- Introduction
- Why use React Strict Mode
- Setting up your React app
- Getting started with React Strict Mode
- Further questions
- More great articles from CopyCat Blog
- Conclusion
- Further readings
- Video on React Strict Mode and Examples
Introduction
React Strict Mode is a developer tool that has been around in React from version 16.3. But, if you are like me, you might be seeing it in your index.js
file without giving it a thought about why it has been there (if you bootstrapped your app using CRA).
// index.js
import React from 'react';
import ReactDOM from 'react-dom/client';
import './index.css';
import App from './App';
import reportWebVitals from './reportWebVitals';
const root = ReactDOM.createRoot(document.getElementById('root'));
root.render(
<React.StrictMode>
<App />
</React.StrictMode>
);
// If you want to start measuring performance in your app, pass a function
// to log results (for example: reportWebVitals(console.log))
// or send to an analytics endpoint. Learn more: https://bit.ly/CRA-vitals
reportWebVitals();
In this article, you will learn about React Strict Mode component, how to use it, and its advantages.
Why use React Strict Mode
React Strict Mode is a helper component that checks your component to see if you are using any unsafe lifecycles, deprecated life cycle methods that should not be used and it will cause alerts (warning messages) to be logged to the console while on strict mode. The React team uses this to let you know of methods or functions that they might remove or be deprecated in future versions of React. React Strict Mode is an essential developer tool used for notifying the developer of possible problems in their React web application. These problems range from not following the React guidelines and recommended practices, to using deprecated life cycle methods.
Also, there is no downside to having Strict Mode in production as it does not send the alerts while in production mode.
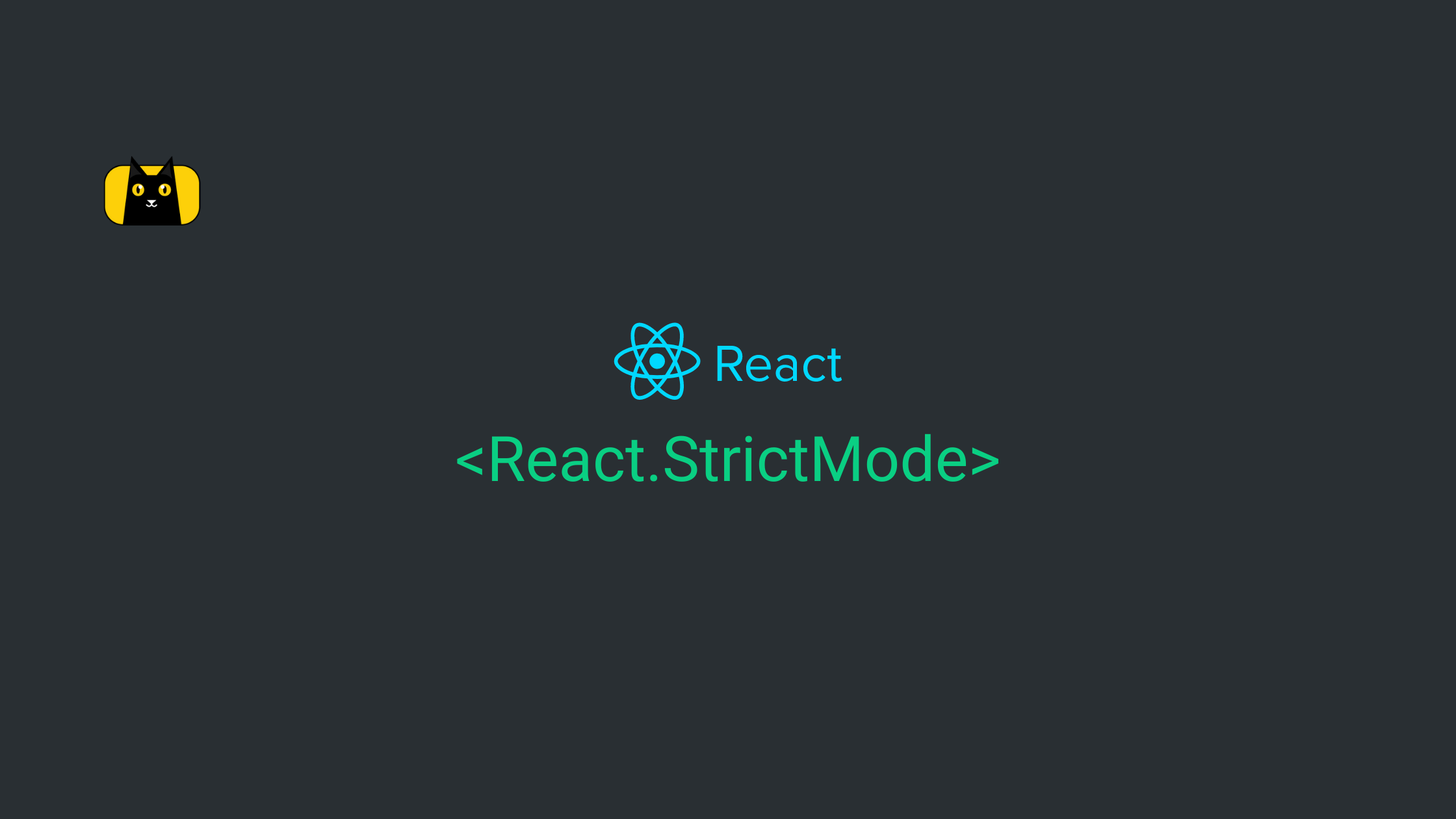
Advantages of using React Strict Mode
- Receive alerts (warning messages) when using deprecated React APIs
- Receive alerts (warning messages) when using unsafe lifecycles
- Alerts about the usage of the legacy string ref API
- Receive alerts when there are unexpected side effects
- It does not render any visible UI.
- It warns about the usage of the deprecated
findDOMNode
.
If you want to speed up your development of React Applications, we’ve built a tool just for that. Check it out here!
Setting up your React app
In this tutorial, you will be creating a React app using CRA.
Setup your development environment
You will scaffold a new React project. In this tutorial, I refer to my React app as react-strict-mode-tutorial
. In your terminal, type the following:
npx create-react-app react-strict-mode-tutorial
After having created your app and all dependencies automatically installed, you will need to navigate into the project directory and run the app. For that, run the following command in your terminal:
cd react-strict-mode-tutorial && npm start
You should see similar output below in your terminal:
$ npm start
> react-strict-mode-tutorial@0.1.0 start
> react-scripts start
You should get the following in your terminal upon completion of the commands above:
Compiled successfully!
You can now view react-strict-mode-tutorial in the browser.
Local: http://localhost:3000
On Your Network: http://192.168.137.1:3000
Note that the development build is not optimized.
To create a production build, use npm run build.
Your React app will then start hot reloading your development environment and your app should be running on localhost:3000
by default.
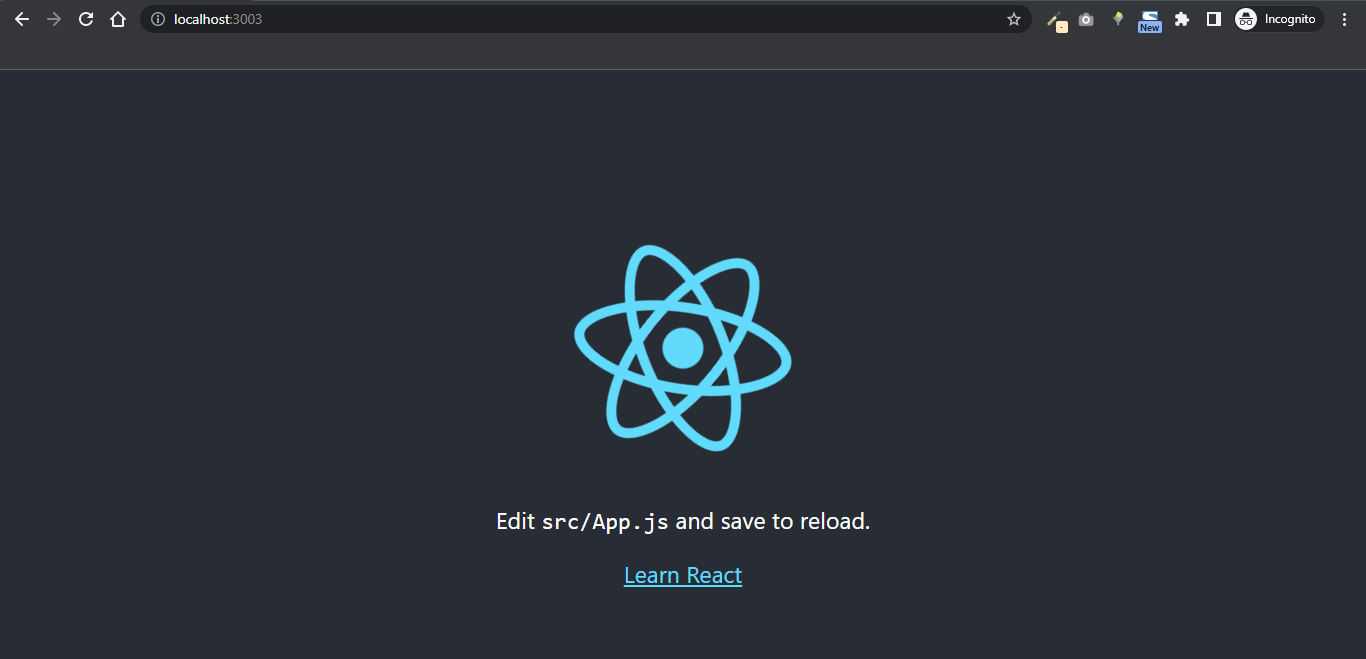
Getting started with React Strict Mode
To use React Strict Mode in your React application, you only need to wrap the children components with either <React.StrictMode>
or <StrictMode>
and then import StrictMode
from React at the top of your component so:
import React from 'react';
function ExampleApplication() {
return (
<div>
<Header />
<React.StrictMode>
<div>
<MyFirstComponent />
<MySecondComponent />
</div>
</React.StrictMode>
<Footer />
</div>
);
}
or
import React, { StrictMode } from 'react';
function ExampleApplication() {
return (
<div>
<Header />
<StrictMode>
<div>
<MyFirstComponent />
<MySecondComponent />
</div>
</StrictMode>
<Footer />
</div>
);
}
Explanation of what is going on with the above snippet
- StrictMode checks will only be applicable on
MyFirstComponent
andMySecondComponent
. This is because they are the children components ofStrictMode
. - However,
Header
andFooter
will not have any checks by Strict Mode. - React Strict Mode will not render any visible UI.
Below is an example of a warning message React Strict will log in to your console:

Further questions
Is Strict Mode enabled in React by default?
Yes. React Strict mode is enabled by default on the entire application if you bootstrapped your app using CRA. However, you do not need to wrap your entire application in Strict Mode, you can choose to isolate only a few components in Strict Mode like so:
Start by importing StrictMode
from react;
// App.js
import { StrictMode } from "react";
Then wrap the components you want Strict Mode
to have access to and monitor
// App.js
const App = () => {
return (
<QueryClientProvider client={queryClient}>
<StrictMode>
<Repositories />
</StrictMode>
</QueryClientProvider>
);
};
Finally, your App.js
will look like so;
// App.js
import { StrictMode } from "react";
import { QueryClient, QueryClientProvider } from "react-query";
import Repositories from "./components/Repositories";
const queryClient = new QueryClient({});
const App = () => {
return (
<QueryClientProvider client={queryClient}>
<StrictMode>
<Repositories />
</StrictMode>
</QueryClientProvider>
);
};
export default App;
Do we need React strict mode?
Yes. The main reason for using it is to detect bugs while in development mode. Strict Mode is a useful React feature, as such you need it. React StrictMode
renders every of your application’s components twice, this enables React Strict mode to identify defects within your application and log the error messages.
More great articles from CopyCat Blog
- [Your Guide To The React Context API](https://www.copycat.dev/
- React Query Tutorial: Getting Started with Fetching Data and State Management with Examples
- React Calendar: How to Build a Reusable Calendar Component
- Guide to building your ReactJS custom hooks
- React Query Tutorial: Getting Started with Fetching Data and State Management with Examples
Conclusion
In this tutorial, you learned about React Strict Mode component and how it benefits you as a developer by allowing you to write efficient and standardized codes. The component does this by sending alerts to the console when React detects that you are using methods that should not be used.
It is recommended to use React Strict Mode if you want to be prepared for future upgrades and do not want to use legacy features.
Further readings
- Strict Mode in ReactJS by Nadun Malinda
- React Strict Mode – by Paola Dolcemascolo
- Using strict mode in React 18: A guide to its new behaviors – LogRocket Blog
Video on React Strict Mode and Examples
If you’re looking out for cool React tools that’ll help you to write component code faster and be production ready faster than your competition, don’t forget to check out the CopyCat plugin for React!
Happy Coding!
For more insightful React articles, check out our blog. We’ve built a tool to cut down development time by about 35%; check it out here!