.
forEach()
forEach() executes a function for each array element.
indexOf()
indexOf() returns the first (least) index of an element with the supplied value inside the array, or -1 if none are found.
join()
join() creates a string by joining every element in the array.
lastIndexOf()
lastIndexOf() returns either -1 if no element matching the provided value is found or the final (largest) index of that element in the array.
map()
map() generates a new array that contains the output of invoking a given function on each item of the original array.
pop()
pop() removes the last element from an array and returns it.
push()
push() increases the length of an array by adding one or more elements to the end.
reduce()
reduce() applies a function on two array values (from left to right) in order to reduce them to a single value.
reduceRight()
reduceRight() does the same thing as reduce. Only this time, it’s from right to left
reverse()
reverse() changes the order of an array’s elements such that the first element becomes the last and vice versa.
shift()
shift() returns the element it removed from an array after removing the first element.
slice()
slice() produces a new array after extracting a portion of an array.
some()
some() returns true if at least one element in the array satisfies the provided testing function.
sort()
The sort() function sorts the items of an array.
splice()
splice() adds and/or removes elements from an array.
toString()
toString() returns a string containing the members of the array.
unShift()
unShift() increases the number of elements at the beginning of an array and returns the array’s new length.
FAQ
What is the difference between a Tuple and an Array in TypeScript?
A tuple is often an array with a defined size and well-known data type. This means that you would need a tuple for a static, clearly defined array.
Let’s demonstrate this with an example;
Say we have a tuple of 3 strings like so;
let names: [string, string, string] = ['Anna', 'Jane', 'Bob'];
At first glance, we can already tell the difference between a tuple and the Typescript array, given the example above.
A tuple clearly defines the item type it accepts(well-known data type) and exactly how many items it will take(defined size).
Therefore, if we take away one string from our names tuple, Typescript returns an error like the below;

We also get an error when we try to extra string to the tuple.
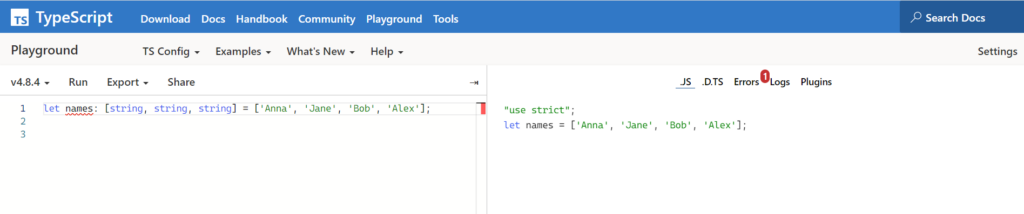
Arrays, on the other hand, do not have this restriction and can accommodate as many items as required. Check out this article on Typescript types – All You Need to Know About TypeScript Types.
How do you declare an array of any type in TypeScript?
A Typescript array with type any, just like its type implies,accepts items of any type. You can declare an array of any type like below;
let fruits: Array<any> = ['Strawberry', 'Pineapple', 'Apple'];
How do you interface an array of objects in TypeScript?
If you want to define an interface for an array of objects, specify the interface for each array object’s type and set the array’s type to Type[]. The type of each item you add to the array must match for the type checker to succeed.
We can demonstrate this below;
// All objects have predefined type
interface User {
id: number;
name: string;
}
const arr: User[] = [
{ id: 1, name: 'Anne' },
{ id: 2, name: 'Jane' },
];
We can also extend this interface like so;
// Objects with extendable type
interface ExtendableUser {
id: number;
name: string;
[key: string]: any; // index signature
}
const arr2: ExtendableUser[] = [
{ id: 1, name: 'Bob' },
{ id: 2, name: 'Jane', salary: 100 },
];
Are arrays a reference type in TypeScript?
Yes, Array is a reference type.
How do you find the type of an array?
We can get the type of an array using the typeof() method. Check out this article explaining in detail – Everything You Need to Know About TypeScript Typeof Operator
Conclusion
Now that you know all the power there is in Typescript Arrays, we hope that you find this article helpful.
At CopyCat, we have a blog filled with well-articulated and easy-to-understand articles for React developers, just like this one. Check it out here!