Introduction
Understanding how to handle Javascript async operations in their components can take time for novice developers. It requires a solid understanding of the different strategies available and how to choose the right one for a project. However, choosing the best strategy is essential and makes your application more efficient.
This article will explore how to build a strategy for handling asynchronous operations in React components. We will begin by providing an overview of asynchronous operations and why they are essential in React development. Then, we will dive into the strategies that can be used to handle asynchronous operations in React components, including lifecycle methods, state management libraries, and the async
and await
keywords in JavaScript. We will also provide examples of how each strategy can be implemented in a React application.
Additionally, we will discuss how to determine the appropriate strategy for a given project and the factors to consider when choosing a method. We will also provide tips for selecting the most effective strategy. As well as best practices for handling asynchronous operations in React components. By the end of this article, you will have a solid understanding of how to build a strategy for handling asynchronous operations in their components. Let’s get started!
What are Asynchronous Operations
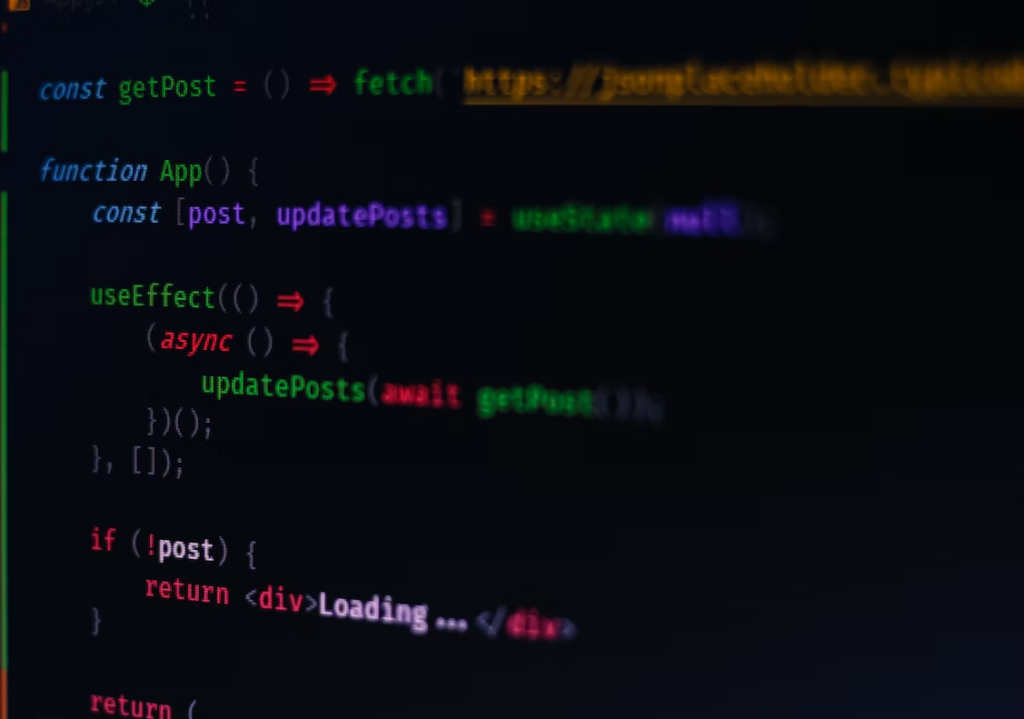
Asynchronous operations are an essential part of building performant and reliable React applications. They allow the application to continue running while waiting for a response from an external source or completing a long-running task. This helps to improve the performance and user experience of the application by keeping the UI responsive and reducing the risk of freezing or crashing.
You may encounter several different types of asynchronous operations in React development. Some common types include:
- Network requests: Sending and receiving data from a server or other external source using libraries such as
axios
orfetch
- Timers: Waiting for a specified amount of time before taking action using JavaScript’s
setTimeout
andsetInterval
functions - Promises: Handling the result of an asynchronous operation using JavaScript’s
Promise
object - Web Sockets: For real-time communication between the application and a server
Asynchronous operations in React components
Asynchronous operations in React components refer to any action that is not executed immediately and waits for an external event or a response to complete. These operations are an essential aspect of React development as they allow the application to continue running while waiting for a response from an external source. Asynchronous operations help improve the performance and user experience of a React application by keeping the UI responsive. This reduces the risk of freezing or crashing.
When handled properly, asynchronous operations can positively impact the performance and user experience of a React application. For example, if an asynchronous operation is not appropriately canceled when a component is unmounted, it can lead to memory leaks and other performance issues. Similarly, suppose the application’s state is not updated correctly after an asynchronous operation is completed. In that case, it can result in the UI needing to be updated correctly or showing incorrect information. It is important to understand the different types of asynchronous operations to choose the appropriate strategy for handling them. By understanding how these operations impact a React application’s performance and user experience, you can handle these operations correctly and deliver optimal performance. Now, how do you choose the best strategies?
Strategies for handling asynchronous operations in React components
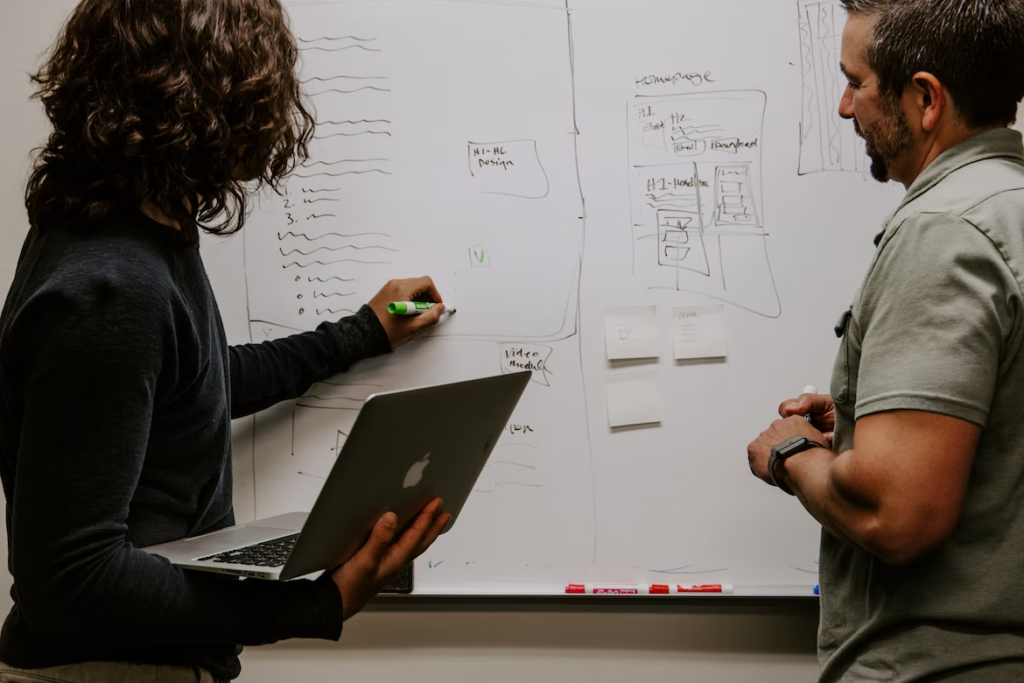
Several different strategies can be used to handle asynchronous operations in React components. Each strategy has its benefits and limitations, and the appropriate method will depend on the project’s specific requirements. We discuss some of the common techniques used.
The Lifecycle method approach
React provides a set of lifecycle methods that can be used to initiate and cancel asynchronous requests. These methods include componentDidMount
and componentWillUnmount
. By initiating an asynchronous request in componentDidMount
, and cancelling it in componentWillUnmount
, you can ensure that the request is only made when the component is mounted and is canceled when the component is unmounted. This approach is simple and easy to understand, but it can become challenging to manage when dealing with complex components with many asynchronous requests.
Here is an example of how to implement the lifecycle method approach in a React component:
Copy code
class MyComponent extends React.Component {
state = { data: null };
async componentDidMount() {
const data = await getData();
this.setState({ data });
}
componentWillUnmount() {
this.setState = null;
}
render() {
return <div>{this.state.data}</div>;
}
}
Here’s a 2-minute video explaining the lifecycle method in React:
The State management library approach
State management libraries such as Redux and MobX can manage the state of asynchronous requests. Using a centralized store to manage the state of the application makes it easier to manage the state of asynchronous requests and ensure that the UI is updated correctly. However, the added complexity of a state management library can make it harder to understand and debug.
Here is an example of how to implement the State management library approach using Redux:
Copy code
class MyComponent extends React.Component {
render() {
return <div>{this.props.data}</div>;
}
}
function mapStateToProps(state) {
return {
data: state.data
};
}
function mapDispatchToProps(dispatch) {
return {
getData: () => dispatch(getData())
};
}
export default connect(mapStateToProps, mapDispatchToProps)(MyComponent);
If you’re unsure which state management library to choose, watch this quick video that goes through each one:
The Async-await approach
The async
and await
keywords in JavaScript can be used to handle asynchronous operations in a more synchronous, linear way. This can make the code easier to read and understand, but it can also make it more difficult to handle errors and cancel asynchronous requests.
Here is an example of how to implement the Async-await approach:
Copy code
function MyComponent() {
const [data, setData] = useState(null);
const handleClick = async () => {
const data = await getData();
setData(data);
};
return (
<div>
<button onClick={handleClick}>Get Data</button>
{data}
</div>
);
}
Watch this quick YouTube tutorial on how to use JavaScript Async Await:
It is important to weigh the benefits and limitations of each approach and choose the one most appropriate for your project. Additionally, as the React ecosystem is continuously developing, new features such as hooks and context API could also be used to handle asynchronous operations more elegantly.
Choosing the right strategy for your project
When choosing the right strategy for handling asynchronous operations in React, consider these facts:
Performance requirements
The performance of your application is one of the most important factors to consider when choosing an asynchronous operations strategy. Suppose your application needs to handle many concurrent requests or perform complex calculations. In that case, it may be more appropriate to use a state management library or the async
and await
keywords to ensure that the application can handle the load and perform optimally.
Complexity
If your application is small and simple, the lifecycle method approach may be sufficient. However, if your application is large and complex, using a state management library or the async
and await
keywords can help to manage the complexity and make the code easier to understand and maintain.****
Developer preference
It is also important to consider the developers’ preferences working on the project. For example, suppose the developers are more comfortable with the lifecycle method approach. In that case, it may be more appropriate to use this approach, even if another direction would be more appropriate from a technical standpoint.
Additionally, here are some tips for determining the most effective strategy for your project:
- Understand the problem: Before selecting a strategy, you must understand the problem you are trying to solve. This will help you choose a strategy best suited to the issue at hand.
- Experiment: Try out different strategies and compare the results. You will better understand each approach’s benefits and limitations, and it will help you choose the most appropriate method for your project.
- Keep it simple: Avoid using complex libraries or frameworks unless they are necessary. Keep your code simple and easy to understand. This will make it easier to debug and maintain in the long run.
- Remember the best practices: Follow best practices for handling asynchronous operations in React components. This will help you to choose the strategy that is most likely to be successful.
Choosing the right strategy for handling asynchronous operations in React components can be a challenging task. Still, by considering the above factors, you can ensure that their applications can handle asynchronous operations effectively, thus delivering optimal performance and user experience.
Best practices for handling asynchronous operations in React components
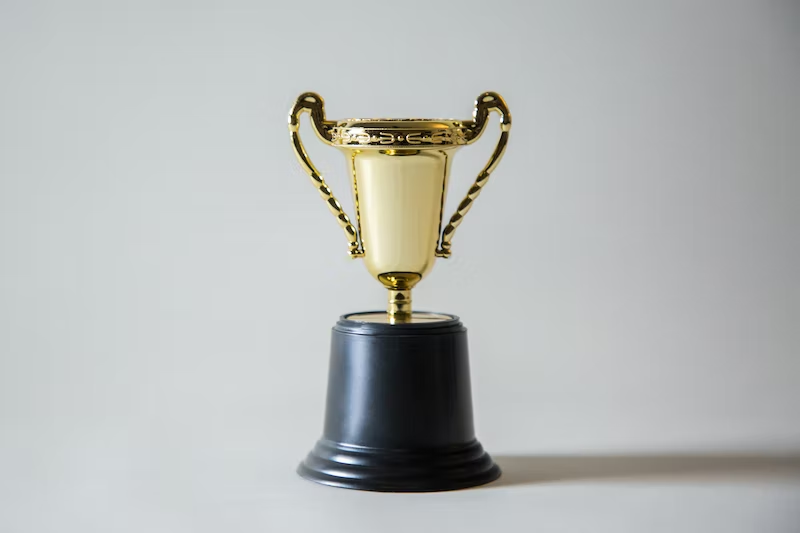
By following best practices, developers can make applications that handle asynchronous operations effectively, deliver optimal performance, and provide good user experience. One of the most important practices is to optimize your asynchronous operations.
Optimizing Asynchronous Operations
Optimizing asynchronous operations is crucial for building performant and reliable React applications. It can improve the application’s performance by reducing the number of requests made to the server, caching the results of requests, or optimizing the render performance. This can lead to better user experience by reducing the time it takes for the application to respond to user input or by ensuring that the UI is always up-to-date. Additionally, it can reduce the number of resources the application uses and make the codebase easier to understand and maintain. This can help developers work more efficiently and produce more reliable code. Overall, developers can create a more seamless and responsive user experience by optimizing asynchronous operations while ensuring that the application performs optimally.
Here are some tips for optimizing asynchronous operations in React applications:
- Minimize the number of asynchronous operations that are performed by consolidating multiple requests into a single request, or by caching the results of previous requests.
- Use
debouncing
orthrottling
when handling events that are triggered frequently like scrolling or typing in a form field. - Use
requestIdleCallback
andsetTimeout
with a small delay to perform non-critical operations in an efficient way. - Use caching: Use caching techniques to reduce the number of requests made to the server by using browser cache, in-memory cache, or a caching library like**
memoize-one
**
Best Practices Checklist
Aside from optimization, there are other ways to ensure you are handling asynchronous operations efficiently.
- Use libraries: Use libraries such as
axios
orfetch
to handle network requests, rather than using the nativeXMLHttpRequest
orfetch
APIs. This can make it easier to handle errors and optimize performance. - • Handle errors: Use proper error handling techniques to ensure that the application can recover gracefully from errors. This can be achieved by using try-catch blocks or handling errors with promises.
- Debuggability: Ensure that the codebase is easy to debug by using proper naming conventions, logging, and debugging tools.
- Test: Use automated testing to ensure that the application can handle asynchronous operations correctly. By writing tests for the different parts of the application that handle asynchronous operations, you ensure the application handles different scenarios correctly.
- Optimize updates: Optimize the number of updates made to the UI by avoiding unnecessary re-renders or optimizing the render performance. This can be achieved by using
shouldComponentUpdate
,React.memo
, anduseMemo
hooks. You can learn how to use React.memo with this excellent guide.
Conclusion
In conclusion, handling asynchronous operations in React components is essential to building performant and reliable React applications. Developers must have a solid understanding of the different strategies available and choose the right one for a given project. Asynchronous operations can significantly impact the performance and user experience of a React application. Thus, it’s crucial to handle them correctly.
In this article, we have explored the strategies that can be used to handle asynchronous operations in React components, including lifecycle methods, state management libraries, and the async
and await
keywords in JavaScript. We have also discussed how to determine the appropriate strategy for a given project and the factors to consider when choosing a method. Additionally, we discussed the best practices for handling asynchronous operations in React components, including tips for optimizing asynchronous operations in React applications and error handling, debugging, and testing.
By following the strategies and best practices outlined in this article, you can build reliable and performant applications that can handle asynchronous operations effectively. Building reliable performing applications can take time and effort, and revising and editing layout code can be tedious. To speed this process up, you can use CopyCat, a design-to-code tool that generates code with the click of a button. It will speed up your development and bring your production to the next level.
And keep in mind that new features and tools will continue to develop in the React ecosystem, so stay up-to-date and evaluate which tools are best suited for your specific use case.