- Introduction
- Q1. Why are Fragments better than Divs?
- Q2. Why should we not Update the State directly?
- Q3. How does the Virtual Dom work?
- Q4. When would you call React's useMemo() method?
- Q5. What is the difference between class and functional components?
- Q6. Describe the lifecycle methods in React.js.
- Q7. Describe useState in detail.
- Q8. Explain Reconciliation in React
- Q9. What is the difference between Controlled and Uncontrolled Components?
- Q10. Explain what a higher-order component is
- Conclusion
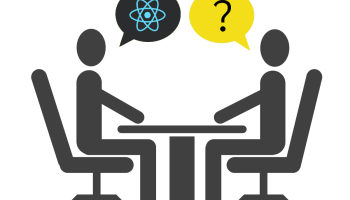
Introduction
React has become a go-to solution for developers wanting to construct user interfaces. It is one of the most popular front-end JavaScript frameworks with over 17 million weekly downloads on Npm and 199k stars on GitHub.

React is the most used frontend library according to the state of frontend 2022 survey.
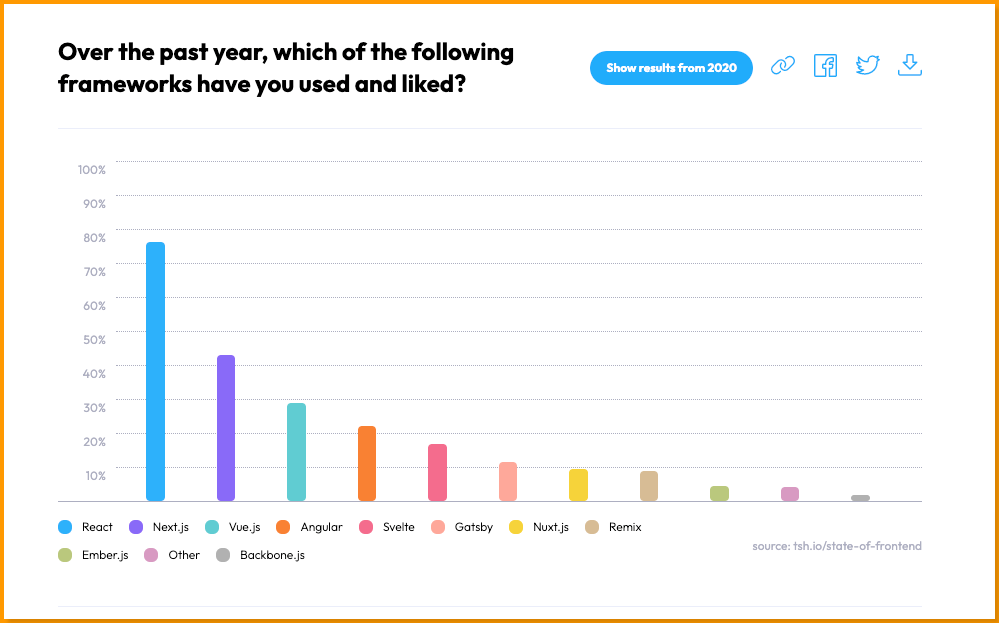
React’s emphasis on declarative programming and reusable components makes it easier to create sophisticated and performant user interfaces.
You’ve come to the perfect spot if you want to brush up on your React abilities in preparation for a job interview. In this piece, we’ll go through the top 10 React interview questions and answers for 2022.
These questions are intended to assess your understanding of fundamental React principles and patterns, as well as your ability to think critically and solve issues.
Declarative Programming Model
The declarative programming model is a crucial aspect of React. This implies that rather than defining the actions the browser should take to update the UI, you just define what the UI should look like at a given moment in time. React will then update the UI to fit your description. This makes reasoning about your program easy and can help you avoid common issues.
In addition to its declarative programming paradigm, React contains the “Flux” architecture. It is a strong tool for managing state and data flow. This design employs a unidirectional data flow. This makes it easy to forecast how data will flow through your react application as well as discover and fix problems.
If you’re looking for a tool to convert Figma to React, CopyCat is your best bet. Use CopyCat to quickly convert your Figma design to React; learn more about this tool here.
Now that we know what React is and why it’s essential, let’s look at the top 10 React interview questions for 2022.
Q1. Why are Fragments better than Divs?
When this question is asked, the interviewer is mostly looking to see if you understand and care about controlling the performance of your react application. Because divs add extra nodes to the DOM, which can influence the performance of your application negatively. When the page is rendered, the browser must establish and maintain these extra nodes, which might require time and resources. As a result, your application may become slower and less efficient.
As a result, the answer to this question will be as follows:
In React, fragments are more advantageous than div elements because they allow you to organize a list of child component without adding extra nodes to the DOM. This increases the efficiency of your components and makes your rendering methods easier to comprehend. Furthermore, utilizing fragments allows you to return multiple items from a component’s render function without having to wrap them in an additional element like div, section or span, etc. This can aid in the organization of your components and the avoidance of unneeded DOM nodes.
Q2. Why should we not Update the State directly?
This question is designed to assess your grasp of data flow in React. The basic answer to this question is that directly updating the state will not re-render the react components. But if you want to appear professional, thus the correct response to this question is any of the following:
- It violates the fundamentals of unidirectional data flow, a key idea in React. Data that flows in a single direction, from the parent component to the child component, is said to have a unidirectional data flow. By skipping this flow and updating the state directly, you run the risk of having your react and web applications behave unexpectedly.
- Directly altering the state can result in unexpected behavior. Such as the inception of infinite loops or the needless re-rendering of components. This can make it challenging to comprehend and debug your code.
- Directly changing the state can make it more challenging to understand and maintain your code. Since their state might be connected to a particular implementation, it can also make it more challenging to reuse components.
- You can make your code more predictable and simple to work with by adhering to the unidirectional data flow and avoiding direct state updates. This can make it simpler for you to write clear, well-structured code that is also easier to maintain.
Q3. How does the Virtual Dom work?
React employs the virtual DOM to optimize updates to the actual DOM. When the state of a component changes, the virtual DOM computes the difference between the previous and new versions of the component. This calculation is performed in JavaScript, which is significantly faster than updating the DOM. The virtual DOM then updates the actual DOM with only the necessary changes, making updates faster and more efficient.
Furthermore, the virtual DOM works by storing in memory a lightweight representation of the actual DOM. This representation is a tree-like structure, with each node representing a DOM element. When the state of a component changes, the virtual DOM updates the corresponding node in the virtual representation and computes the difference between the component’s previous and new versions. Then it makes the bare minimum of changes to the actual DOM.
Q4. When would you call React’s useMemo() method?
The interviewer is now checking how thoroughly you have used react applications or if you have worked on complex projects. Consequently, the following would be the response to this question:
When you want to enhance a component’s performance in React by caching an expensive computation, you would call the useMemo()
method. This can be helpful if you have a time-consuming, complicated calculation that you would rather not repeat if the dependencies remained the same. The useMemo()
allows you to memoize the calculations’ outcome so that it only needs to be recalculated if one of the dependent variables has changed. This can help increase the component’s performance and boost the effectiveness of your react application.
However, to use useMemo
, all you have to do is pass it a list of dependencies as well as the function you want to memoize as the first parameter. The function will be called and the output saved in a cache when React displays your component. If any of the dependencies sent to useMemo
changes, the function will be re-run and the cache will be updated with the revised result. The cached outcome will be applied in all other cases.
It’s vital to remember that useMemo
is only useful if you wish to avoid executing a complicated computation or expensive action with each render.
The useMemo
‘s performance advantages will typically be minor, hence it is normally preferable to design plain, simple code that is simple to read and maintain. Use useMemo just if you have a particular performance issue that you’re seeking to fix.
Q5. What is the difference between class and functional components?
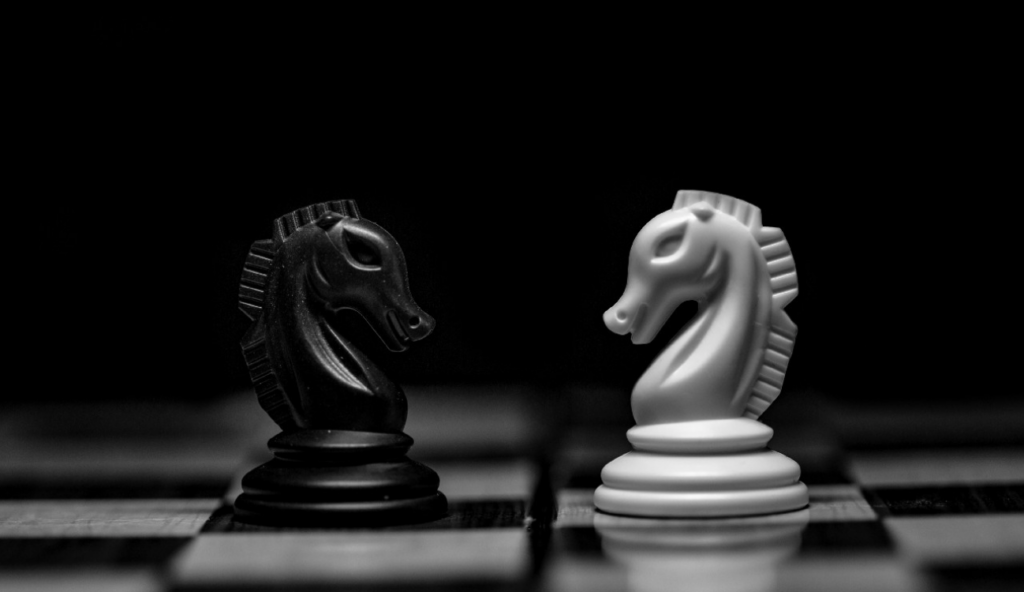
There are two different ways to define a component in React: class components and functional components. JavaScript classes that extend the base React are known as class components.
The class component has a render()
method that returns the component’s JSX representation. Contrarily, functional components are JavaScript functions that take an argument called props and return a JSX representation of the component.
Class components have access to extra features like local state and lifecycle methods. It also has the ability to manage a component’s context, which is one of the main differences between class and functional components. Functional components are more straightforward and can only access the props that have been passed to them.
Class components are typically appropriate for defining complex components that have local state, and lifecycle methods, or require context management. Simple components that are only required to accept props and render a view are better suited for a functional component. The specific requirements of the component you are defining ultimately determine whether to use class or functional components.
You can check out a video resource for better understanding of when to use class or functional components in React.
Q6. Describe the lifecycle methods in React.js.
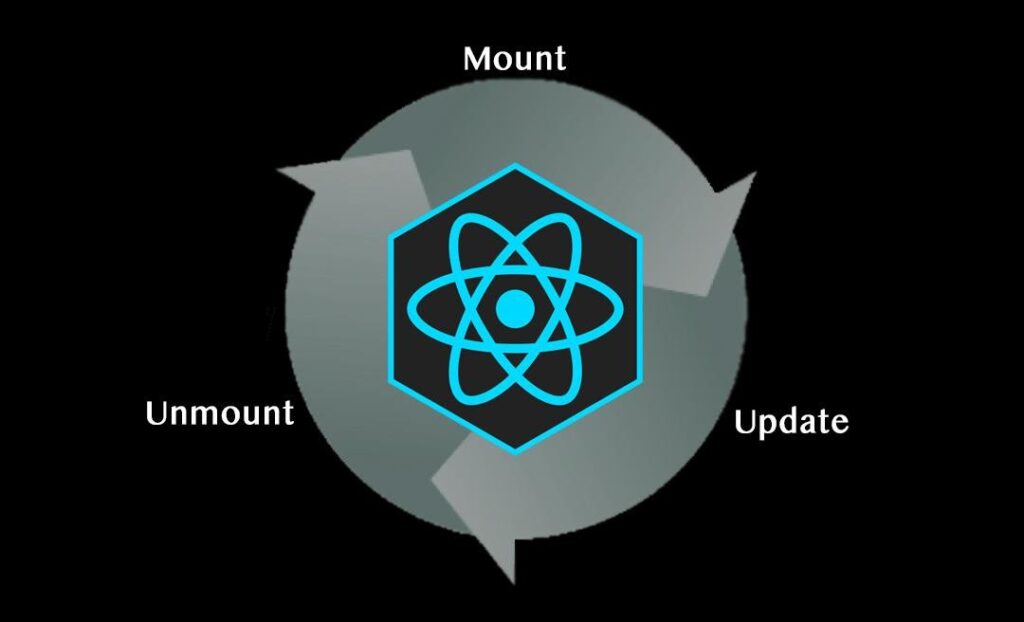
The lifecycle of a component in React refers to the various phases that a component experiences from creation to destruction. When a component is created, updated, or destroyed, the React lifecycle method lets you perform specific actions and control what happens at each stage.
React’s primary lifecycle methods are:
componentDidMount()
: After a component has been rendered to the DOM, this method is called. This method sets up the component in any way that is required, including gathering data or configuring event listeners.shouldComponentUpdate()
: Before a component is updated, this method is called. When a component needs to be re-rendered, you can decide using this method. You can stop the component from being updated by returning false from this method.componentDidUpdate()
: A component is updated before this method is called. After the component has been updated, you can use this method to carry out any necessary cleanup or other tasks.componentWillUnmount()
: Before a component is destroyed, this method is invoked. Any cleanup work that needs to be done for the component can be done using this method. Including removing event listeners and canceling any pending network requests.
Although there are several methods used to manage a component’s lifecycle, these are the main lifecycle methods in React. The actions that are taken when a component is created, updated, or destroyed can be controlled by using these methods. You can also control what happens at each stage of a component’s lifecycle. The behavior of your components can be managed in this way, ensuring they behave as you intended them to.
Q7. Describe useState in detail.
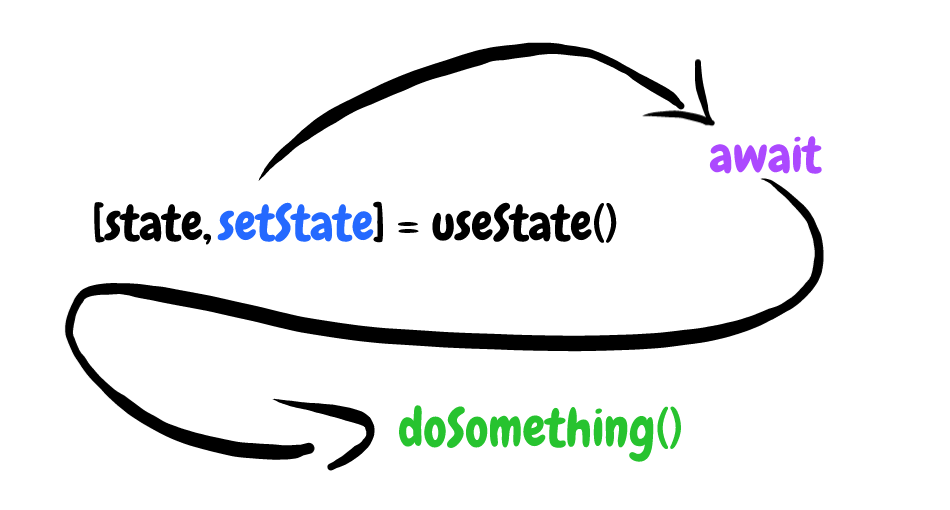
A state hook is a method for a React functional component to keep track of its state. State, in general, refers to the data or variables that a component stores and utilizes to generate its user interface.
Only class-based components could hold state prior to the introduction of hooks. This meant that if a functional component needed a state, it had to be converted to a class-based component. This, however, was not always desired or even practical because it involved modifying the structure of your component and might present further issues.
Functional components may now contain state, much as class-based components, thanks to the useState
hook. As a result, you can keep your component functions short and clear while still managing the state within them.
You must import useState
from the React library at the start of your component file to use it. Then, within your component method, call useState
and pass in your state’s initial value. The useState
hook returns an array containing two elements; the current state value and a function that may be used to change the state.
You may then destructure the array and utilize these two values in your component. The current state value may be used like any other variable, and the update function can be invoked anytime the state has to be changed. This will cause the component to re-render, allowing it to reflect the modified state.
To summarize, useState
enables functional components to have states of their own, allowing them to maintain and update the data that they use to generate their user interface.
This simplifies the creation of basic, functional components that retain the features and capabilities of class-based components.
Q8. Explain Reconciliation in React
Reconciliation is the mechanism through which React updates the DOM when the state of a component changes. When the state of a component changes, React updates the virtual DOM, a lightweight replica of the actual DOM in memory. The virtual DOM computes the difference between the prior and new versions of the component and then updates the actual DOM with the bare minimum of modifications. This speeds up and improves the efficiency of the updates.
This essentially implies that when a component’s prop or state changes, react determines if a real DOM update is required by comparing the newly returned element with the previously displayed one; if they are not equal, react will update the dom, this is a process known as Reconciliation.
Q9. What is the difference between Controlled and Uncontrolled Components?
In React, there are two approaches to constructing forms; controlled and uncontrolled components. A controlled component is one whose value is controlled by the React component, whereas an uncontrolled component is one whose value is controlled by the DOM itself.
React components determine the value of the input in a controlled component, and the component is responsible for handling changes to that value.
When a user types something into a controlled input, the React component that controls the input updates its state, and the input is updated to reflect the new value in the state. This gives the React component complete control over the form input, ensuring that it is always in sync with the rest of the application state.
Meanwhile, The DOM determines the value of the input in an uncontrolled component, and the React component has no influence over it. When a user puts anything into an uncontrolled input, the value of the input is modified, but the React component is unaware of the change. This might cause conflicts between the value of the input and the rest of the application state, making some functionality, such as validation or formatting, more difficult to provide.
In summary, controlled components are usually seen to be a superior approach to constructing forms in React since they allow the React component greater control over the input and make features like validation and formatting easier to implement. Uncontrolled components can be valuable in some circumstances, but they must be utilized with caution.
Q10. Explain what a higher-order component is
A higher-order component (HOC) is a function that accepts a component as an argument and returns another component. In React, HOCs are a valuable tool for reusing code and logic across components. They are frequently used to extend the functionality of existing components, for adding new properties or behaviors.
Creating a higher-order function requires defining a function that accepts a component as an argument and returns a new component. The new component might be based on the input component or wholly distinct. Within the HOC function, you may add extra logic or behavior to the input component and then return the updated component.
Also, HOCs are frequently used to abstract away complicated logic or behavior that is shared by several components. To avoid having each component implement its own authentication and authorization logic, you might, for instance, design a HOC that handles authentication and authorization for a group of components.
Conclusion
For anybody hoping to succeed in their React interview, these top 10 React 2022 interview questions are crucial. The subjects covered in these questions vary from fundamental React ideas to more complex ones like Higher Order Components, data flow, and so on. You may increase your chances of succeeding in your React interview and securing the position of your dreams by becoming familiar with these questions and their responses.
These top 10 React 2022 interview questions and answers will help you prepare for your upcoming interview and give you the confidence you need to succeed, whether you are a novice or an expert React developer.
Do you know that by converting your Figma files to a live React project, CopyCat is a solution that can help you cut your development time by roughly 35%? View it right here.
Interesting Reads from our Blogs: