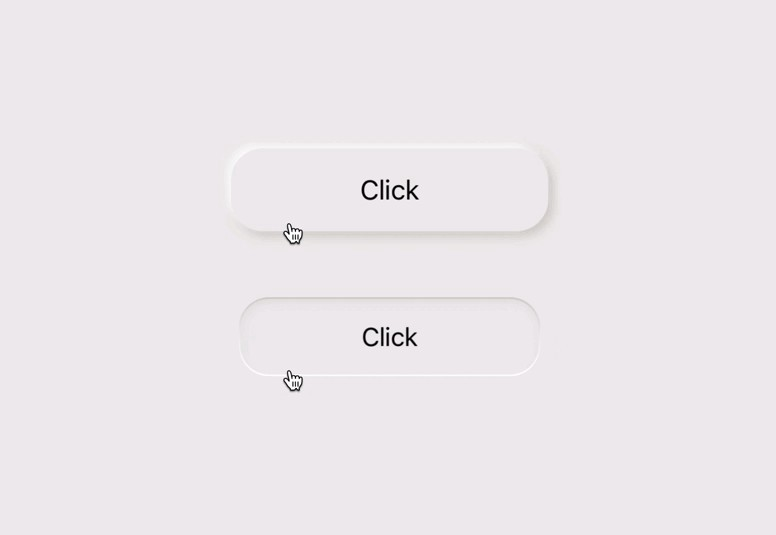
Introduction
If you think about how you got to this very page, we would agree that you made some actions. Actions like searching on the search bar, clicking on the link or subsequent links, and so on. Each of these actions around a website can be referred to as an event.
In JavaScript, an event is something that occurs on a web page. Such as a user clicking a button or moving their mouse over a specific area. When an event occurs, we instruct the web page to respond by displaying a message or changing the page.
For example, if you have a “Click me!” button on a web page, you can use JavaScript to make the page do something when a user clicks on the button. This could be used to display a message saying “You clicked the button!” or changing the color of the button.
Events are an important part of JavaScript’s functionality because they make web pages more engaging and interactive. This article will only cover the onClick event handler in a React application.
To get the most out of this article, it is a plus to have an understanding of the following;
In the upcoming sections, we go deeply into the React onClick event handler. But before we continue, If you’re looking for a great React solution to help you build React components more rapidly and be ready for production, take a look at the CopyCat plugin for React.
What are event handlers in React?
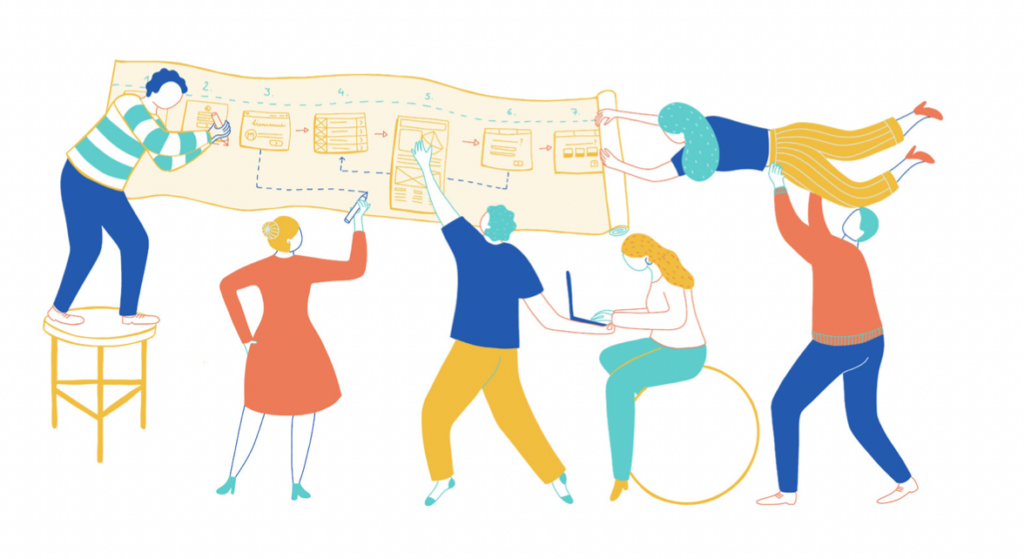
An event handler in React is a function that is used to handle a specific event. Such as a user hovering their mouse over an element or pressing a key on their keyboard. When an event occurs, the event handler function is invoked. It gives information about the event, such as the type of event and the element on which it occurred. The event handler uses this information to respond to the event in some way, like changing the state of the component or calling another function.
Event handlers are an essential component of React because they enable you to add interactivity to your components. For example, you can use an event handler to respond to a user hovering over an element by updating the state of your component or performing some other action.
To define an event handler in React, define a function that will be called when the event occurs. This function can be defined inline, through the use of an arrow function, or as a separate method on the component class. Then, using the on[Event] attribute, attach the event handler to the element that will handle the event. To handle a hover event on a div, for example, use the onMouseOver attribute, as shown below:
<div onMouseOver={this.handleHover}>Hover over me!</div>
When the user hovers over the div, the handle Hover function is called, and it receives event information as arguments. This information can be used by your event handler to respond to the event in some way.
In conclusion, React event handlers respond to particular events on elements, like a key press or hover events. They give you the option to make your components interactive and react to user actions in a variety of ways.
How does the onClick event handler work in React?
When a click event occurs on an element in a React application, the onClick event handler function is called. When a user clicks on an element, the onClick event is triggered. Then the event handler function is invoked, passing it event information. This data can include the type of event, the element on which the event occurred, and any other relevant information.
To use React’s onClick event handler, you must first define a function that will be called when the event occurs. This function is defined inline, through the use of an arrow function, or as a separate method on the component class. Then, using the onClick attribute, attach the event handler to the element that will handle the event. To handle a button click, for example, use the onClick attribute, as shown below:
<button onClick={this.handleClick}>Click me!</button>
When the user clicks the button, the handleClick function is called, and it receives event information as arguments. This information can be used by your event handler to respond to the event in some way.
To summarize, the React onClick event handler allows you to respond to user clicks on elements in your application. It is a function called during a click event, providing event information to respond to user action in some way.
Synthetic events in React
Synthetic events are a way for React to consistently represent browser events across all major browsers. They are referred to as “synthetic” events because React has re-implemented the browser’s event system rather than being native browser events.
Synthetic events in React are a way of representing browser events in a cross-browser consistent manner. They are referred to as “synthetic” because they are not native browser events, but rather a React re-implementation of the browser’s event system.
In React, you must use the on[Event] attribute to attach an event handler function to an element, where [Event] is the name of the event you wish to handle. For instance, you might use the onClick attribute to handle a button’s click event as follows:
<button onClick={this.handleClick}>Click me!</button>
When the user clicks the button, the handleClick function is called with a synthetic event object as an argument. This event object contains event information such as the type of event, the target element, and any other pertinent data. This information can be used by your event handler to respond to the event in some way.
However, synthetic events in React are a way of representing browser events consistently across different browsers. They allow you to handle events consistently without having to write separate code for each browser.
Handling the React onClick Event in React components
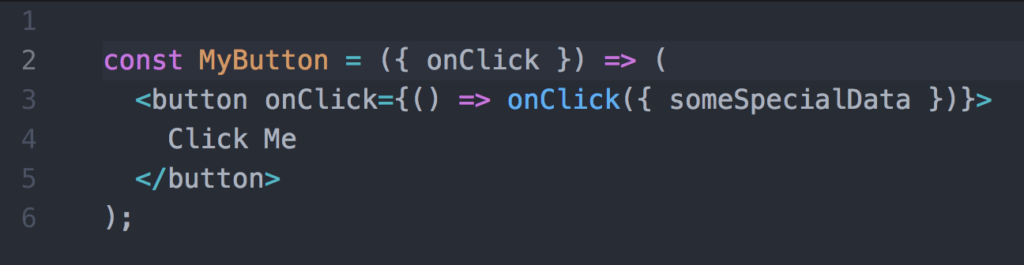
In this section, we will learn how to handle the React onClick event in React components. As we have discussed previously in this article, the React onClick event is used to handle the click event of the React component.
How to update a useState hook in a react component using the onClick event handler
The useState hook in React is a function that lets you add a state to functional components. The state is a type of data storage and management in a React component that is important because it allows the component to maintain and update its data over time.
However, you can update a useState hook in a react component using the onClick event handler. Let us take a close look at the lines of code below;
import React, { useState } from 'react';
import './App.css';
function App() {
const [count, setCount] = useState(0);
return (
<div className="App">
<h1>Count: {count}</h1>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
}
export default App;
The code above demonstrates how to use the onClick event handler to update a useState hook in a react component. The preceding code is a react component with a useState hook that updates the count state when the button is clicked.
However, the main purpose of this code is to demonstrate how to use the onClick event handler to update a useState hook in a react component. We have a button in the react component with an onClick event handler that calls the `setCount` function to update the count state.
The `setCount` function adds 1 to the current count state. When the button is pressed, the `setCount` function is called. The onClick event handler, on the other hand, is an inline function that calls the `setCount` function. This is why an arrow function is required.
To summarize, we can use the onClick event handler to update a useState hook in a react component.
How to call multiple functions in an onClick event handler
You can use JavaScript’s call or apply methods, or the async/await syntax, to call multiple functions in an onClick event handler in React.
Here’s an example of how to use the call method in an onClick event handler to call multiple functions:
function handleClick() {
function1.call(this);
function2.call(this, arg1, arg2);
function3.call(this);
}
<button onClick={handleClick}>Click me!</button>
In this case, the handleClick function is the event handler for the button’s onClick event. The call method is used within the handleClick function to call each of the functions that you want to run. As arguments, the call method accepts this value and any arguments that you want to pass to the function.
Here’s an example of how to use the apply method in an onClick event handler to call multiple functions:
function handleClick() {
function1.apply(this);
function2.apply(this, [arg1, arg2]);
function3.apply(this);
}
<button onClick={handleClick}>Click me!</button>
In this case, the handle Click function is the event handler for the button’s onClick event. The apply method is used within the handleClick function to call each of the functions that you want to run. As arguments, the apply method accepts this value and an array of arguments to pass to the function.
An example of using the async/await syntax to call multiple functions in an onClick event handler is shown below:
async function handleClick() {
await function1();
await function2(arg1, arg2);
await function3();
}
<button onClick={handleClick}>Click me!</button>
In this case, the handleClick function is the event handler for the button’s onClick event. The async/await syntax is used within the handleClick function to call each of the functions that you want to run. The await keyword is used to wait for each function to complete before proceeding to the next.
To summarize, you can use the call or apply methods, or the async/await syntax, to call multiple functions in an onClick event handler in React. This enables you to perform multiple tasks in response to a user clicking on an element in your application.
Handling an inline function using React onClick event handler
To handle an inline function in React using the onClick event handler, use an arrow function to define the function inline, as shown below:
<button onClick={() => this.handleClick()}>Click me!</button>
The onClick attribute is used to attach the onClick event handler to the button in this example. The event handler is defined by an arrow function that takes no arguments and calls the component’s handleClick method. When the user clicks the button, the handleClick method is invoked, and it receives event information as arguments.
You can also use the bind method to bind this value to the event handler function, as shown below:
<button onClick={this.handleClick.bind(this)}>Click me!</button>
The onClick attribute is used to attach the onClick event handler to the button in this example. The event handler is defined by invoking the bind method on the handleClick method, which assigns the method’s this value to the current component. When the user clicks the button, the handleClick method is invoked, and it receives event information as arguments.
To summarize, in React, you can use an arrow function or the bind method to define the event handler inline and attach it to the element using the onClick attribute to handle an inline function. This enables you to define the event handler function and attach it to the element in a single step, eliminating the need to define a separate event handler method on the component.
Passing parameters to an onClick event handler
In React, you can pass parameters to an onClick event handler by using an arrow function to define the event handler inline and including the parameters as part of the function’s argument list, as shown below:
<button onClick={(event) => this.handleClick(event, param1, param2)}>
Click me!
</button>
The onClick attribute is used to attach the onClick event handler to the button in this example. An arrow function is used to define the event handler, which takes the event object and two parameters, param1 and param2, as arguments. When the user clicks the button, the handleClick method is called with the event object and the two parameters as arguments.
You can also use the bind method to bind this value and the parameters to the event handler function, as shown below:
<button onClick={this.handleClick.bind(this, param1, param2)}>
Click me!
</button>
This example uses the onClick attribute to affix the button’s onClick event handler. By calling the bind method on the handleClick method, which sets this value and the method’s two parameters, param1 and param2, the event handler is created. The handleClick method will be invoked when the user clicks the button, and it will be given this value, along with the two parameters, as arguments.
In conclusion, you can use an arrow function or the bind method to define an onClick event handler inline and pass parameters to it by including them in the function’s argument list. In order to respond to the event in some way, you can use this to pass information to the event handler function.
Using Synthetic event in React with the onClick event handler
When using the onClick event handler in React, you must first attach the handler to the element using the onClick attribute, and then access the event data using the event object that is passed to the handler function.
Here is an illustration of how to use a fake event with React’s onClick event handler:
function handleClick(event) {
// Use the event object to access the event data
const target = event.target;
const type = event.type;
const x = event.clientX;
const y = event.clientY;
// Use the event data to respond to the event
console.log(`You clicked on ${target.tagName} at ${x},${y}`);
}
<button onClick={handleClick}>Click me!</button>
The handleClick function in this illustration serves as the event handler for the button’s onClick event. The event object is accessed within the handleClick function to gain access to event data such as the target element, event type, and mouse coordinates. The event is then dealt with using this information, perhaps by logging a message to the console.
In summary, to use a synthetic event with the onClick event handler in React, you need to attach the event handler to the element using the onClick attribute, and then access the event data using the event object.
Conclusion
Over the course of this article, we have been able to look at what event handlers are in React, how it works, synthetic events in React, handling the React onClick event in React component, using Synthetic events in React with the onClick event handler. We also talked about how to update a useState hook in a react component using the onClick event handler, how to call multiple functions in an onClick event handler, Passing parameters to an onClick event handler.
Check out the tutorial video below to explore more about the onClick event:
If you want to develop UI quicker than your competitors, check out our CopyCat tool. With the CopyCat Figma Bootstrap plugin, your Figma designs are consequently converted into fully supported, production-ready Bootstrap and React.js codes.
For more interesting reads, see the Copycat blog here.