- Pitfall #1: Using Too Many Third-Party Libraries
- Pitfall #2: Poor Component Design
- Pitfall #3: Not Optimizing React Performance
- Pitfall #4: Inefficient State Management
- Pitfall #5: Security Vulnerabilities
- Pitfall #6: Poor Performance on Mobile Devices
- Pitfall #7: Over-Rendering Components in React
- Pitfall #8: Inadequate Testing
- Pitfall #9: Overuse of Redux
- Pitfall #10: Not Keeping Up with Updates
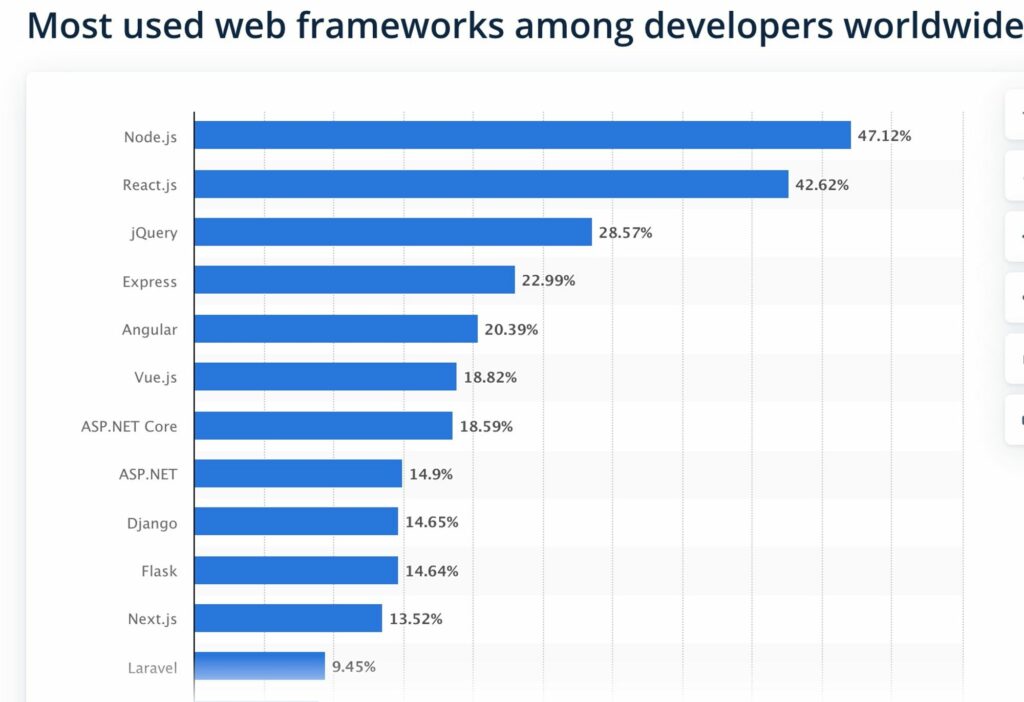
Did you know that React.js is used by over 17 million websites worldwide, including major players like Facebook, Instagram, and Airbnb? It is the most used web frameworks worldwide. This popular JavaScript library has revolutionized web development with its component-based architecture and fast rendering times, enabling developers to create dynamic and interactive user interfaces. However, despite its many benefits, React.js has its fair share of pitfalls that developers should be aware of.
In this article, we look closely at some common pitfalls developers may encounter when working with React.js. From poor component design to security vulnerabilities and inefficient state management to the overuse of Redux, we cover various potential issues that can arise when using React.js. Developers can create high-quality, performant, and secure React.js applications by knowing these pitfalls and following best practices.
So, if you’re ready to dive into the dark side of React.js, keep reading to learn how to avoid these common pitfalls and create better web applications.
Pitfall #1: Using Too Many Third-Party Libraries
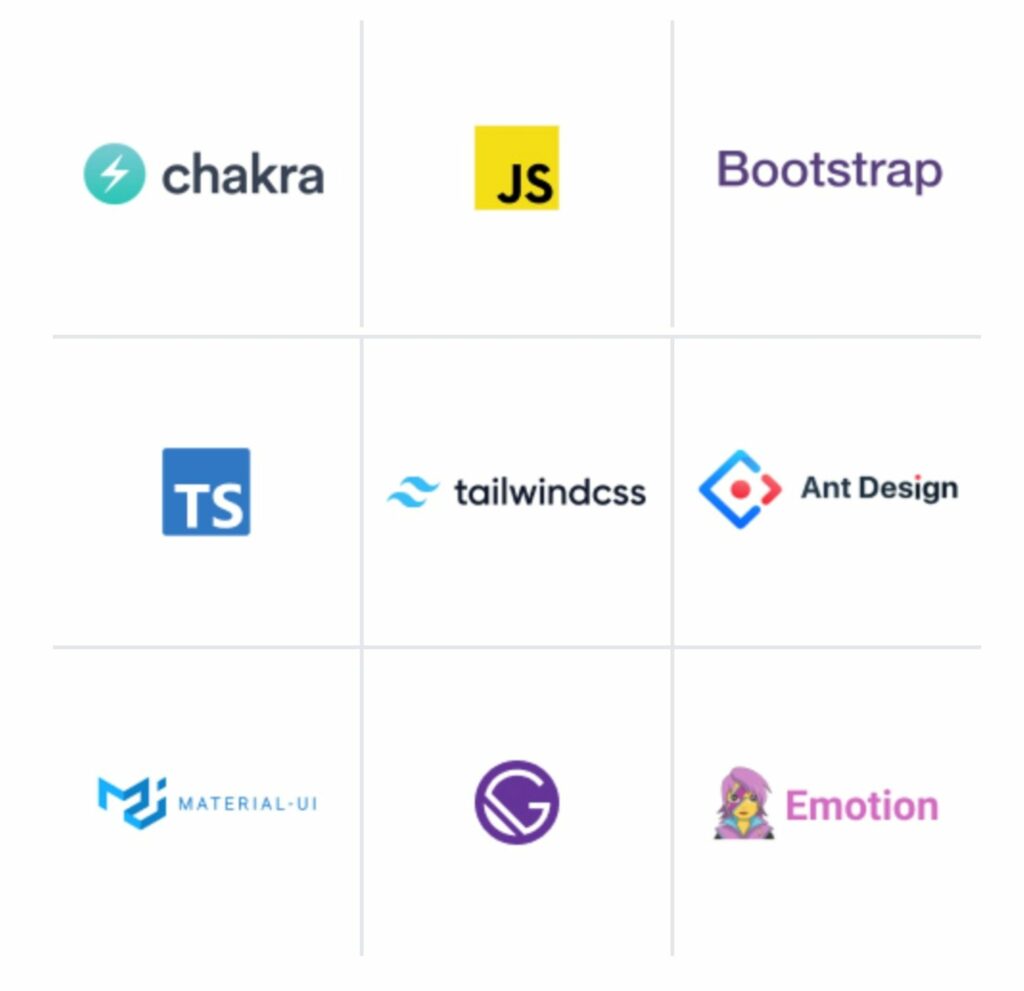
One of the most significant advantages of React.js is the vast ecosystem of third-party libraries that can be used to enhance its functionality. However, it’s easy to fall into the trap of using too many of these libraries, which can negatively affect your application.
For example, let’s say you’re building a simple React.js application that requires basic functionality like handling user input and displaying data. You can add a bunch of third-party libraries to enhance the application, such as a datepicker library, a tooltip library, and a carousel library. While each of these libraries may add some useful features to your application, they also add extra code and complexity that can slow down your application and make it more challenging to maintain.
In addition to performance issues, too many third-party libraries can make your application more vulnerable to security risks. Each library may have vulnerabilities or dependencies, exposing your application to potential attacks.
To avoid this pitfall, it’s essential to be selective when choosing third-party libraries for your React.js application. In this article, we have listed the top component libraries you can use with your React project. Only choose libraries that are essential to your project and have a good track record for performance and security. It would be best to keep an eye on the size of your application and regularly review the libraries you’re using to ensure they’re still necessary.
By taking a cautious approach to using third-party libraries, you can ensure your React.js application is performant, secure, and optimized for success.
Pitfall #2: Poor Component Design
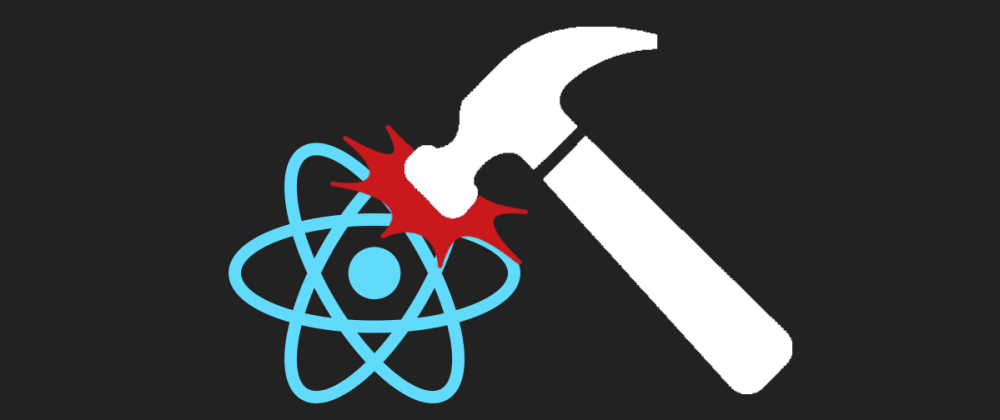
Source: https://dev.to/bornfightcompany/bad-reactjs-practices-to-avoid-a1b
React.js is all about creating reusable components that can be combined to build complex and interactive user interfaces. But, poor component design can lead to a range of problems that can make your application less performant, harder to maintain, and less enjoyable to use.
One of the biggest mistakes developers make when designing components is creating overly complex or tightly coupled components. This can make it difficult to understand how components work together and lead to much repetition in your code. It’s like building a puzzle where each piece is too complicated and can’t fit properly.
Another common issue is the overusing states in your components. While the state is a powerful tool in React.js, too much can lead to performance issues and make your components harder to manage. It’s like having too many gears in your bike, where you can’t find the right one, and the chain keeps slipping.
To avoid these issues, following React best practices when designing your components is essential. This includes keeping your components small and focused on a single task, using props to pass data between components, and avoiding excessive use of state. Think of components as building blocks that are easily understood and work well together, like Lego bricks. Functional components are another way you can ensure that components are lean.
By focusing on good component design, you can create React.js applications that are easier to maintain and more performant. So, take the time to design your components thoughtfully, and watch your application come together like a well-oiled machine.
Pitfall #3: Not Optimizing React Performance
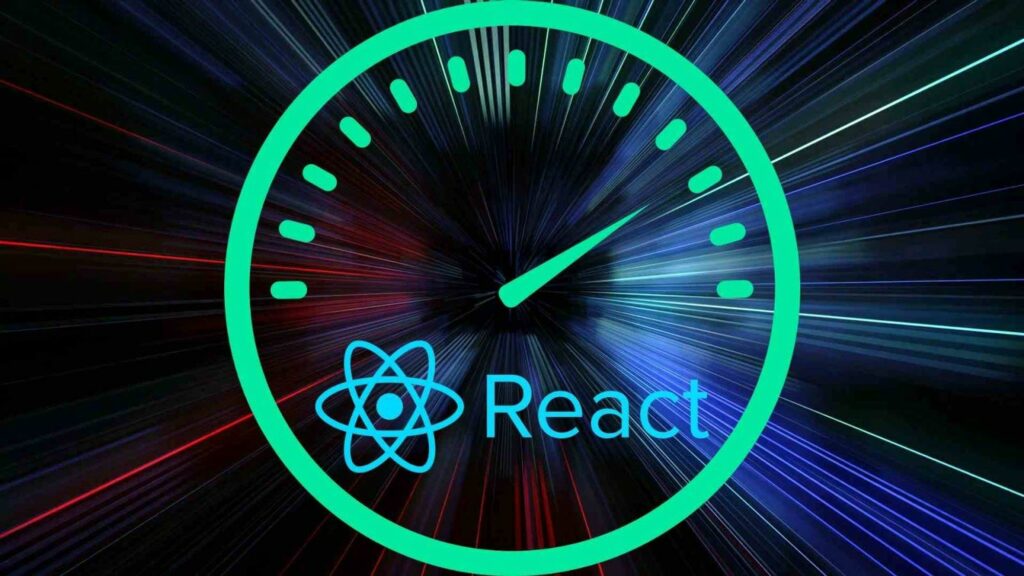
Source: https://www.freecodecamp.org/news/tips-to-enhance-the-performance-of-your-react-app/
Rendering Too Many Components
One of the most common performance pitfalls is rendering too many components or rendering them too frequently. This can slow down your application, making it feel unresponsive and slow to users.
Not Implementing shouldComponentUpdate
Another issue impacting React performance is not implementing shouldComponentUpdate, which allows you to optimize rendering by only re-rendering components when necessary.
Large File Sizes
In addition to rendering issues, your application’s size can significantly impact its performance. Large file sizes can lead to slow load times, which can cause users to lose patience and leave your application. To mitigate this, consider implementing code splitting or lazy loading to only load the components that are necessary at the time.
Measuring and Improving Performance
To avoid these performance pitfalls, measuring your application’s performance and identifying areas that need improvement is essential. You can use tools like the React Profiler and Chrome DevTools to identify slow components and other performance bottlenecks. Regularly reviewing your code to ensure you’re not unnecessarily rendering components is also essential.
By optimizing the React app performance, you can create a fast and responsive user interface that keeps your users engaged and satisfied. So, take the time to optimize your application’s performance, and watch your users enjoy a seamless experience.
Pitfall #4: Inefficient State Management
State management is a critical aspect of building a React application. It’s what allows you to create dynamic user interfaces that respond to user input and update in real time. However, if you don’t manage your state efficiently, it can lead to performance issues, bugs, and other problems.
Using Local Component State for Global State
One common mistake in state management is using local component state for global state. While it might seem like an easy solution at first, it can quickly become unwieldy and difficult to manage as your application grows in size and complexity. Instead, consider using a dedicated state management solution like Redux or MobX.
Overly Complex State
Another pitfall is creating overly complex state structures. This can make it difficult to reason about your application’s behavior and lead to bugs and performance issues. Instead, strive for simplicity and keep your state structures as simple as possible.
Not Using Immutable State
In React, the state is meant to be immutable, meaning it should not be directly modified. Instead, you should create new copies of your state when you need to make changes. Not using an immutable state can lead to bugs and make it difficult to reason about your application’s behavior.
Here’s a quick video about React State Management:
Pitfall #5: Security Vulnerabilities
Security is critical when building any application, and React is no exception. As with any web application, React applications can be vulnerable to various security vulnerabilities.
Cross-Site Scripting (XSS)
Cross-Site Scripting (XSS) is a common vulnerability in web applications, and React applications are no exception. It occurs when an attacker injects malicious code into a web page, which can be executed in a victim’s browser. To prevent XSS attacks in your React application, sanitize all user input and use a Content Security Policy (CSP).
Insecure Data Storage
Another common vulnerability in web applications is insecure data storage. This can occur when sensitive information is stored in plain text or when data is not properly encrypted. Use proper encryption and hashing techniques when storing sensitive data to avoid this vulnerability in your React application.
Poor Authentication and Authorization
Authentication and authorization are critical aspects of any web application and, if not implemented correctly, can lead to significant security vulnerabilities. Use secure authentication and authorization practices in your React application, such as multi-factor authentication and implementing proper session management.
Not Updating Dependencies
Finally, not keeping your React dependencies up to date can leave your application vulnerable to known security vulnerabilities. Make sure to stay up to date with the latest security patches and updates to all of your React dependencies.
You can create a more secure React application by being aware of these common security vulnerabilities and taking steps to mitigate them. So make sure to sanitize user input, use proper encryption and hashing techniques, implement secure authentication and authorization practices, and keep your React dependencies up to date.
Pitfall #6: Poor Performance on Mobile Devices
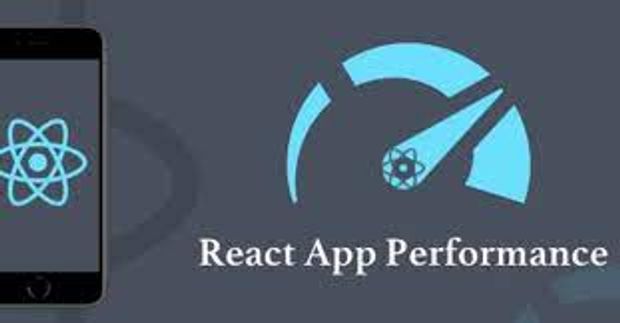
Source: https://www.youtube.com/watch?v=KCr-UNsM3vA
With the increasing popularity of mobile devices, it’s essential to ensure that your React application performs well on these platforms. Here are some common issues that can lead to poor performance on mobile devices.
Heavy Component Trees
React applications can have complex component trees that can impact performance, particularly on mobile devices. The heavier the component tree, the longer it takes for the application to render on a mobile device. To avoid this issue, optimize your component tree and minimize the number of nested components.
Large Images and Videos
Images and videos are often a critical part of modern web applications, but large files can significantly impact performance on mobile devices. To avoid this issue, optimize your images and videos for mobile devices and use responsive images to reduce the load time.
Unoptimized Code
Poorly optimized code can also impact the performance of your React application on mobile devices. Use best practices like code splitting, lazy loading, and server-side rendering to improve performance.
Lack of Testing on Mobile Devices
Testing your React application on mobile devices is essential to identify any performance issues. Not testing on mobile devices can lead to poor user experiences, which can impact the success of your application.
By addressing these common performance pitfalls, you can create a more performant React application that provides an excellent user experience on mobile devices. So optimize your component tree, images, and videos, use best practices for code optimization, and test your application on mobile devices.
Pitfall #7: Over-Rendering Components in React
React is known for its fast rendering capabilities, but over-rendering components can slow down your application’s performance. Here are some common causes of over-rendering and how to avoid them.
Unnecessary Component Updates
React components can render even if their data has not changed. This is because React compares the new state or props with the old state or props using a shallow comparison. If you pass a new object or array to a child component, even if it has the same values, React will consider it as new props, and the component will re-render. To avoid this, you can use React.memo
or shouldComponentUpdate
to optimize the rendering process.
Large Component Trees
React components can also over-render if the component tree is too large. The larger the component tree, the more time React renders and updates the DOM. To avoid this, you can break your components into smaller components and use techniques like lazy loading to optimize your application’s performance.
Costly Rendering Operations
React components can also over-render if they perform costly rendering operations. These include operations that modify the DOM or cause layout changes. To optimize your components’ rendering process, you can use useEffect
and other lifecycle methods to perform expensive operations only when necessary.
By avoiding these common causes of over-rendering, you can optimize your React application’s performance and provide a better user experience.
Pitfall #8: Inadequate Testing
Testing is essential to software development, but it should be noticed when developing React applications. Inadequate testing can result in buggy code, security vulnerabilities, and poor user experience. Here are some common testing pitfalls to avoid when developing React applications.
Lack of Unit Testing
Unit testing tests individual components or functions to ensure they work as expected. Unit tests are essential to React development because they help you catch bugs early in the development process. However, many developers need to write more unit tests or skip them altogether, leading to buggy code and poor user experience. To avoid this, you should write unit tests for each component or function in your React application.
Lack of Integration Testing
Integration testing is how individual components or functions work together to ensure they work as expected. Integration tests are essential in React development because they help you catch bugs that may only appear when multiple components or functions work together. However, many developers need to write more integration tests, leading to unexpected bugs and poor user experience. To avoid this, you should write integration tests for your React application’s critical components and functions.
Lack of End-to-End Testing
End-to-end testing is testing your application’s flow, from user input to output. End-to-end tests are important in React development because they help you catch bugs that may only appear when multiple components or functions work together, and user input is involved. However, many developers need to write more end-to-end tests, leading to unexpected bugs and poor user experience. To avoid this, you should write end-to-end tests for your React application’s critical user flows.
Pitfall #9: Overuse of Redux
Redux is a popular state management library used in many modern web applications. However, it’s essential to use it judiciously and not overuse it. Overusing Redux can lead to unnecessary complexity, bloated code, and decreased performance.
One common mistake is using Redux to manage every state in your application, even when it’s unnecessary. This can lead to an overly complicated state tree and make it difficult to debug issues when they arise. It’s essential to identify which parts of your application truly need Redux and which ones don’t.
Another pitfall is using Redux for simple state changes that could be handled more efficiently with local component states or props. For example, if you have a form with a few input fields, it’s unnecessary to use Redux to manage the state of each input field. Instead, you can use local component states or props to manage the form data.
Overusing Redux can also lead to decreased performance, as every change to the Redux store triggers a cascade of updates throughout the application. This can slow down your application and make it less responsive to user interactions.
To avoid this pitfall, it’s essential to carefully consider which parts of your application truly need Redux and which ones don’t. Use local component state or props for simple state changes and reserve Redux for managing complex, global states. Additionally, optimize your Redux usage by using techniques such as memoization and selectors to improve performance.
Pitfall #10: Not Keeping Up with Updates
React is a rapidly evolving technology with frequent updates and new releases. Failing to keep up with these updates can lead to several pitfalls, including:
Compatibility Issues
As React evolves, changes to the API and internal workings of the library can lead to compatibility issues with other libraries and frameworks. By keeping up with updates, you can ensure your application remains compatible with other technologies in your stack.
Missing Out on New Features
New releases of React often bring new features and functionality that can improve the performance and usability of your application. By failing to keep up with updates, you may miss out on these improvements and fall behind the curve in performance and functionality.
To avoid these pitfalls, it’s crucial to stay up to date with the latest releases of React. This can involve regularly checking for updates and testing new releases in a development environment before rolling them out to production. By keeping up with updates, you can ensure that your application remains secure, compatible, and up-to-date with the latest features and functionality.
As you continue to work with React, consider: what other pitfalls have you encountered or heard of, and how have you addressed them? By sharing knowledge and experience, we can collectively improve our approach to React development and build better applications. Keep exploring, experimenting, and learning, and you’ll be well on your way to mastering the art of React development.