- What are React Props?
- How Props works in React
- Creating a React App
- How Props Works in Class Components
- How Props Works in Functional Components
- Examples of React Props Data Type
- Special Props in React Component
- Destructuring React Props
- Destructuring Props in React Class Components
- Destructuring Props in React Functional Components
- Difference Between Props and States in React?
- What Data Type can I Pass as a Prop Value?
- What are React PropTypes?
- Conclusion
What are React Props?
Props represent a component’s ” properties, ” and it’s similar to the concept of parameters and arguments in JavaScript functions. Props enable us to share dynamic data from one component to another.
How Props works in React
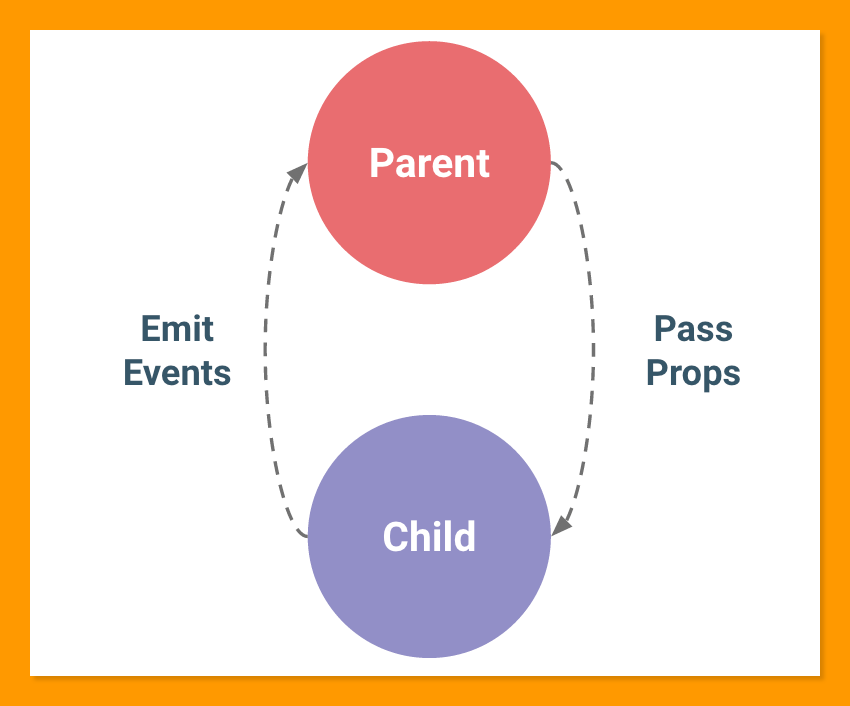
- React uses unidirectional data flow, which means that props are passed from the top component to the bottom component. This is useful for only transferring data (properties) from a parent component to a child component (and not the other way around).
- Component props are an object data type and are present in both class and functional components.
- Component props are a type of object and any JavaScript data type can be passed into the component as props.
- Component props are read-only. They cannot be modified from the child component that receives the prop. However, the child component can emit events to modify the props.
- Component props can also be used to pass state to other components.
In the next section, we’ll set up a new React application for this tutorial.
Creating a React App
Skip this section if you have an existing React application playground.
Or use the following command to create a new react application:
npx create-react-app my-app
Next, run the following command to navigate to your newly generated app directory:
cd my-app
Then run the following command to start your React server:
yarn start // or npm start
Now, your server should launch in your browser at http://localhost:3000/, as shown below:
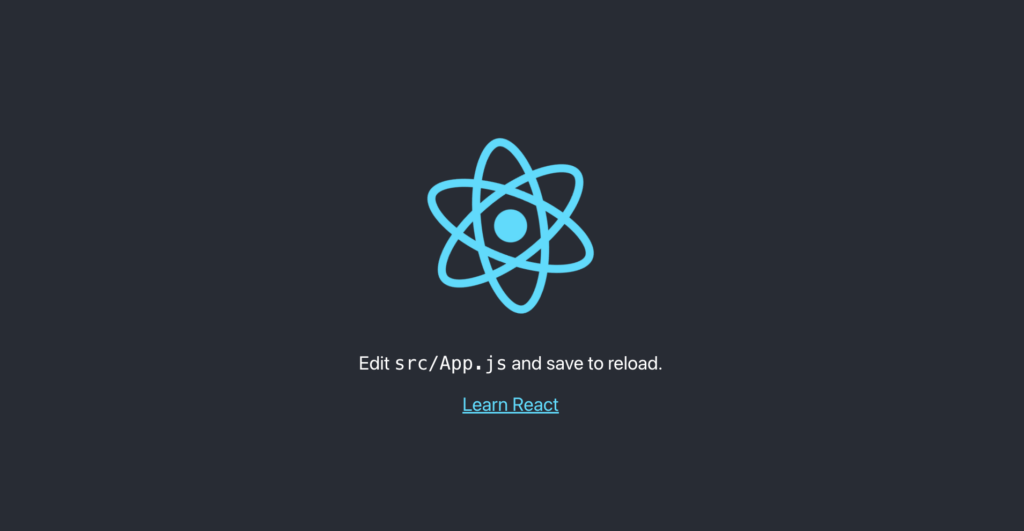
In the next sections, we’ll discuss how props work in React class and functional components.
How Props Works in Class Components
Accordingly, in this section, we’ll discuss how props are defined and accessed in React class components.
How to Set Props in Class Components
Props are accessed through this.props in a class component, meaning that we don’t have to declare the props while creating a class component.
Given that we want to create an Emoji class component that renders the user’s favourite smiley.
Create a new Emoji.jsx file in the src folder with the following class component:
import React, { Component } from "react";
import "./styles.css";
export default class Emoji extends Component {
render() {
return (
<section className='container'>
<h2>My favorite emoji is {this.props.smiley}</h2>
</section>
);
}
}
From the component above, we’re able to access the smiley from the props object using the dot notation because the component props is an object and that’s where new props will be added for the component.
Next, create a new styles.css ****file inside the src folder with the CSS below, to have the same interface as this tutorial:
.container{
display: flex;
height: 100vh;
align-items: center;
justify-content: center;
background-color: #121212;
color: #fff;
}
h2{
font-size: 2rem;
font-family: 'Courier New';
}
Passing Props to Class Components
Props are passed to components as HTML attributes, such as the src and alt attributes in the img tag.
<img src="" alt=""/>
We can then pass the smiley prop to the Emoji component when rendered as shown below:
<Emoji smiley='😜' />
Then, render the Emoji component in the App.js file as shown in the below code:
import React from "react";
import Emoji from "./Emoji";
function App() {
return (
<React.Fragment>
<Emoji />
</React.Fragment>
);
}
export default App;
Our Emoji component will output the following on the browser:
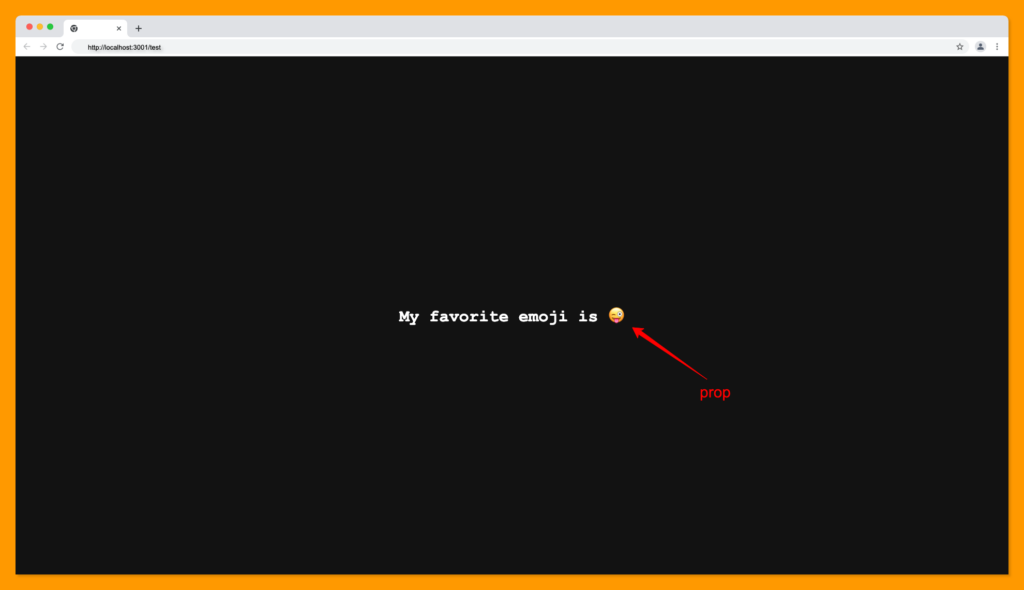
When a smiley prop is not passed to the Emoji component, like this:
<Emoji />
The Emoji component will then render as follows:
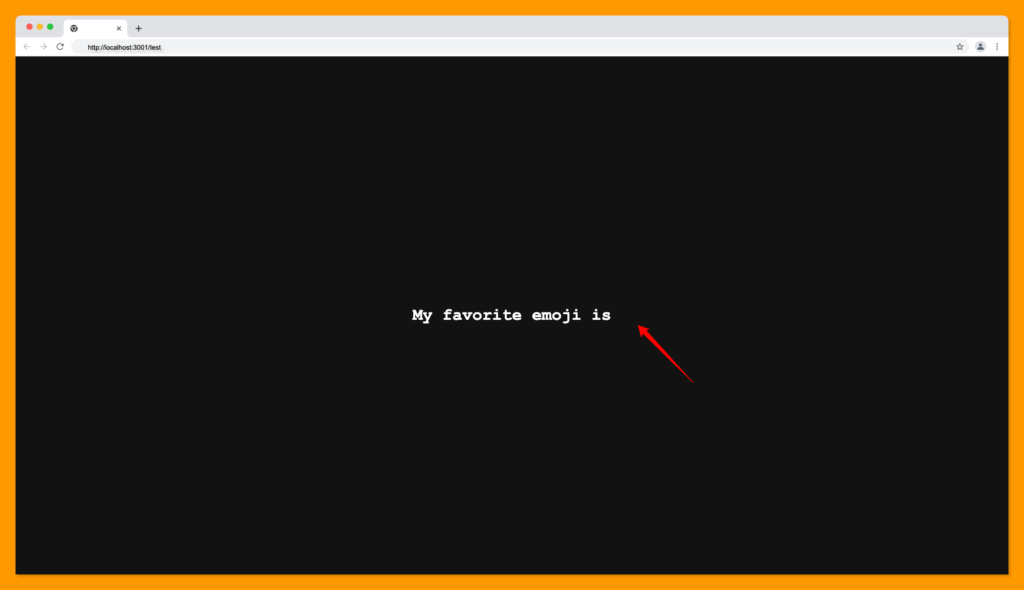
Presently, we can improve this using the default props. The next section will teach us how to set default props in a class component.
How to Set Default Props in Class Components
As shown in the previous section, we sometimes want our component props to have a default value that will be displayed when the prop is not passed to the component when rendered.
We can make use of the defaultProps property to set the default value for a component prop likewise:
Emoji.defaultProps = {
prop: defaultValue,
};
Our Emoji component will now look as follows with the default smiley value set to “👋”:
import React, { Component } from "react";
import "./styles.css";
export default class Emoji extends Component {
render() {
return (
<section className='container'>
<h2>My favorite emoji is {this.props.smiley}</h2>
</section>
);
}
}
Emoji.defaultProps = {
smiley: "👋",
};
Following, the default props are set at the bottom of the component, and we can set as many props in the defaultProps object.
Emoji.defaultProps = {
prop1: defaultValue,
prop2: defaultValue,
prop3: defaultValue,
propX: defaultValue,
};
Now, the Emoji component will render the default smiley when one is not passed to the component:
<Emoji />
If we don’t pass data, the Emoji component will output the following:
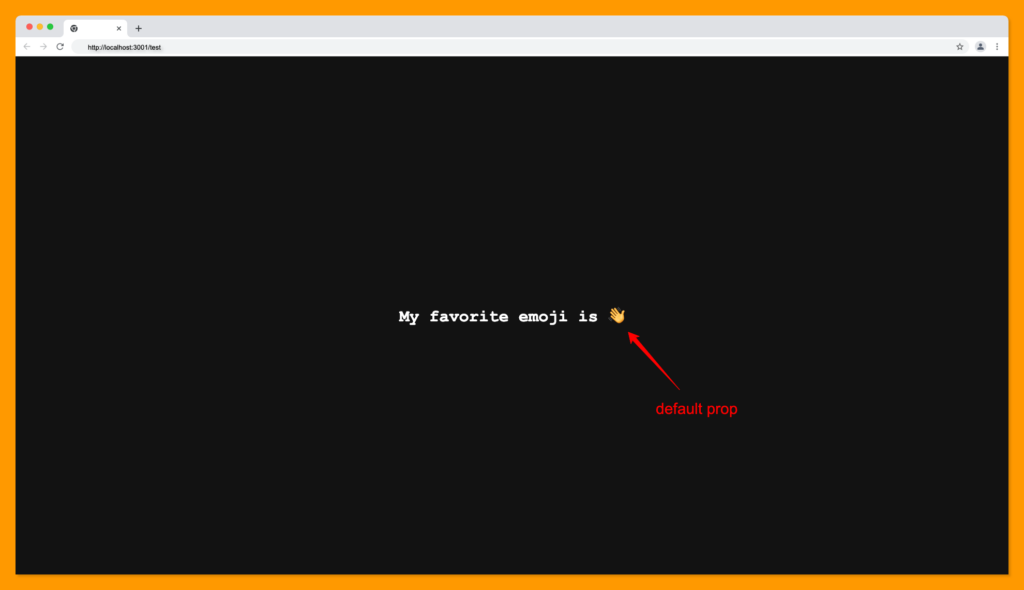
How Props Works in Functional Components
In this section, we’ll discuss how props are defined and accessed in React functional components.
For this section, create a new component named Fruit.jsx in the src folder
How to Set Props in Functional Components
Setting props in a functional component is similar to a regular JavaScript function declaring parameters.
As a result, we can rewrite our emoji class component as a fruit functional component as follows:
import "./styles.css";
const Fruit = (props) => {
return (
<section className='container'>
<h2>My favorite fruit is {props.fruit}</h2>
</section>
);
};
export default Fruit;
Unlike a class component, where we access component props via the built-in this keyword, we access functional component props via the props parameter we’ve declared.
Passing Props to Functional Components
Passing props to a functional component is the same as passing props to a class component which can be assumed as passing arguments to a JavaScript function.
Firstly, render the Fruit component in your app component file as follows:
<Fruit />
The Fruit component will then render as follows:
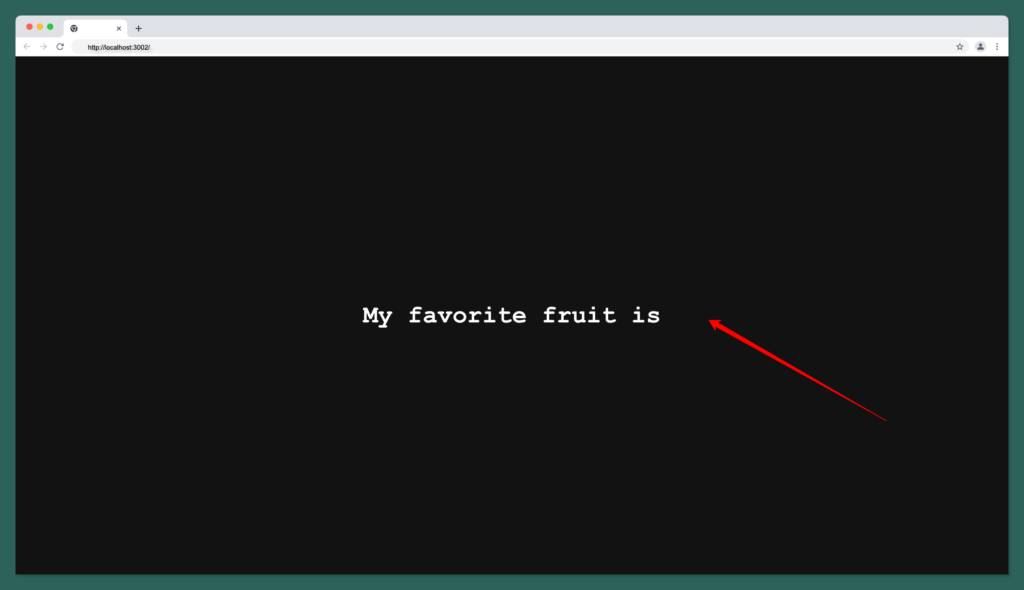
We can also improve this by using the default props. We’ll learn how to set default props in a functional component in the next section.
How to Set Default Props in Functional Components
Similar to class components, we can make use of the defaultProps property to set default values for props in a functional component as follows:
Fruit.defaultProps = {
prop: defaultValue,
};
Our Fruit component will now look as follows with the default fruit value set to “🥭”:
import "./styles.css";
const Fruit = (props) => {
return (
<section className='container'>
<h2>My favorite fruit is {props.fruit}</h2>
</section>
);
};
Fruit.defaultProps = {
fruit: "🥭",
};
export default Fruit;
The default props are also set at the bottom of the component, and we can set as many props in the defaultProps object as shown below:
Fruit.defaultProps = {
prop1: defaultValue,
prop2: defaultValue,
prop3: defaultValue,
propX: defaultValue,
};
Now, the Fruit component will render the default fruit when one is not passed to the component:
<Fruit />
If we don’t pass data, the Fruit component will output the following:
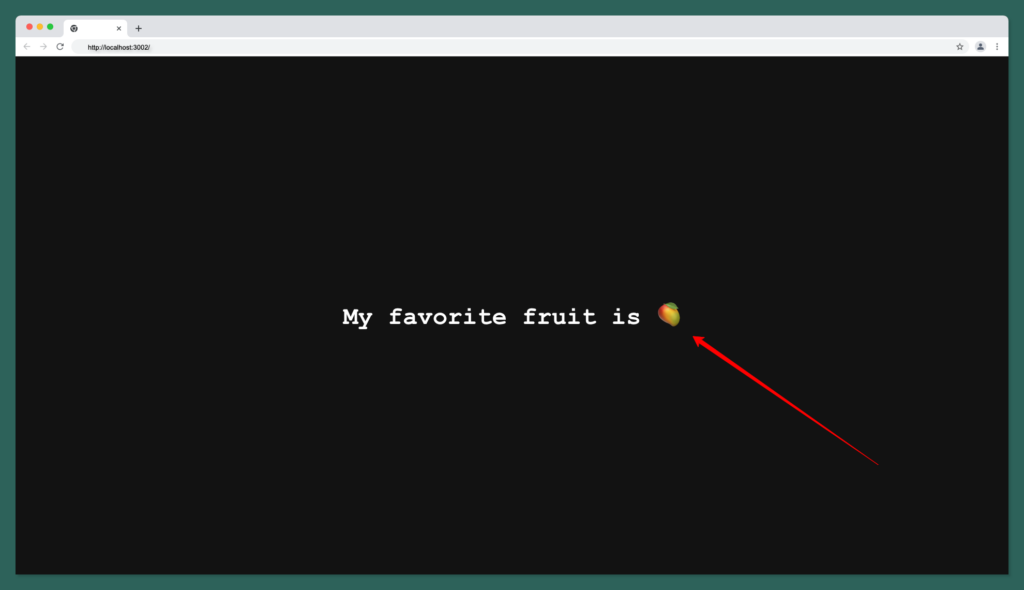
Examples of React Props Data Type
Additionally, any data type can be passed to a component as a prop. The examples below show how to pass common data types to a component as a prop in React.
String as React Component Props
Strings are a common data type in JavaScript and can be passed as a prop using the double or single quotation.
<MyComponent text={"This is a text prop"} />
The string type is the only data type that can be passed to a component as a prop without the curly brackets as shown below:
<MyComponent text="This is a text prop" />
Number as React Component Props
Numbers are primitive data types and can be positive, negative, or in float format, we can pass a number type as a prop to a React component as follows.
A positive number can be passed as a component prop like this:
<MyComponent age={23} />
A negative number can be passed as a component prop like this:
<MyComponent temperature={-23} />
A float or decimal number can be passed as a component prop like this:
<MyComponent measurement={2.3} />
Object as React Component Props
Objects are a non-primitive data type that allows you to store multiple collections of data in JavaScript.
We can pass objects as props in plain objects, as shown below:
<MyComponent
profile={{
name: "Sam",
country: "Nigeria",
}}
/>
Or as an object with a variable name:
// const myProfile = {
// name: "Sam",
// country: "Nigeria",
// };
<MyComponent profile={myProfile} />
Array as React Component Props
Arrays are non-primitive data types that are used to store multiple elements in a single variable.
We can pass arrays as props in plain arrays, as shown below:
<MyComponent favoriteFruit={["orange", "apple", "banana"]} />
Or as an array with a variable name:
// let myFruits = ["orange", "apple", "banana"]
<MyComponent favoriteFruit={myFruits} />
Learn about JavaScript data types below:
Special Props in React Component
React has some built-in props and their names which are reserved should be avoided when you’re creating your own props.
Below is the list of the special props in a React component:
- Children
- Key
- Ref
If you need to make use of this name as a prop to your component, ensure to change the name as demonstrated below:
Don’t do this ❌
<MyComponent key={key} children={children} ref={ref} />
Do this instead ✅
<myComponent myKey={key} myChildren={children} myRef={ref} />
Destructuring React Props
Destructuring is an ES6 shorthand for easily extracting and unpacking an array element or object property into a distinct variable. React props are objects and can be destructured in both class and functional components.
Destructuring Props in React Class Components
We can destructure props in a class component within the render method as follows:
render(){
const {prop1, prop2, prop3, propx} = this.prop;
}
We can apply this in our Emoji class component as shown below:
import React, { Component } from "react";
import "./styles.css";
export default class Emoji extends Component {
render() {
const { smiley } = this.props; // new line
return (
<section className='container'>
<h2>My favorite emoji is {smiley}</h2>
</section>
);
}
}
Our rendering still remains the same:
<Emoji smiley='😃' />
Destructuring Props in React Functional Components
React props can be restructured in two ways in a React functional component.
- Destructuring in the component parameter
export const FunctionalComponent = ({prop1, prop2, prop3, prop4}) => {};
- Destructuring within the functional component:
export const FunctionalComponent = (props) => {
const { prop1, prop2, prop3, prop4 } = props;
};
We can apply either of these methods to our Fruit functional component as follows:
import "./styles.css";
const Fruit = (props) => {
const { fruit } = props; // new line
return (
<section className='container'>
<h2>My favorite fruit is {fruit}</h2>
</section>
);
};
export default Fruit;
You can learn more about JavaScript destructing from the video below:
Difference Between Props and States in React?
Props | State |
---|---|
Props are passed from a parent component | State is created within the component |
Props are the main concept that allows React components to be reusable. | React components are not reusable because of state. |
Props allow you to pass data as an argument from one component to another. | State only keeps track of the component’s information. |
React props are immutable. | State is mutable. |
React Props are read-only. | State changes can be asynchronous. |
What Data Type can I Pass as a Prop Value?
Any data type including React components can be passed as a prop to a React component. All data passed to a component are called props.
What are React PropTypes?
We may unintentionally pass the incorrect data type to a component prop which might crash our app; we can avoid this by using Proptypes. PropTypes are simply a mechanism for ensuring that the passed value is of the correct data type.
You can learn more about Proptypes below:
Conclusion
React props are the main concept that allows components to be reusable in React app. We’ve learned how props work in both React class and functional components.
Finally, you can find more incredible articles like this on our blog at CopyCat. CopyCat is a tool that saves you about 35% of development time by converting your Figma files to an up-and-running React project. By all means, check it out here.
Interesting Reads From Our Blogs
- React Hooks: Understanding How React useRef Works
- How to Add Toast Notifications to a React App Using React Toastify
- React Datepicker Complete Guide With Examples
Check out the CopyCat plugin for React if you’re seeking amazing React tools to help you build component code faster and be production-ready than your competition!
Happy Coding!