Introduction
As a web developer, it can be tempting to focus on mastering one programming language, such as JavaScript, to become an expert. However, a broad understanding of web development is often more valuable in the long run. You can become a more versatile and adaptable developer by understanding the full spectrum of web development, from front-end to back-end to DevOps. You will also be able to take on a wider range of projects and better understand your client’s needs. This article will discuss why mastering coding fundamentals are more valuable than expertise in one language and how you can achieve it.
Debunking Myths
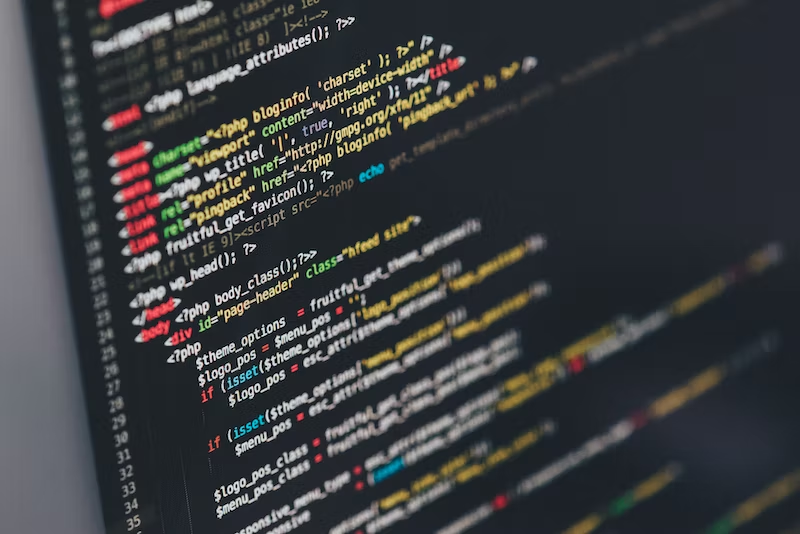
The belief that mastering one computer language is more important than learning coding fundamentals is a common misconception among developers. Coding fundamentals are the building blocks of computer science and are essential for solving complex problems and building reliable software. With a solid understanding of the basics, you will be able to adapt to new technologies. As they say, you want to be the jack of all trades and master of none.
In addition, fundamentals can be applied to multiple programming languages and make learning new languages and technologies easier. Additionally, coding fundamentals can improve your problem-solving skills and make you more valuable to potential employers. In a rapidly evolving software industry, it’s essential to be adaptable and deeply understand computer science concepts. You can become a more versatile, adaptable, and skilled developer by learning coding fundamentals.
Why You Should Focus on Learning Coding Fundamentals
There are several reasons why learning coding fundamentals is more important than mastering one language.
Coding Fundamentals Apply to Multiple Languages
While it is undoubtedly important to be proficient in the language you are using, having a strong foundation in coding fundamentals can make learning new languages and technologies easier. This versatility can be particularly valuable in a rapidly evolving software industry where new languages and technologies are constantly being developed.
Coding Fundamentals Improve Your Problem-Solving Skills
Algorithms and data structures are key tools for solving complex problems and can be applied to various situations. By learning these concepts, you become a better problem-solver and more effective at tackling challenges.
Coding Fundamentals Make You More Valuable to Potential Employers
In a competitive job market, it is important to stand out and demonstrate your value to potential employers. Strong coding fundamentals show that you have a deep understanding of computer science concepts and are well-equipped to tackle complex problems. This can make you more attractive to potential employers and open up more job opportunities.
Coding Fundamentals Help You Stay Current in Your Field
As mentioned earlier, the software industry is constantly evolving, and new languages and technologies are constantly developed. You can quickly adapt to new technologies and stay current by having a solid foundation in coding fundamentals. This can be particularly valuable for React developers, as the React ecosystem constantly evolves and new libraries and tools are introduced.
Pros and Cons of Learning Coding Fundamentals
Pros:
- Coding fundamentals can be applied to multiple programming languages and make learning new languages and technologies easier.
- Coding fundamentals can improve your problem-solving skills and make you a more effective developer.
- Coding fundamentals can make you more valuable to potential employers and enhance your job prospects.
- Coding fundamentals can help you stay current in a rapidly evolving software industry.
Cons:
- Learning coding fundamentals can be time-consuming and may require a significant investment of time and resources.
- Some developers may prefer to focus on mastering a single programming language. As a result, they may need to see the value in learning coding fundamentals and be slower at adapting.
- It can be challenging to learn coding fundamentals, especially if you are new to programming or have limited experience.
Overall, the pros of learning coding fundamentals tend to outweigh the cons. While learning coding fundamentals may take time and effort, the benefits can be significant. This includes enhanced job prospects, improved problem-solving skills, and adaptability to new technologies. Whether you are a beginner just starting or an experienced developer looking to expand your skill set, learning coding fundamentals are a valuable investment that can pay off in the long run.
What are the core coding fundamentals?
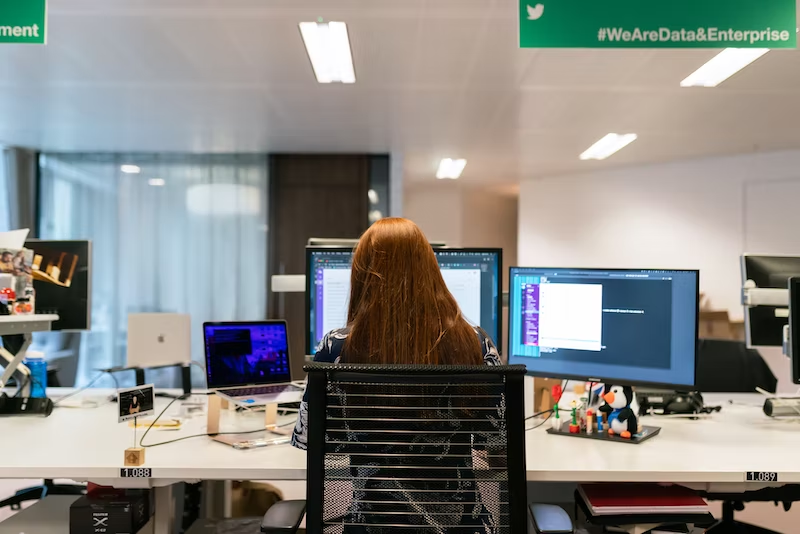
Some of the core coding fundamentals that are particularly important for a junior React developer to learn include:
Algorithms:
An algorithm is a set of steps for solving a problem or achieving a goal. Algorithms are a fundamental part of computer science and are used to solve a wide range of problems, from sorting data to finding the shortest path between two points. As a React developer, learning about different algorithms and how to apply them to solve problems is important. Algorithms are used to optimize the performance of web applications by choosing the most efficient algorithms for a given problem. They’re also used to implement features like recommendation systems, search engines, and machine learning models.
Some popular algorithms to know:
- Sorting algorithms: Sorting algorithms are used to rearrange a list of items into a specific order, such as ascending or descending order. Some popular sorting algorithms include bubble sort, merge sort, and quick sort.
- Search algorithms: Search algorithms are used to find a specific item in a list of items. Some popular search algorithms include linear search and binary search.
- Graph algorithms: Graph algorithms are used to solve problems involving graphs, which are data structures that consist of nodes and edges. Some popular graph algorithms include depth-first search and breadth-first search, which are used to traverse graphs, and Dijkstra’s algorithm, which is used to find the shortest path between two nodes in a graph.
- Dynamic programming: Dynamic programming is an algorithm design technique used to solve optimization problems by breaking them down into smaller subproblems and storing the results of the subproblems to avoid recomputing them.
Here’s a great video about the “5 Algorithms Every Programmer Should Know” by Simple Programmer:
Data structures:
A data structure organizes and stores data in a computer so that it can be accessed and modified efficiently. Some common data structures include arrays, linked lists, stacks, and queues. As a junior React developer, learning about different data structures and how to use them effectively to store and manipulate data is essential. Learning about the time and space complexity of different data structures and how to implement them in code would be best. Below are just a few examples of data structures that every developer should be familiar with. Many other data structures and techniques are important for developers to learn, depending on their specific areas of focus.
Some popular data structures to know:
- Arrays: An array is a contiguous block of memory that stores a fixed-size sequence of elements of the same data type. Arrays are used to store and access data in a linear fashion and are efficient for accessing elements by their index.
- Linked lists: A linked list is a data structure that consists of a sequence of nodes, each of which stores a value and a reference to the next node in the list. Linked lists are used to store and access data in a linear fashion and are efficient for inserting and deleting elements.
- Stacks: A stack is a data structure that stores a collection of elements and allows access only to the element at the top of the stack. Stacks are used to implement last-in, first-out (LIFO) semantics and are useful for implementing undo and redo functions.
- Queues: A queue is a data structure that stores a collection of elements and allows access only to the element at the front of the queue. Queues are used to implement first-in, first-out (FIFO) semantics. They are also useful for implementing job scheduling and communication between processes.
- Trees: A tree is a data structure that consists of a set of nodes organized into a hierarchy. Trees are used to store and access data in a hierarchical fashion and are useful for implementing hierarchical data structures, such as file systems and decision trees.
- Graphs: A graph is a data structure that consists of a set of nodes and a set of edges that connect the nodes. Graphs are used to store and access data in a non-linear fashion and are useful for representing relationships between data, such as in social networks and transportation systems.
If you’re in a hurry to learn data structures, watch this 8 hour course about the “5 Data Structures You Should Know as a Software Engineer” by Simple Programmer:
Object-oriented programming (OOP):
OOP is a programming paradigm that is based on the concept of “objects”, which are data structures that contain both data and methods that operate on that data. OOP is a key concept in many programming languages, including JavaScript, and is an important aspect of React development. It is important to learn about OOP concepts, such as encapsulation, inheritance, and polymorphism, and how to apply them in your code. It’s also good to learn about the benefits and limitations of OOP and how to design and implement object-oriented systems. You can learn what an OOP is by watching “OOP (Object Oriented Programming) Explained Simply” by LearnCode.academy:
Design patterns:
A design pattern is a reusable solution to a common software design problem. Design patterns are useful for building maintainable and scalable software, and are an important aspect of software development. As a result, it is important to learn about common design patterns and how to apply them in your code. It’s also a good idea to learn about the benefits and limitations of design patterns and how to choose the most appropriate pattern for a given problem. Watch this crash course to learn “Design Patterns in 8 Minutes” by LearnCode.academy:
Testing:
Testing is a crucial part of software development and is used to ensure that code is correct and reliable. Being able to test efficiently and effectively is a key skill any good developer has. Hence, it’s important to learn about different types of testing, such as unit testing and integration testing, and how to apply them to your code. By learning about testing, you can also ensure that your code is correct and of high quality.
Watch this great video for a quick summary of coding fundamentals you should know. “Coding Fundamentals: What Every Programmer Needs to Know” by CS Dojo:
How Web Developers Master Coding Fundamentals
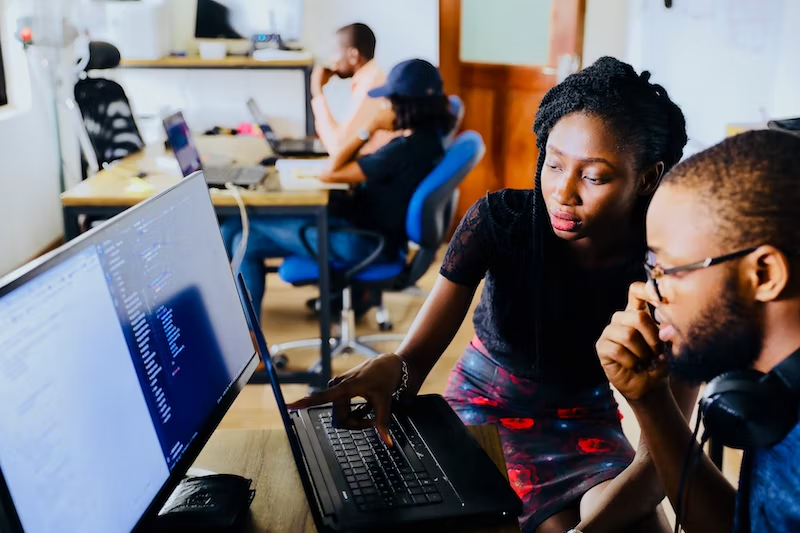
Solve coding challenges
The best way to master coding fundamentals is to spend time practicing and applying them to real-world problems. Solve coding challenges on websites and work on small side projects to gain hands-on experience. Here are some front end developer friendly sites that offer coding challenges.
- FreeCodeCamp: FreeCodeCamp is a non-profit organization that offers a comprehensive curriculum for learning web development, including front-end development. It includes interactive coding challenges, projects, and articles that cover HTML, CSS, and JavaScript.
- Frontend Mentor: Frontend Mentor offers a variety of coding challenges that focus on building real-world websites and user interfaces. Each challenge includes a design file and a set of user stories that describe the functionality that needs to be implemented.
- CSS Diner: CSS Diner is a website that offers a series of interactive CSS selectors challenges. It helps to strengthen your skills in writing CSS selectors and how to target specific elements in your HTML.
- JavaScript30: JavaScript30 is a course offered by Wes Bos, where he provides 30 different coding challenges that focus on JavaScript. Each challenge covers different JavaScript concepts such as DOM manipulation, events and more.
- Codecademy: Codecademy is an online learning platform that offers courses in web development, including a course on front-end development that covers HTML, CSS, and JavaScript. It also offers interactive coding challenges and projects to help you apply what you’ve learned.
These are some example of websites that offers front-end specific coding challenges, and by working through them, you will be able to practice and improve your skills in HTML, CSS, and JavaScript, and building user interfaces.
Join frontend specific communities
Seek out experienced developers on different platforms and learn from them. Some popular platforms include discord, slack, and reddit. Below are some specific communities that are great to join and seek out support or community.
- Front-end Developers: This discord community is focused on front-end development and it covers a wide range of topics such as HTML, CSS, JavaScript, React, Angular, and Vue.js, also providing a place to share your projects and get feedback.
- LearnWebDev: This discord community is focused on helping people learn web development, including front-end development, with the help of a community of experienced developers.
- Devcord: This discord community answers queries related to front-end development and coding. It has a community of over 39,000 members and welcomes newbies to join their platform.
- CodeNewbie: This online community is geared towards coding newbies and has a great panel for any coding questions and advice.
- DevChat: A friendly group of developers that give away free knowledge and help. It’s a very friendly community, open to and respectful of every programming language, level of experience, age, time zone, gender, etc.
- Reactiflux: This is the official Discord community for Reactiflux, a community of developers who love React.js. You can ask for help, give help, and get in touch with people who are passionate about React.
Building and experimenting with Progressive Web Applications (PWAs)
PWAs are web apps that can be installed on a user’s device and run offline. Building and experimenting with PWAs can help web developers master coding fundamentals by gaining a deeper understanding of web app functionality, offline capabilities, and how to use service workers.
Building and experimenting with Serverless architecture
Serverless architecture is a way of building and running applications and services without having to manage infrastructure. By building and experimenting with Serverless architecture, web developers can master coding fundamentals by gaining a deeper understanding of event-driven programming, and how to design and build scalable and cost-effective systems.
Building and experimenting with GraphQL
GraphQL is a query language for your API that allows clients to define the structure of the data they need. By building and experimenting with GraphQL, web developers can master coding fundamentals by gaining a deeper understanding of API design, data fetching and how to handle client needs with flexible and efficient way.
By following these tips, you can build a strong foundation in coding fundamentals and become a more skilled and adaptable developer.
Conclusion
In conclusion, while mastering a single language can be valuable, learning coding fundamentals is often more important, especially for junior React developers looking to improve their skills. You can become a more versatile, adaptable, and skilled developer by building a strong foundation in coding fundamentals. Investing time in basics can improve your job prospects and take in a broader range of projects. Whether you are a beginner just starting or an experienced developer looking to expand your skillset, learning coding fundamentals is an important step to help you achieve your goals and succeed in the software industry.
You can also use CopyCat to achieve your strong foundation in coding basics. Try it out on your favorite design and test how well you know your fundamentals.