Introduction
Modals are common User Interface(UI) elements in applications. Material UI, a React JS component library makes it easy and straightforward to implement Modals.
During the design stage of developing applications, it is possible to transform those designs into code using the Copycat Figma plugin. It is a great tool and works well.
What is a Modal?
A Modal (also called Modal window) is any component that positions itself on top of the main window of an application. Modals disable the main window until users close them.
One Importance of a modal is displaying important information to the user. A Modal can also be a call-to-action for the user. Whenever interaction needs to be paused to pass a message across to the user, a Modal is used.
What is Material UI Modal?
Material UI Modal is a pre-built component in Material UI. It allows for flexibility to build and customize modal windows of any type and purpose. It also serves as the base for the other specialized Modal components in Material UI.
Building a Simple Modal in React
To use Material UI Modal, we need to make sure some things are in place. First of all, the article assumes that the reader has basic knowledge of React JS. The article also assumes that the reader already has a React JS Application running. With those in place, we move to the next step.
Install & Setup Material UI
To install Material UI, we run the following command in our terminal:
npm install @mui/material @emotion/react @emotion/styled
This command installs Material UI and Emotion (the Styling engine) into our app. After this step, we move on to properly using the Modal component.
Creating a simple Modal
We should note that what actually gets displayed on the screen is going to be whatever the Modal Component contains as a child.
Creating a new component in our React App called “SimpleModal”, we put in the following lines of code.
import { useState } from 'react'
import { Modal, Box, Typography, Button } from '@mui/material'
function SimpleModal() {
const [ open, setOpen ] = useState(false)
const handleOpen = () => setOpen(true)
const handleClose = () => setOpen(false)
const style = {
width: '25rem',
bgcolor: 'background.default',
p: 2,
position: 'absolute',
top: '50%',
left: '50%',
transform: 'translate(-50%, -50%)'
}
return (
<div>
<Button variant='contained' onClick={handleOpen}>Open Modal</Button>
<Modal
open={open}
onClose={handleClose}>
<Box sx={style}>
<Typography variant='h6' component='h2'>Modal Header</Typography>
<Typography variant='body1' paragraph>Lorem ipsum dolor sit amet consectetur adipisicing elit. Impedit necessitatibus corrupti, quo at earum eveniet veritatis enim alias esse placeat.</Typography>
</Box>
</Modal>
</div>
)
}
export default SimpleModal
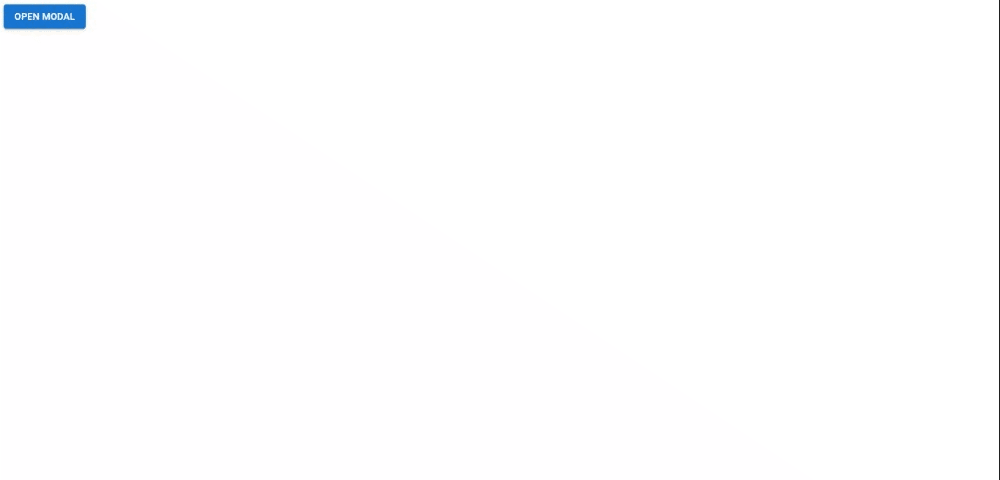
Here, <Modal>
serves as a container and Box as a child of the container. To build a Modal, it needs to have a single child that contains all the other elements on display inside the window.
Types of Modals in Material UI
Even though a component called Modal exists in this component library, it is low-level and still needs extra customization to satisfy some specific needs.
There are other components in the library that are Modals too but work better for specific needs. They are even built using the Modal component as a base.
Below are the 4 types of modals in Material UI with a video resource to learn more about them:
- Dialog
- Menu
- Drawer
- Popover
Conclusion
With this article, you should have a firm grasp on how Modals work in Material UI and how to create one yourself. Also, don’t forget to check out Copycat Figma Plugin. It converts Figma designs to code.