- Introduction to React Chrome Extensions
- Creating A New React APP
- What is a React Chrome Extension
- 1. React Developer Tools
- 2. Redux DevTools
- 3. React Context DevTool
- 4. React Performance Devtool
- 5. React VT
- 6. HTML To React
- 7. Website HTML To React
- 8. Reactime
- 9. React Quantum
- 10. Realize for React
- 11. React Stores Devtools Extension
- 12. Jinno: Design any website React/HTML/sketch
- 13. React Repositories New Tab
- Wrapping Up
Introduction to React Chrome Extensions
Google developed Google Chrome (or just Chrome), a popular internet browser. One of the reasons Chrome browser is the most popular browser for web development is that it has Chrome developer tools out of the box and allows third-party dev tools to be installed to the browser, to provide more capabilities for web development aka React Chrome extension.
Developers call Chrome third-party dev tools extensions, which allow developers to detect what’s wrong with their app in a development environment. You can install all Chrome DevTools from the Chrome extension marketplace.
Similarly, Figma allows users to download and use Figma plugins on the tool itself. CopyCat is a Figma to React tool that converts Figma design to React code with the click of a button. Eliminate messy handoffs with production ready code and speed up development by focusing your time solving challenges you want.
Creating A New React APP
React is a JavaScript UI library for developing visual components for single-page applications.
To follow along properly and test out how some of these extensions work. Create a new React project with the command below:
npx create-react-app my-react-project
The command above will create a new project named my-react-project using Create React APP CLI. If you don’t know what a Create React App CLI is, you should check this guide.
We’ll use the newly created project to experiment with all the React Chrome developer tools we’ll explore in this article.
Start your React app with the command below:
npm run start
A new tab will be opened on your browser and our React app page should look something like this:
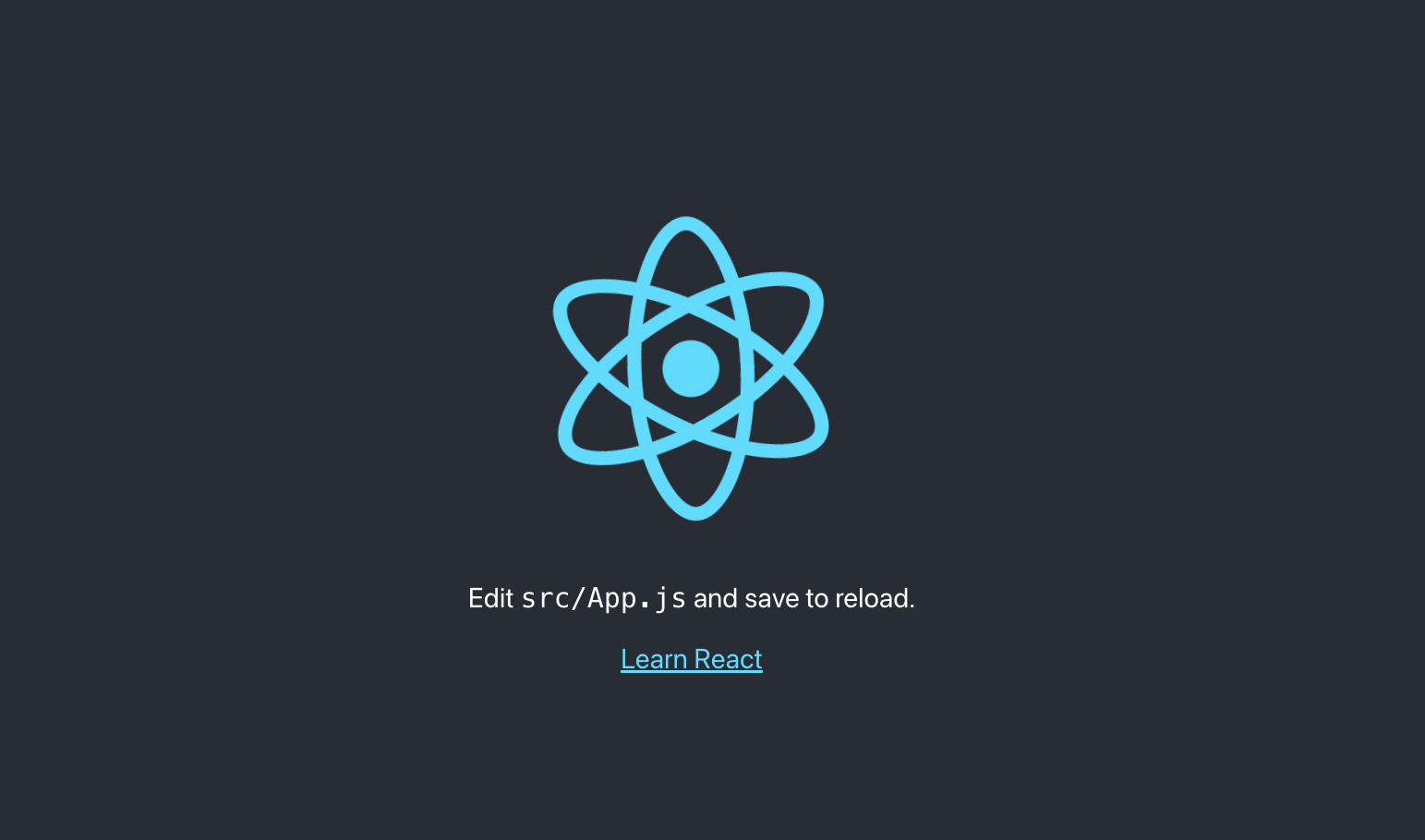
What is a React Chrome Extension
A React Chrome extension is a developer tool (or DevTools) that is dedicated to developing and maintaining a React app. They’re useful for debugging and inspecting React components, States, Redux, Context API, React DOM elements, etc.
Listed below are the top 13 React Chrome extensions that boost your productivity as a React developer. Maybe you’ll find your favorite chrome extension here.
1. React Developer Tools
The first extension on the list is the React developer tools. It’s the official Chrome DevTools extension for the open-source React JavaScript library, allowing you to inspect and visually analyze the React component hierarchies in the Chrome Developer Tools.
Facebook React Team maintains the React developer tool extension.
How to Install React Developer Tools Extension?
Below is a quick guide on how to install the React developer tools. This step will be used in installing any other React dev tool from the Chrome Webstore.
- Visit the Chrome extension marketplace
- Search for React developer tools and click the “Add to Chrome” button to install the React DevTools extension:
- Click on the “Add extension” button from the popup:
- You’ll get this popup notification after a successful installation:
This tutorial uses the Chrome extension 4.24.7 version and yours might be a little different depending on the version you have.
After installing the React developer tools, two new tabs will be added to your Chrome DevTools, as illustrated below: the tabs” ⚛️ Profiler” and “⚛️ Components.”
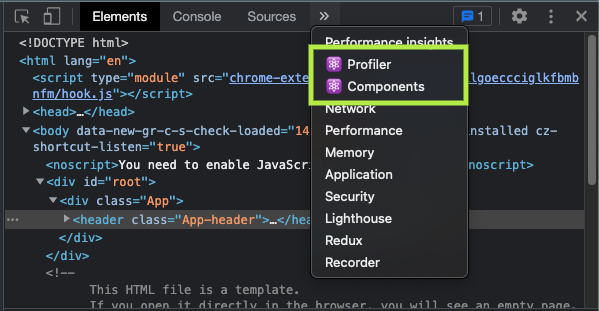
You’ve learned how to install a Chrome extension in this section. We’ll go over how to make use of the React DevTools tab ‘components’ in the next section.
How To Use The React Developer Tools Components Tab
The Components tab displays the React root components that were rendered on the page, as well as the child components that are rendered within the root components.
Let’s say you have a Button.jsx component that accepts a text prop, with the following:
import React from "react";
export const Button = ({ text }) => {
return <button>{text}</button>;
};
Then import it inside of your <App /> component. You should have something like this on your page:
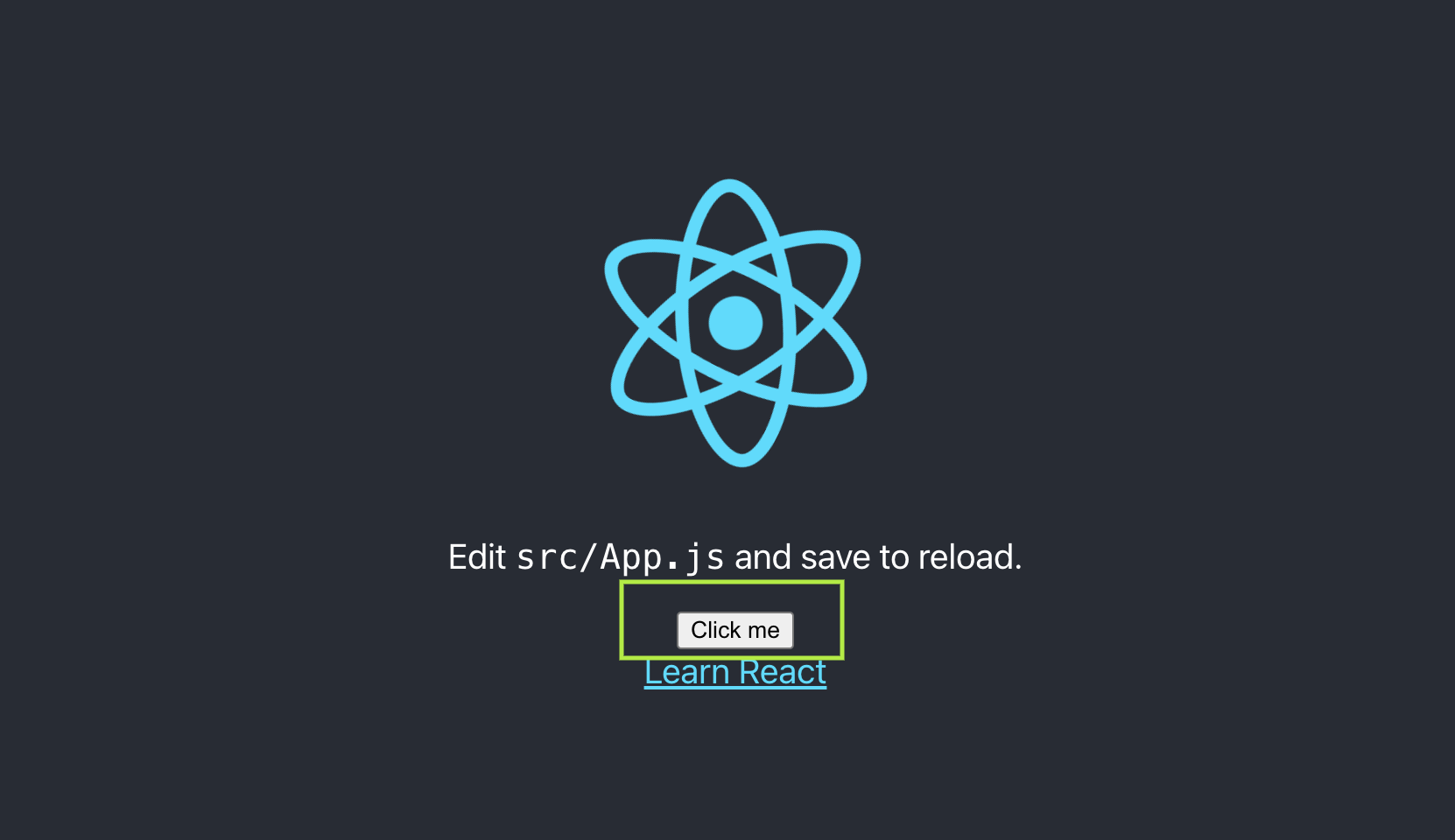
You can inspect your React page component tree by navigating to your Chrome React DevTools Components tab, as illustrated below:
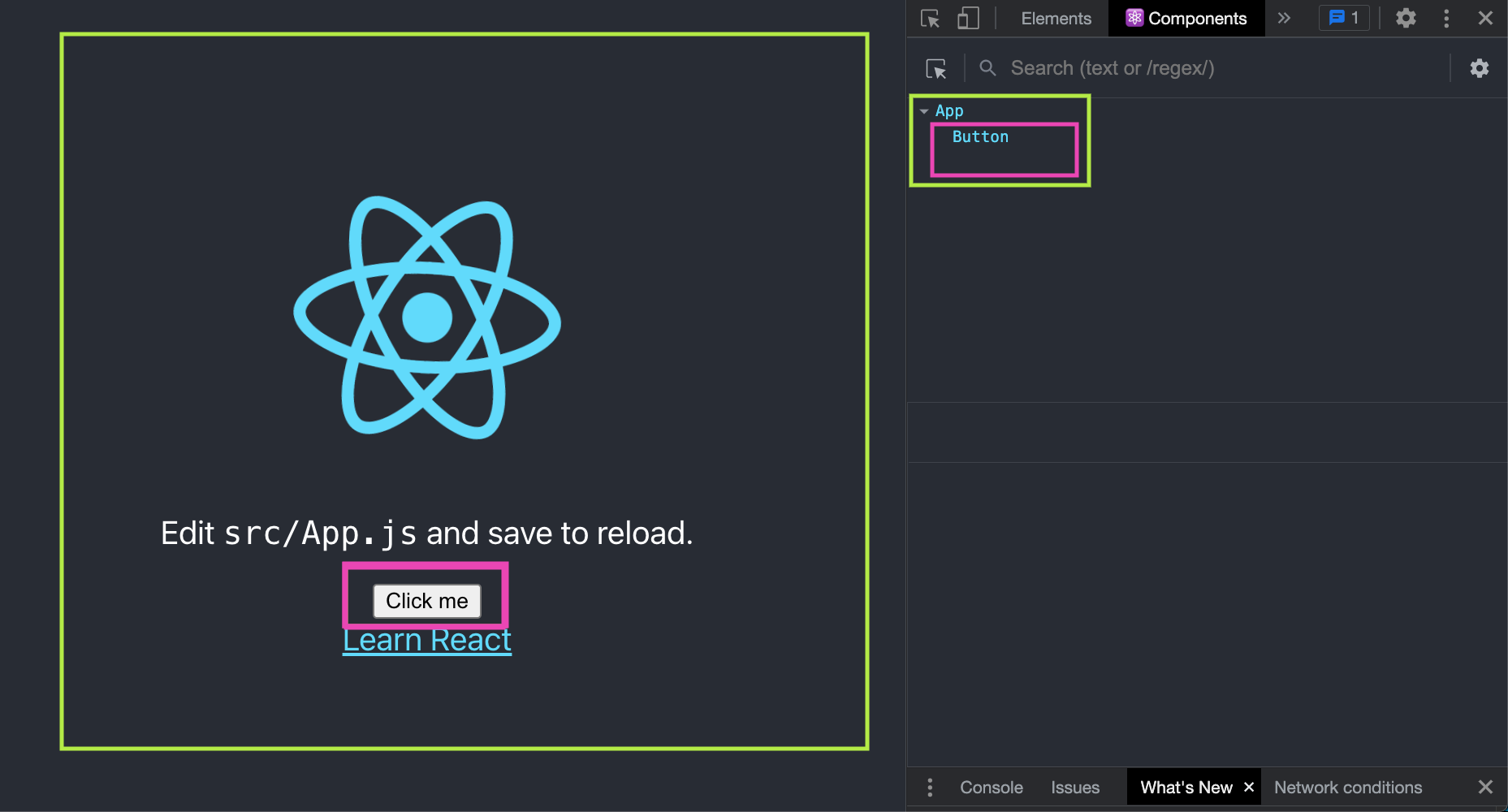
From the illustration above, the tab shows that we only have two React components rendered in our React dom: the App component and the Button component that we created.
By selecting one of the components in the tree, we can easily inspect and edit any of their current props and state in the panel on the right, as demonstrated below:

You can also inspect the selected component (i.e the Button component) to know which component rendered it (i.e the App component rendered the Button component), the component that created the App component, and the version of the react-dom you’re using.
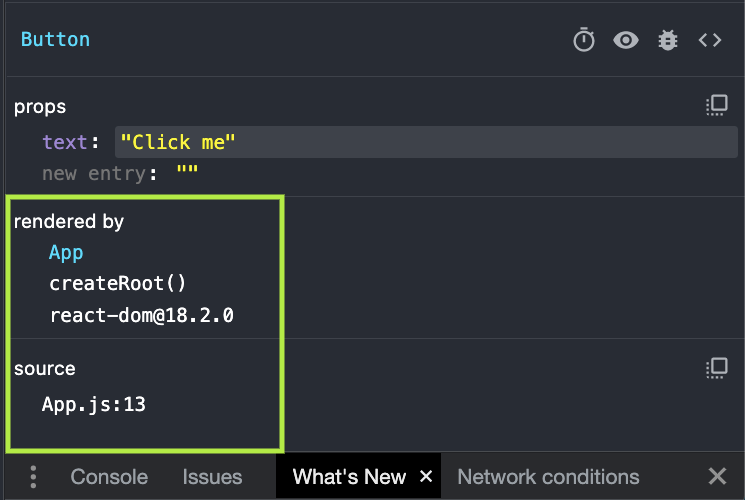
How To Use The React Developer Tools Profiler Tab
The Profiler tab is the second feature you’ll find in the React devtool. It allows you to record the performance and detect issues in your React app.
According to the React documentation – “The profiler tab plugin uses React’s experimental Profiler API to collect timing information about each component that is rendered to identify performance bottlenecks in a React application”.
To see how Profiler Tab works, update your Button component with the following code:
import React from "react";
export const Button = ({ text, handleClick }) => {
return <button onClick={() => handleClick()}>{text}</button>;
};
The Button component above accepts two props, the text which is the button text, and the handleClick which is a function that’ll be executed when the button is clicked.
Next, update your App.js file with the following:
import React from "react";
import "./App.css";
import { Button } from "./components/Button";
function App() {
const [count, setCount] = React.useState(0);
const handleClick = () => {
setCount(count + 1);
};
return (
<div className='App App-header'>
<p> {count} </p>
<Button text={"Click me"} handleClick={handleClick} />
</div>
);
}
export default App;
From the code above, the handleClick function increases the count state by 1 each time the button is clicked:

On the Profiler tab, click on the start recording icon:
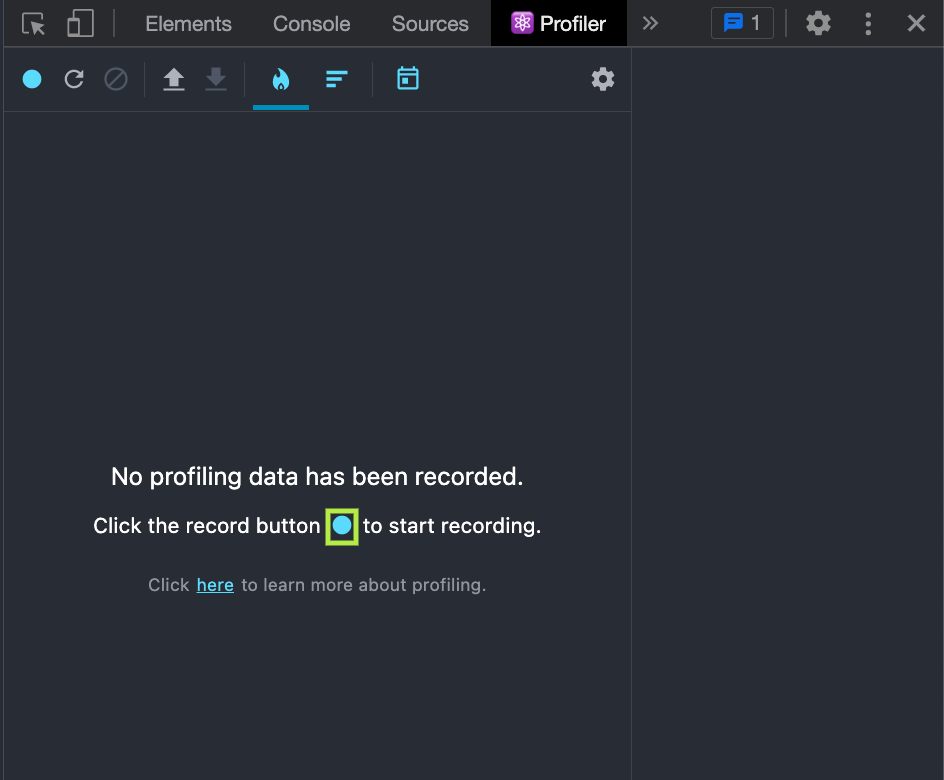
Once the Profiler has started recording, React DevTools will automatically collect the performance information of each component each time your application renders.
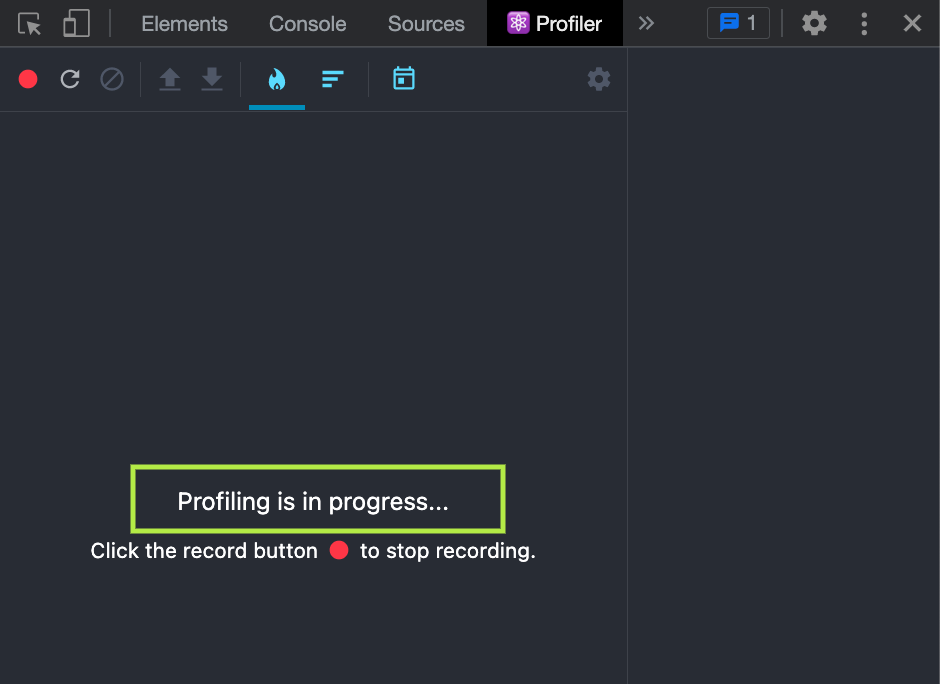
To make our component rerender, click the button up to 5 times (or make your component be re-rendered once or more). Click the stop recording icon “🔴” when you’re done.
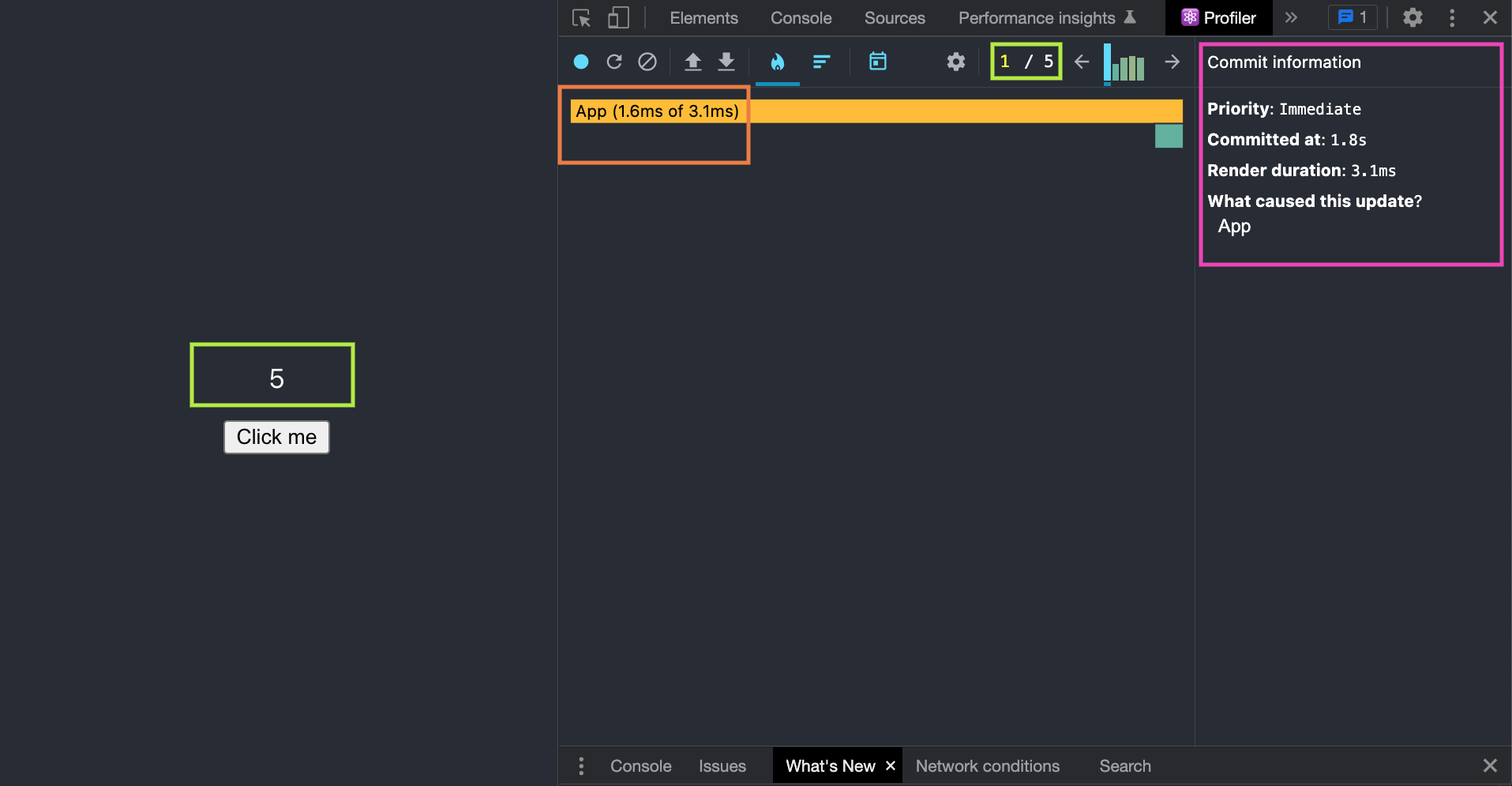
Generate a Visual Report
The Profiler tab will generate a visual report indicating the number of times your components are re-rendered and the duration it takes for each render (i.e we clicked the button 5 times, and the Profile tab detects the render time for each of the clicks).
The first click took 3.1ms to render:
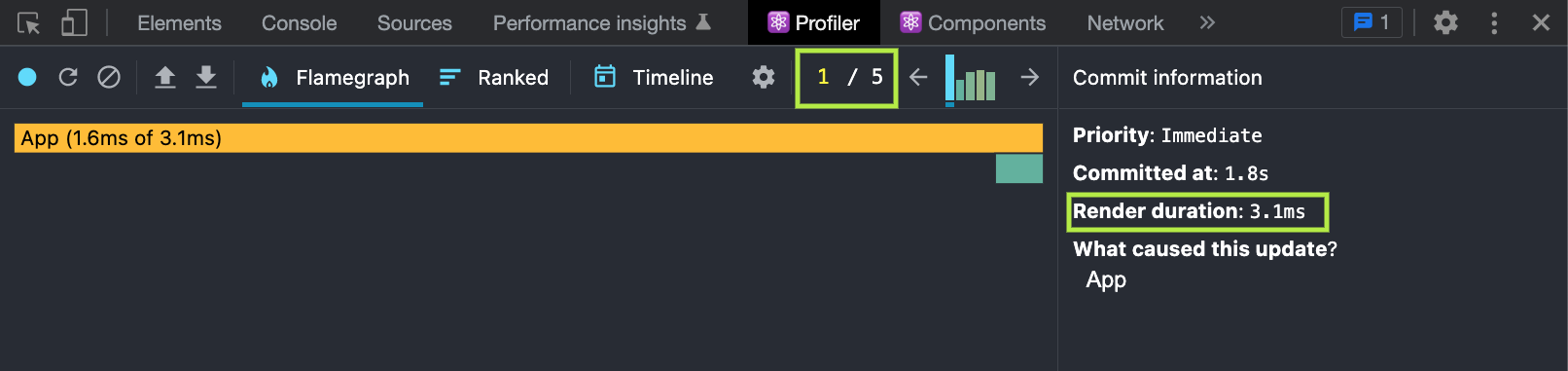
While the 5th click took 0.7ms to render:
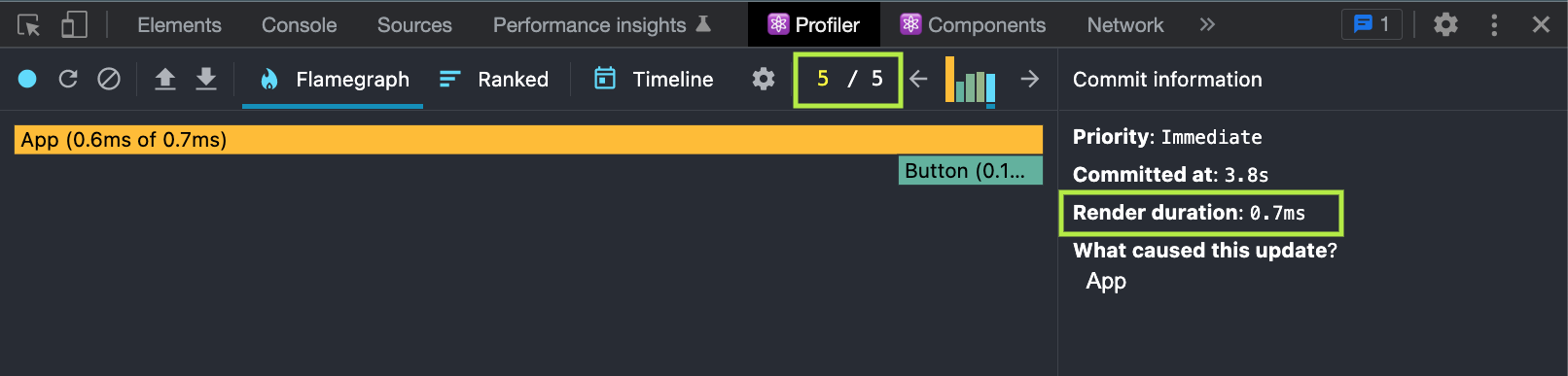
This makes more sense in a robust React application when the time complexity is crucial.
You can learn more about the React Developer Tools “⚛️ Profiler tab” from the official React documentation here.
You can also learn more about React Developer Tools. How to use the “⚛️ Profiler tab” and the “⚛️ Components tab” from the video below:
2. Redux DevTools
As a React developer using React Redux for state management, this is a must-have extension for you to have installed as one of your React Chrome developer tools or as a standalone app. Redux DevTools enables you to monitor triggered actions and their effect on your Redux state.
You can install the Redux DevTools extension from the Chrome Webstore here.
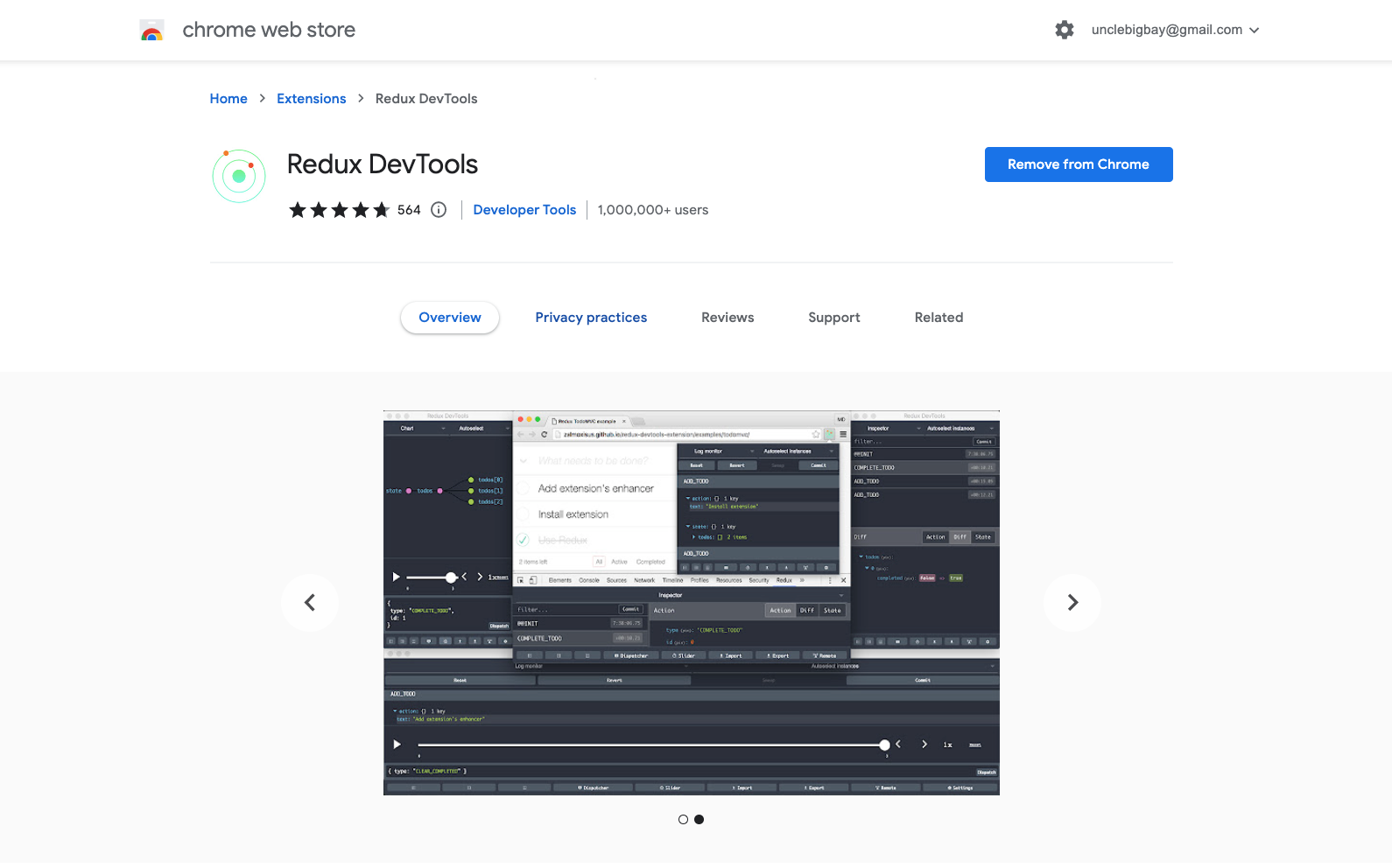
And install it into your project with the single command line below:
npm install --save redux-devtools-extension
Redux DevTools extension allows you to do the following in your React app:
- Persist Redux store on page reload
- Inspect every state and action payload in your Redux store.
- Keep track of all changes in your Redux state
- Keep track of Redux actions and their effects on states
- Pause or cancel Redux actions
- Redux Devtools allows you to reply to any previous actions
A typical Redux DevTools looks something like this:
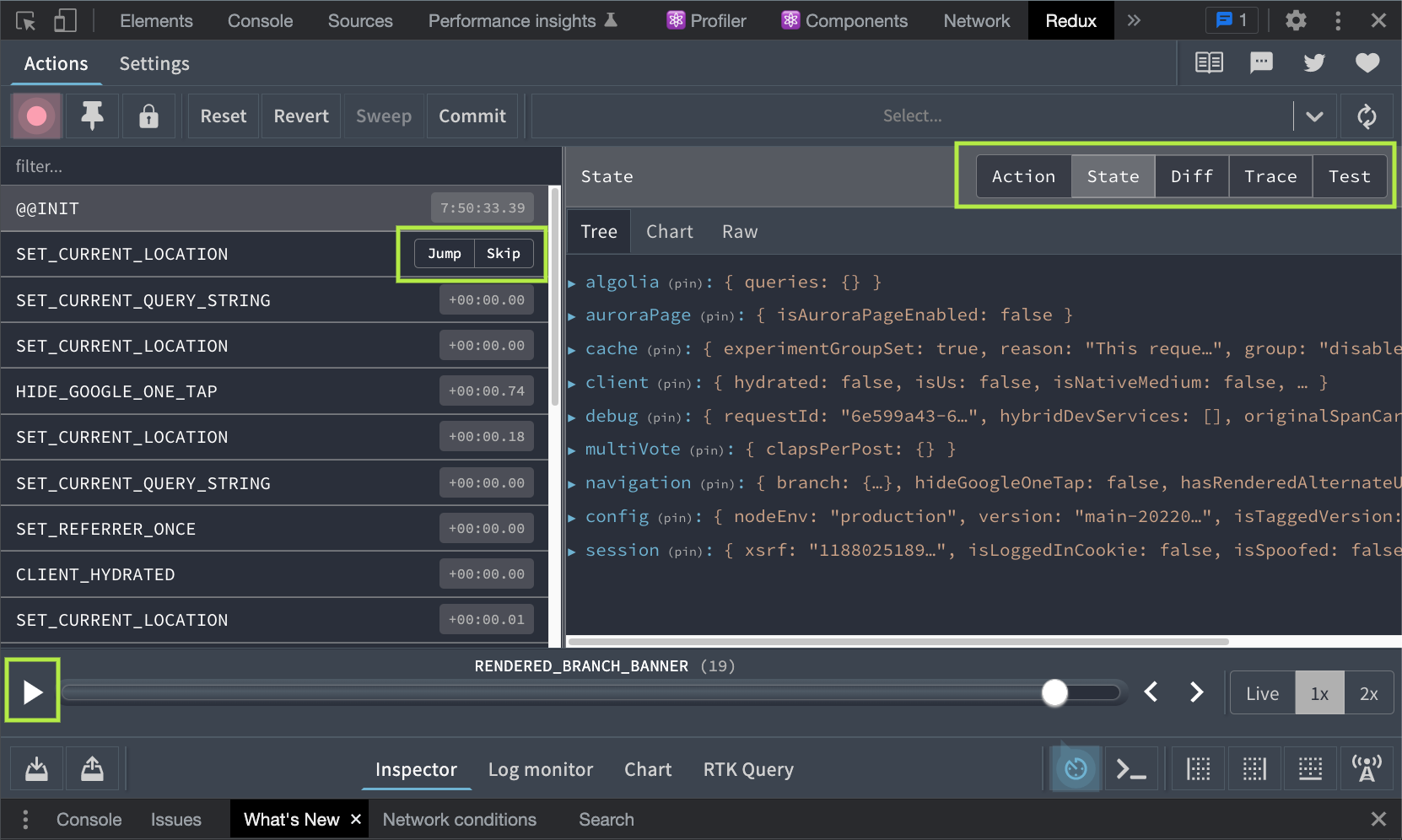
You can learn more about using the React-Redux Developer tools from the video below:
3. React Context DevTool
Similar to React Redux, context API is used for managing states in a React app, you can learn the difference here.
Users use React Context DevTool to debug and keep track of state changes in React Context and useReducer API.

You can install the React Context DevTool extension from the Chrome Webstore here (This extension requires you to have the React Developer Tools installed on your browser).
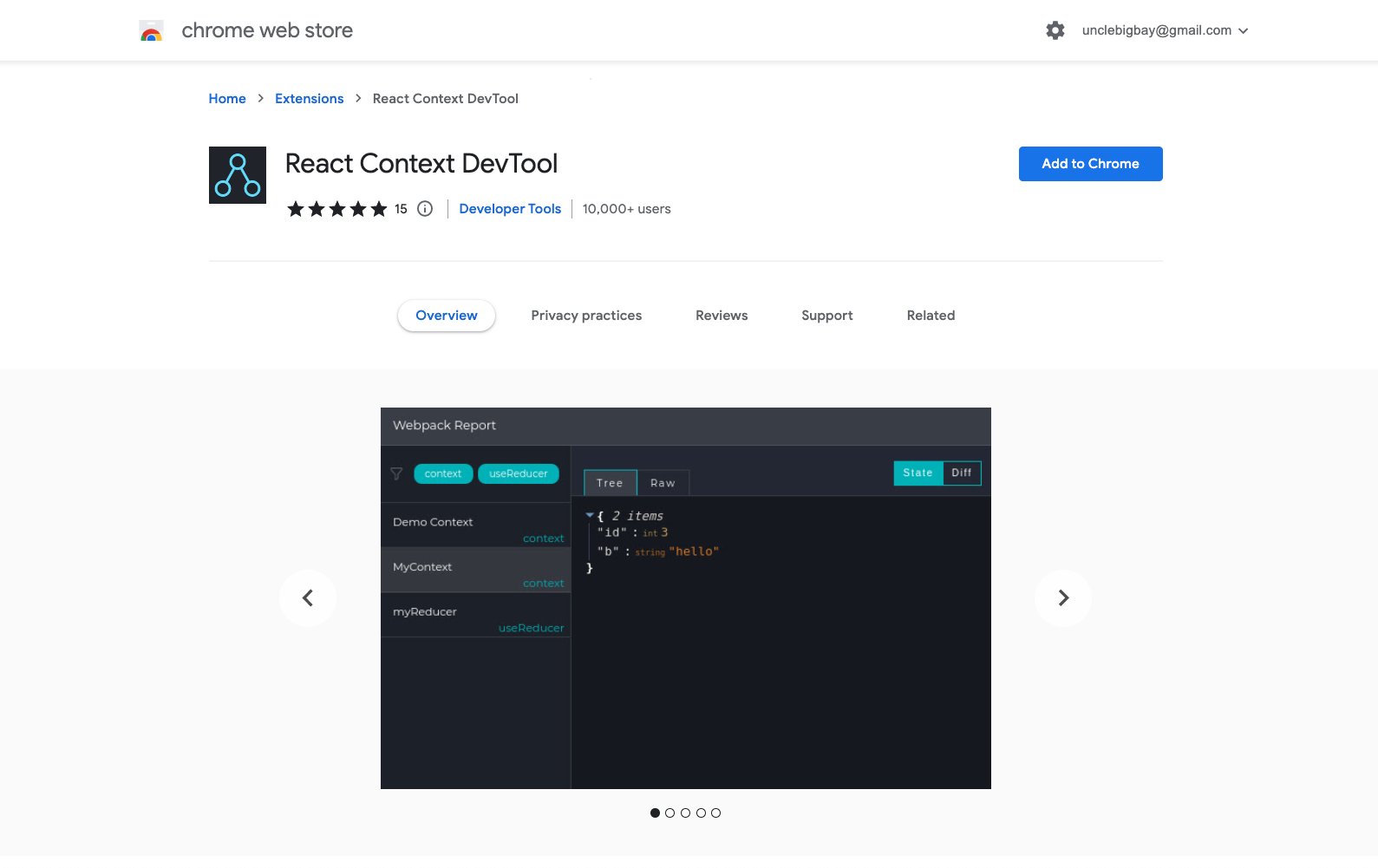
React Context DevTool allows you to do the following in your project:
- Inspect every state and action payload in your Context API store.
- Keep track of all state changes in your Context API
- Keep track of actions and their effects on states in Context API
4. React Performance Devtool
The React Performance Devtool focuses on diagnosing why React components are not performing how they’re expected to and why they take a longer time to load.
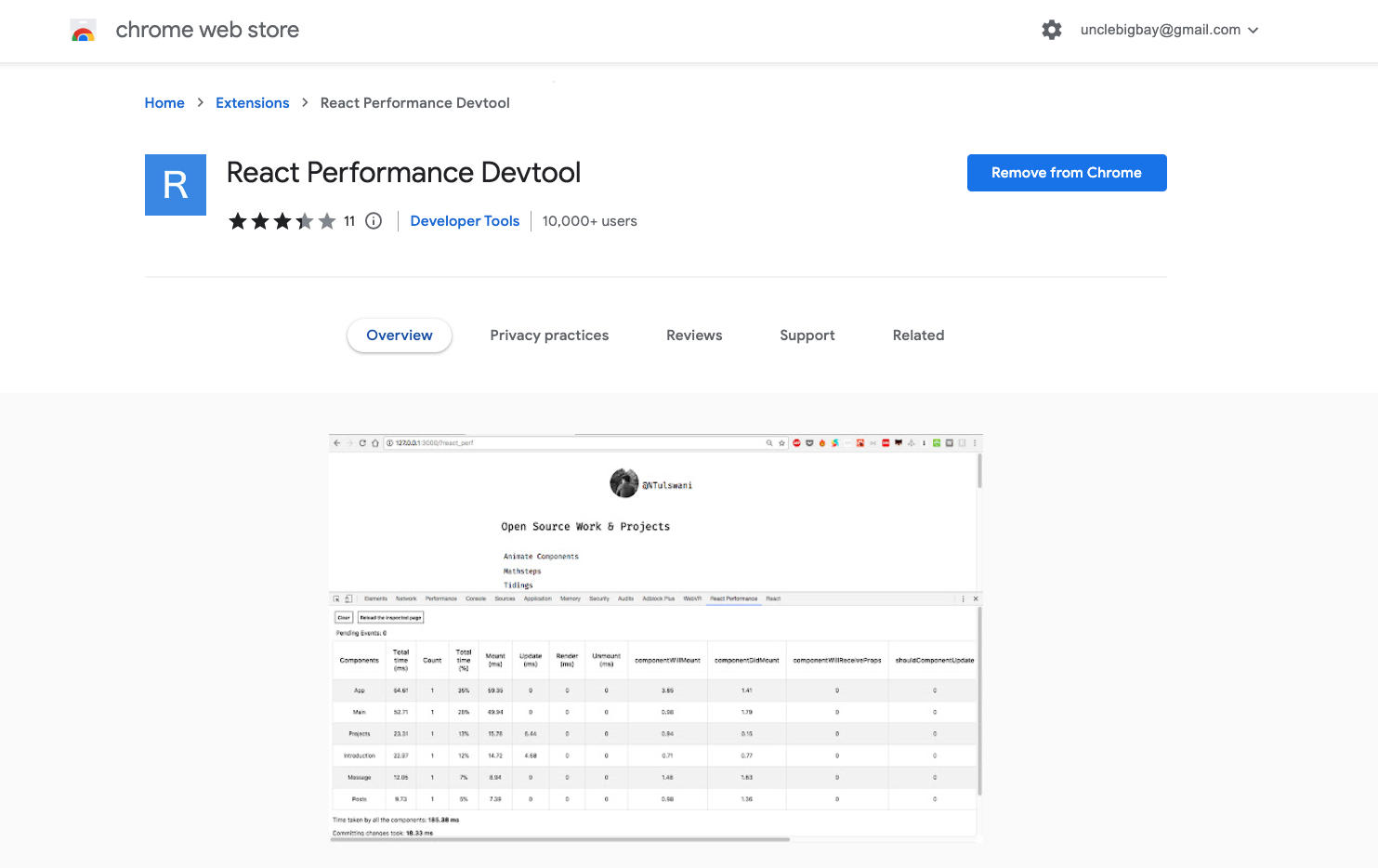
React Performance Devtool helps you:
- Remove or unmount unused components in your React apps.
- Inspect what is blocking or taking more time after an operation has been started.
- Examine which components are taking more time to load.
Below is the official demo of how to use React Performance Devtool:

5. React VT
React VT is a visual component testing tool for React apps. It presents a live view of your React component structure, along with its current state and props.
You can install the React VT from the Chrome Webstore here.
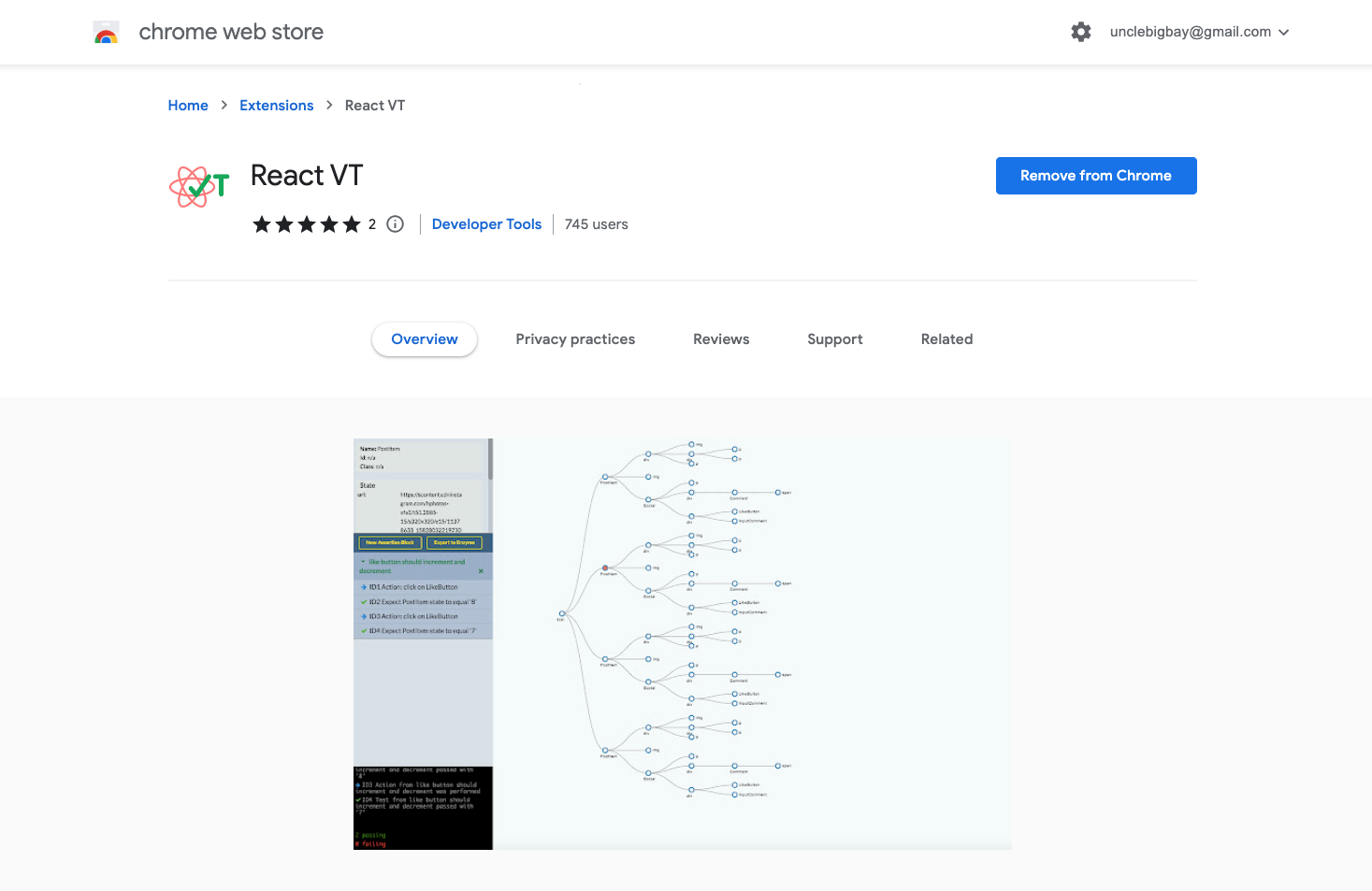
After successfully installing the React VT extension, check this installation guide to set up the package in your React app.
Below is the official demonstration of React VT in a React app:

As demonstrated above, you can declare assertions and test them in real-time while interacting with your React applications. Once you’re satisfied with the defined tests, you can export the assertions into an Enzyme file and use it as a basis to start writing your Enzyme tests. What is Enzyme?.
6. HTML To React
HTML to React allows you to convert HTML tags to JXS directly from your browser. You can use this extension to easily auto-generate a class or functional component by copy-pasting an HTML code snippet inside the text area.
See the demo below:

The extension also helps convert HTML attributes such as class to className JSX attributes in camelCase, as shown below:

You can install the HTML to React extension from the Chrome Webstore here:

7. Website HTML To React
As the name implies, the HTML to React extension helps you to convert any selected website element into a React component.
After installing the Website HTML TO React extension from Chrome Webstore:
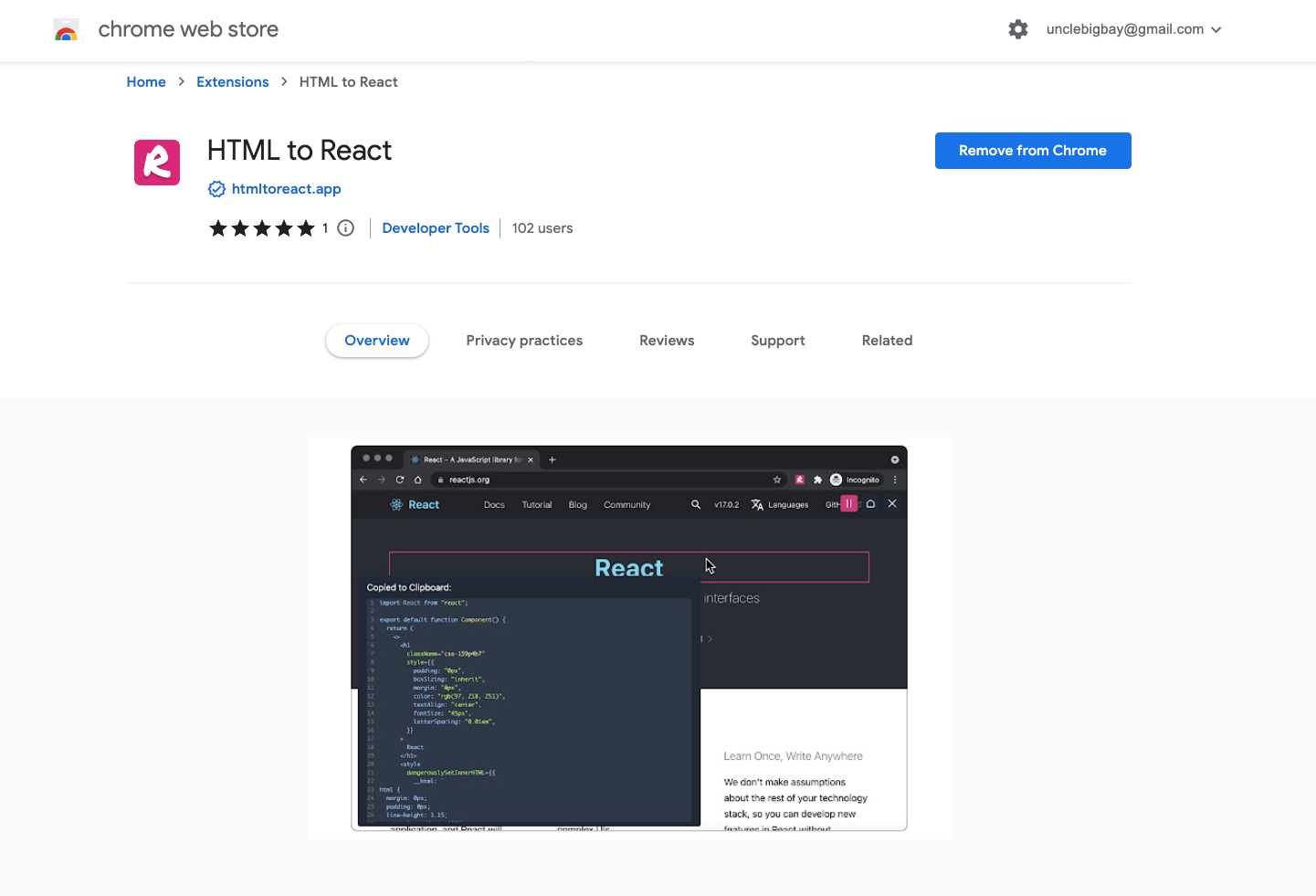
Navigate to the website that you wish to inspect and convert to a React component:

As demonstrated above:
- Firstly click on the Website HTML to React extension icon to start
- Click the play button to start inspecting any visual design components on the website.
- The modal window displays the actual auto-generated code and auto-copies to your clipboard. Use Ctrl. + V to paste the code snippet into your React source code.
8. Reactime
Reactime is an open-source React Chrome developer tool for time travel debugging and performance monitoring in React applications by memorizing the state of components each time they render.
You can install the Reactime extension from the Chrome Webstore here.
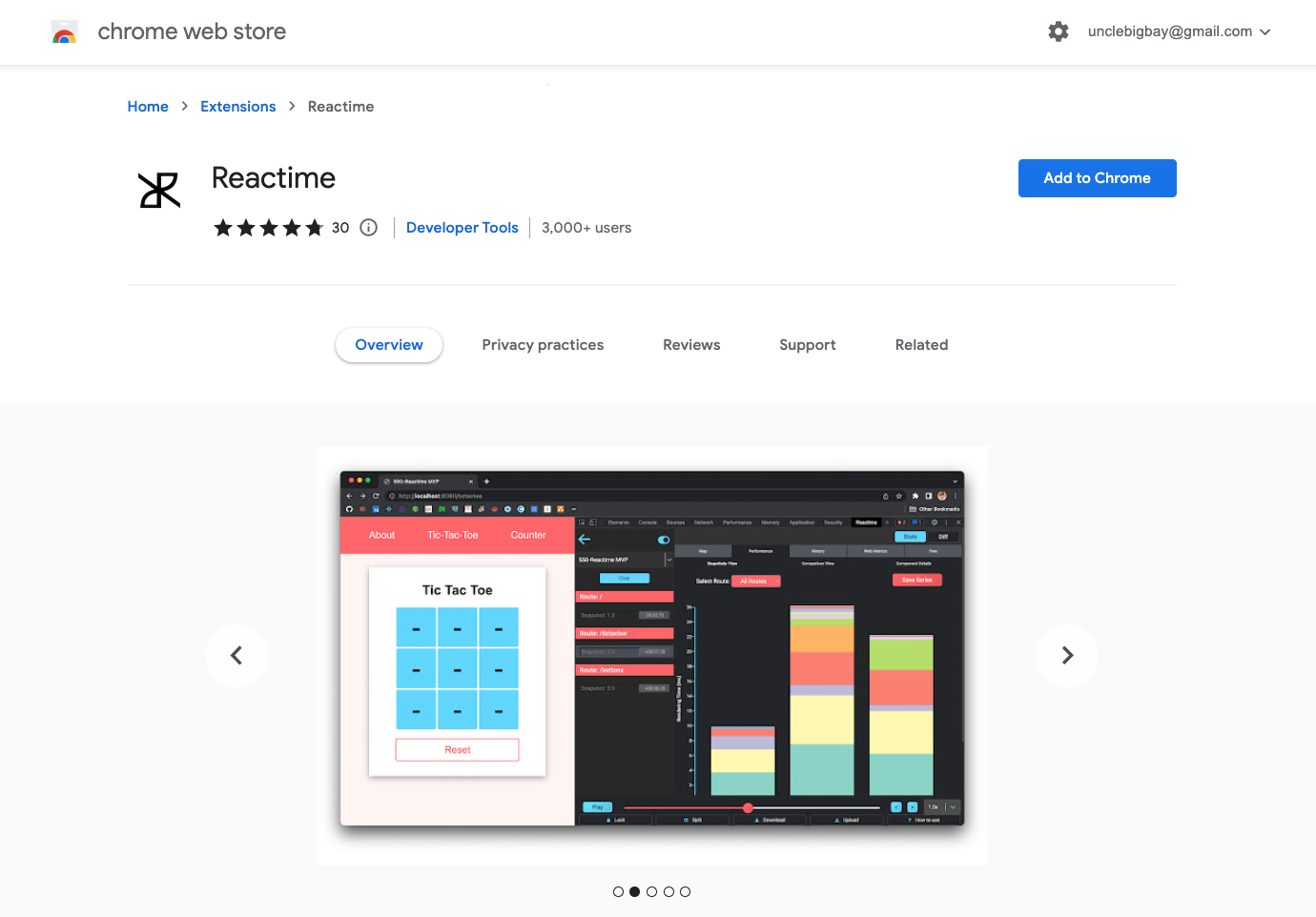
Reactime enables developers to record snapshots of a React app state. It allows you to jump between and inspect state snapshots, and monitor performance metrics such as component render time and render frequency.
Reactime extension version 14.0.0 features includes:
- Component Tree Visualization
- Record Snapshots of Application State
- Time-Travel Debugging
- Snapshot Series Comparison
- Component Render Time & Frequency
- Support for Gatsby & Next.js
- Beta Support for TypeScript
You can read more about working with the Reactime extension here.
9. React Quantum
React Quantum is another Chrome extension that creates a visual model for your React components. It parses through your React application to create a color-coded tree model of its component hierarchy when you hover over them.
See below for the official demo video of React Quantum:
You can install the React Quantum extension from the Chrome Webstore here.
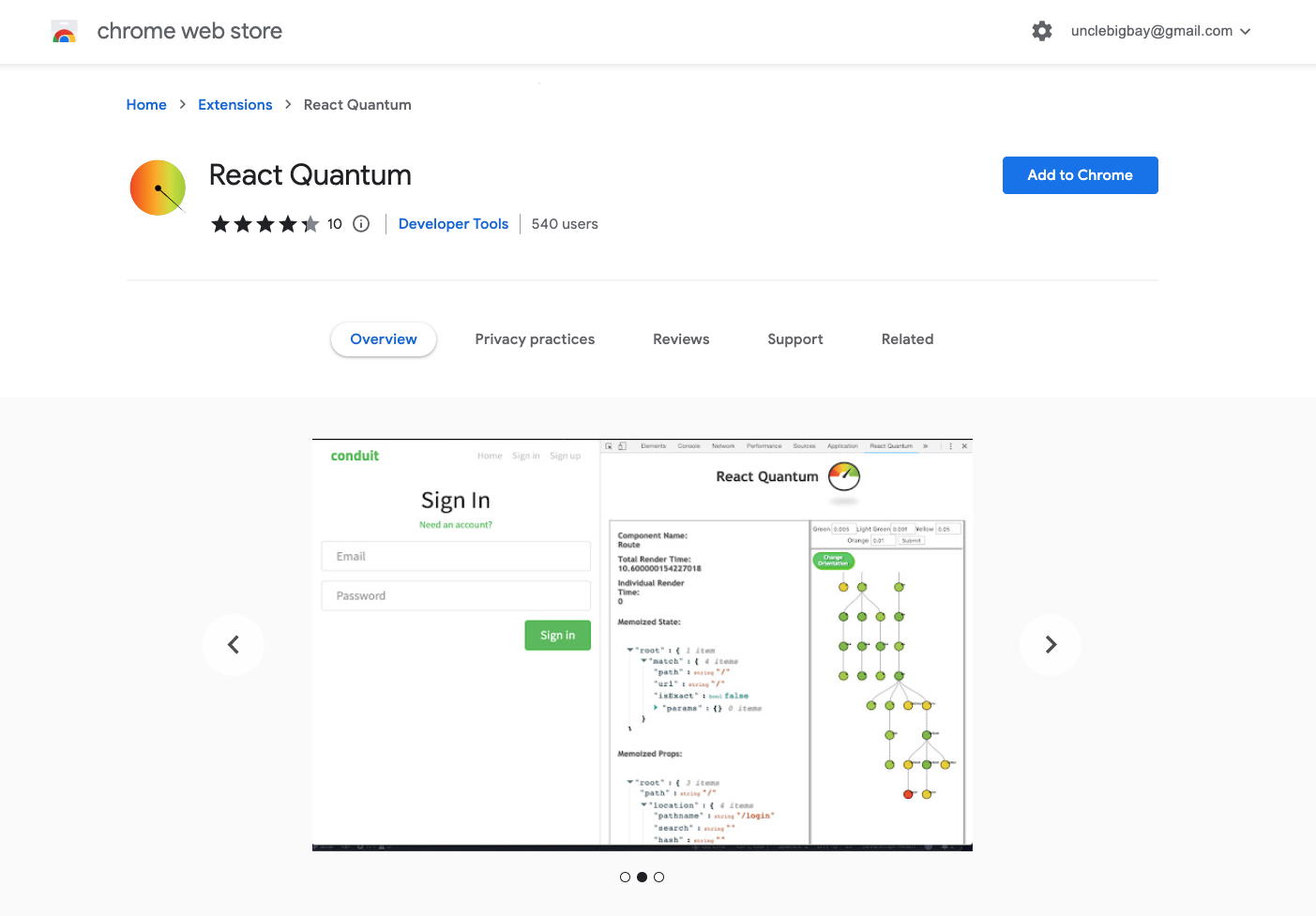
10. Realize for React
Realize for React is a React component tree visualizer. It allows React developers to track states and to have a holistic overview of the components created.
Realize for React developer tool is useful to visualize the state and structure of your React project when they’re growing in scale and complexity.
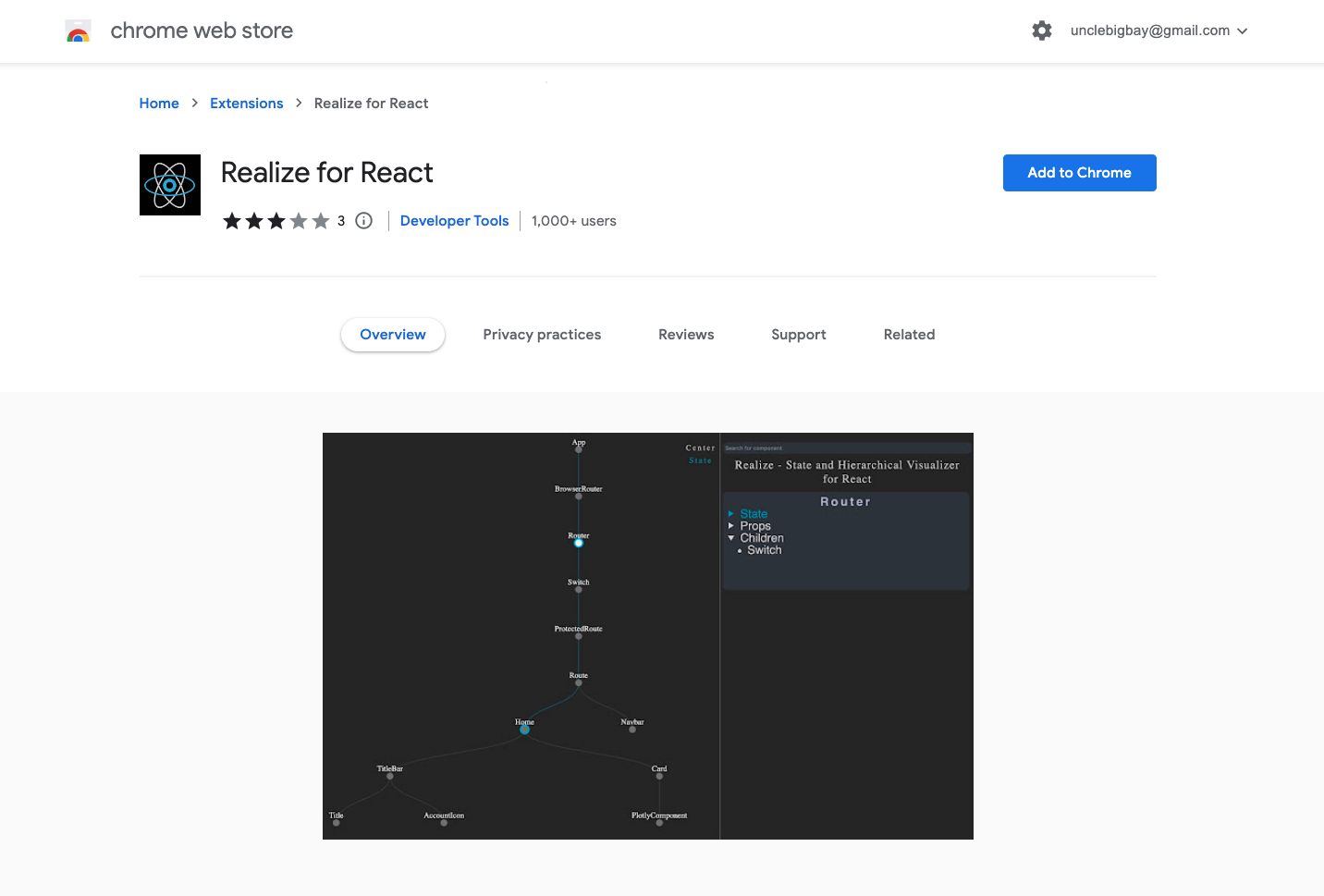
Realize is best used on non-deployed applications and you can install it from here.
11. React Stores Devtools Extension
The developers madeReact Stores Devtools extension for debugging React stores. It’s used along with the Chrome React devtools extension.
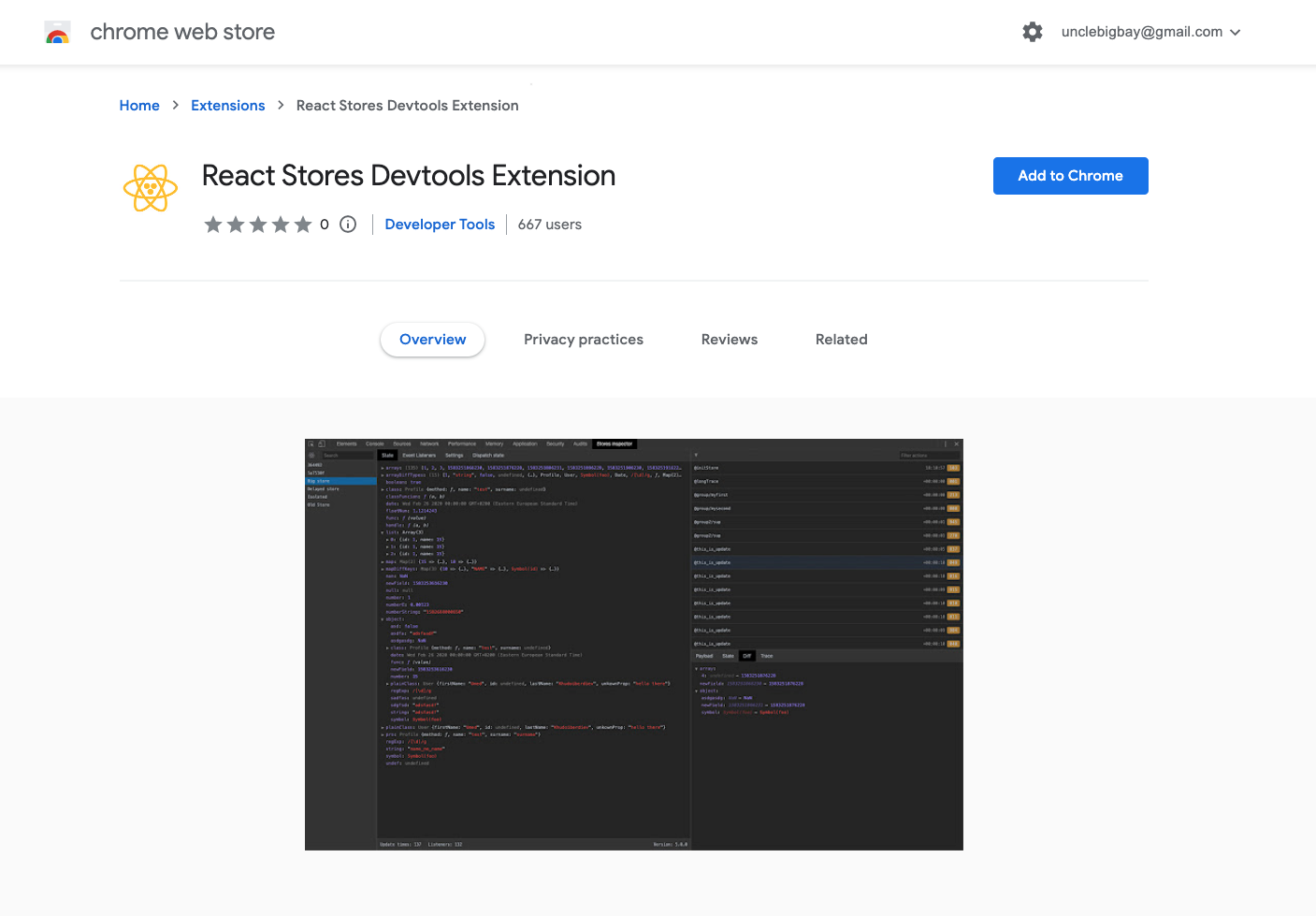
The React Stores Devtools extension features include:
- Inspect React store’s current state
- Check store history step-by-step
- Compare differences between states
- Dispatch state directly from devtools
- Clickable stack trace for history and listeners
- Use in the production build
12. Jinno: Design any website React/HTML/sketch
Jinno is a design system-focused extension that allows you to live edit any website as demonstrated below:

With the Jinno extension, you can move around any element on a website to have a visual of how they’ll look before designing them.
You can also modify the website properties (i.e text color) directly like you’re using Figma or Sketch. Use Jinno to save design snippets of any website built using React:

Jinno features include the following:
- Find any React open-source component
- Build design system
- Build storybooks
- Export Reactjs code
- Export Html code
- And export CSS code
You can learn how to convert Figma designs into Tailwind CSS here.
13. React Repositories New Tab
React Repositories New Tab is a chrome extension that displays the popular, new, and fresh public React Github repositories when you open a new Chrome tab.
See below for a demonstration:

Every new tab randomly shows the top ten list of most popular of all time, most popular newly created repositories, or most popular freshly updated (pushed) repositories.
Install the React Repositories New Tab from Chrome Webstore here.

Wrapping Up
Chrome extensions or developer tools make life easier for developers for detecting bugs earlier during development or on a production project. We explored up to 13 useful react dev tools in this article.
Where Do You Go Next?
Now that you know the useful Chrome extensions to improve your productivity as a React developer.